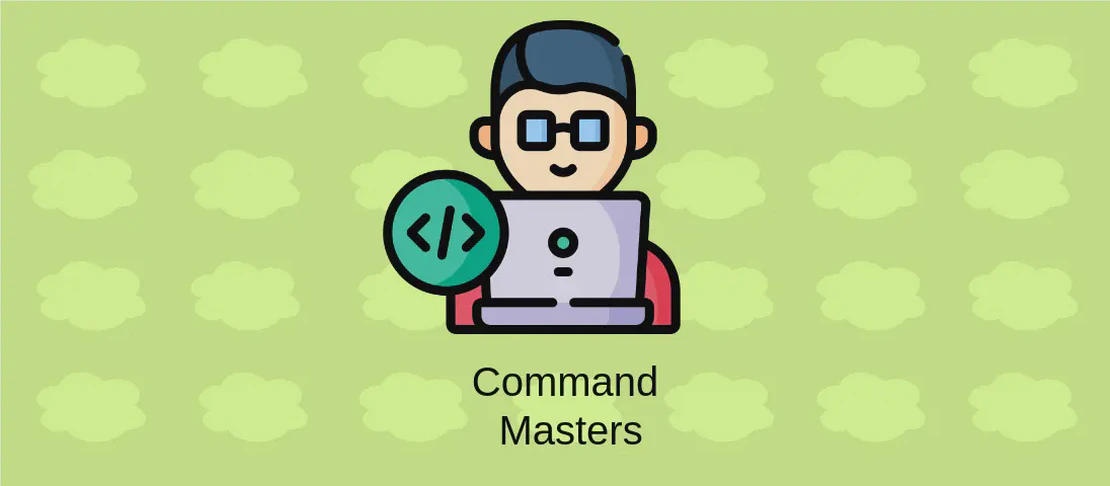
How to use the command '2to3' (with examples)
The 2to3
tool is an automated utility for converting Python 2 code into Python 3 code. As Python 3 has become the standard, with Python 2 being officially unsupported as of January 1, 2020, developers often need to update legacy Python 2 codebases. The 2to3
tool analyzes Python 2 code and converts it to comply with Python 3 syntax and practices. However, it’s worth noting that this tool has been deprecated since Python 3.11 and is completely removed in Python 3.13. Despite its removal, understanding its use can provide insights into code conversion processes and historical code base management.
Use case 1: Display changes without performing them (dry-run)
Code:
2to3 path/to/file.py
Motivation:
This use case involves running the 2to3
tool in a preview mode to assess which modifications the tool would apply to a given Python 2 script. This is particularly useful when you want to understand the scope of changes without immediately altering code. It helps developers to make informed choices about whether further manual adjustments are necessary alongside the automated changes.
Explanation:
- 2to3: Invokes the 2to3 code conversion tool.
- path/to/file.py: Specifies the path to the Python 2 file you want to analyze.
Example Output:
The tool lists potential changes. For instance, the output might show lines in Python 2 syntax followed by suggested changes, such as updating print statements.
Use case 2: Convert a Python 2 file to Python 3
Code:
2to3 --write path/to/file.py
Motivation:
This use case is essential for developers ready to convert their Python 2 code to a Python 3 compatible format. Running this allows you to directly update the file in place, enabling immediate compatibility with Python 3 features and syntax.
Explanation:
- 2to3: Initiates the 2to3 tool for code conversion.
- –write: Indicates that the tool should apply the changes directly to the specified file.
- path/to/file.py: Designates the specific Python 2 file to convert.
Example Output:
After executing, the original file is rewritten with Python 3 syntax. For example, print statements without parentheses are updated to their Python 3 format.
Use case 3: Convert specific Python 2 language features
Code:
2to3 --write path/to/file.py --fix raw_input --fix print
Motivation:
This use case is particularly useful when you want to target specific language features for conversion, leaving the rest of the code untouched. This can be beneficial when certain syntax changes, like adjusting raw_input
function to input
or updating print statements, are a priority.
Explanation:
- 2to3: Runs the conversion tool.
- –write: Specifies the conversion should be applied to the file.
- –fix raw_input: Ensures the tool replaces
raw_input()
withinput()
, a common change from Python 2 to 3. - –fix print: Converts print statements from Python 2 format to include parentheses, complying with Python 3 syntax.
- path/to/file.py: The target file for these specific changes.
Example Output:
The resulting file will only have changes related to raw_input
and print
functions being updated.
Use case 4: Convert all Python 2 features except specified ones
Code:
2to3 --write path/to/file.py --nofix has_key --nofix isinstance
Motivation:
This is beneficial when you want to convert all code to Python 3 but wish to exclude certain features that you plan to handle manually or customize differently. This selective conversion helps manage specific cases where automatic conversion may not be ideal.
Explanation:
- 2to3: The conversion tool.
- –write: Directly applies changes to the specified file.
- –nofix has_key: Excludes
has_key
from the conversion process, preventing automatic removal in dict usage. - –nofix isinstance: Prevents changes to
isinstance
calls, allowing for custom handling. - path/to/file.py: The file being converted partially.
Example Output:
Changes are applied except for has_key
and isinstance
statements, which remain in their original Python 2 format.
Use case 5: List available language features for conversion
Code:
2to3 --list-fixes
Motivation:
Listing all possible fixes provides insight into what conversions 2to3
can automate. It serves as a reference guide, helping developers understand the extent and capability of 2to3
in transforming a Python 2 codebase to Python 3.
Explanation:
- 2to3: The command to access conversion functionalities.
- –list-fixes: Requests a list of all possible language features and syntax elements that
2to3
can convert.
Example Output:
The output lists all the conversion options available, such as changing print
statements, adapting to new division rules, or removing old string formatting methods.
Use case 6: Convert directory of Python 2 files to Python 3
Code:
2to3 --output-dir path/to/python3_directory --write-unchanged-files --nobackups path/to/python2_directory
Motivation:
Applying 2to3
to an entire directory allows batch processing of multiple files, making it time-efficient when upgrading large projects with numerous Python 2 source files. You can systematically update the entire codebase, crucial for future-proofing software.
Explanation:
- 2to3: Executes the conversion tool.
- –output-dir path/to/python3_directory: Specifies where to place converted files instead of overwriting the originals, maintaining a Python 3 version.
- –write-unchanged-files: Includes files not altered in the conversion process, ensuring a complete directory in the new output.
- –nobackups: Avoids creating backup files for each original file, conserving disk space.
- path/to/python2_directory: Specifies the source directory containing all Python 2 files for conversion.
Example Output:
A new directory filled with files either converted to Python 3 or kept unchanged, ready for immediate Python 3 runtime compatibility.
Use case 7: Run 2to3 with multiple threads
Code:
2to3 --processes 4 --output-dir path/to/python3_directory --write --nobackups --no-diff path/to/python2_directory
Motivation:
Using multiple threads accelerates the conversion process by dividing workloads across processor cores. This is particularly advantageous for large projects where single-threaded operations could take considerable time.
Explanation:
- 2to3: Initiates the conversion tool.
- –processes 4: Splits processing across four concurrent threads, enhancing conversion speed.
- –output-dir path/to/python3_directory: Directs converted code to a separate directory.
- –write: Commits changes to the files directly.
- –nobackups: Prevents creating backup copies of original files.
- –no-diff: Suppresses the diff output for conversions, streamlining logs.
- path/to/python2_directory: Specifies the directory containing the Python 2 files.
Example Output:
A multi-threaded conversion results in a completed Python 3 directory in substantially less time than a single-threaded approach.
Conclusion:
The 2to3
tool was an instrumental utility in transitioning Python 2 codebases to Python 3 by automating syntax updates. Despite its deprecation and removal in later Python versions, understanding how it operates provides valuable lessons in managing and modernizing code. The examples above showcase various functionalities of 2to3
, from specific feature fixes to batch file processing, reflecting both its power and limitations in facilitating the transition to newer language versions.