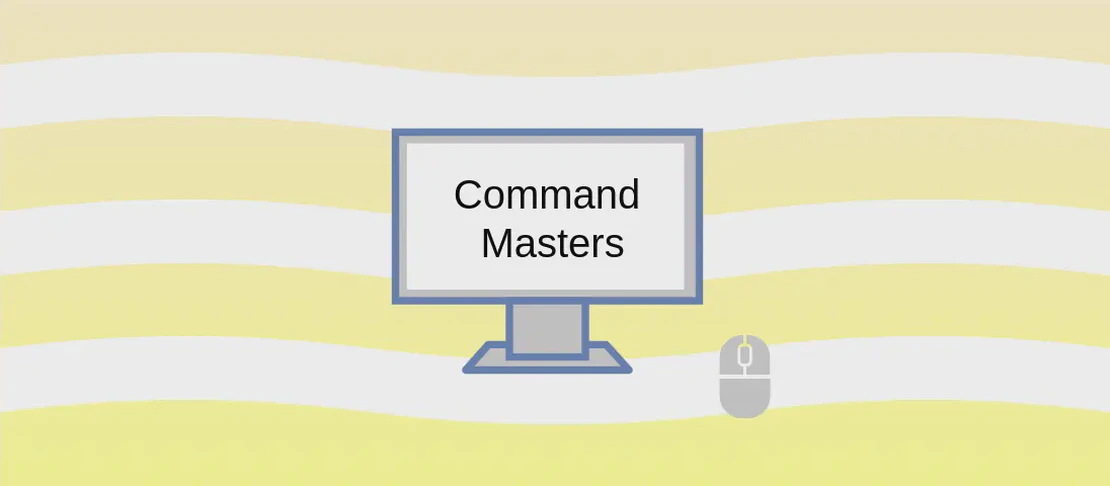
How to Use the Command 'accelerate' (with examples)
Accelerate is a powerful library that enables developers to run the same PyTorch code across various distributed configurations, simplifying the process of scaling up machine learning experiments. By utilizing Accelerate, users can seamlessly transfer their existing single-node code to a distributed environment, maximizing the efficiency of computational resources and reducing the complexity traditionally associated with distributed machine learning tasks.
Use case 1: Print environment information
Code:
accelerate env
Motivation: Before diving into complex machine learning tasks, understanding the available environment setup is crucial. This command helps verify the current system’s capabilities, ensuring that all necessary components for distributed processing or model training are present.
Explanation:
env
: This argument tells the accelerate command to print detailed information about the current computing environment. It checks for things like installed libraries, available GPUs, and other relevant configurations.
Example Output:
- PyTorch version: 1.9.0
- CUDA available: True
- Number of GPUs: 4
- Python version: 3.8
- Accelerate version: 0.4.1
Use case 2: Interactively create a configuration file
Code:
accelerate config
Motivation: When scaling machine learning tasks, having a well-defined configuration file is key. This command guides users through setting up their environment for distributed training, ensuring that all necessary parameters are correctly configured.
Explanation:
config
: Invokes the interactive mode where users will be asked to provide information about their desired configuration, such as the type of distribution strategy, the number of processes, the use of mixed-precision, and more.
Example Output:
Setting up configuration:
- Parallel processing strategy: DataParallel
- Number of processes: 8
- Use mixed-precision: No
Configuration saved to ~/.cache/huggingface/accelerate/default_config.yaml
Use case 3: Print the estimated GPU memory cost of running a Hugging Face model with different data types
Code:
accelerate estimate-memory name/model
Motivation: Efficient resource management in machine learning tasks is essential. Estimating GPU memory requirements can help choose appropriate hardware and ensure models run smoothly without encountering memory issues.
Explanation:
estimate-memory
: This subcommand calculates and displays the estimated memory usage for a pre-defined ML model.name/model
: Replace this placeholder with the actual Hugging Face model identifier you wish to estimate, such as ‘bert-base-uncased’.
Example Output:
Estimating memory requirements for bert-base-uncased:
- FP32 memory requirement: 8.4 GB
- FP16 memory requirement: 4.2 GB
- Int8 memory requirement: 2.1 GB
Use case 4: Test an Accelerate configuration file
Code:
accelerate test --config_file path/to/config.yaml
Motivation: Ensuring that the configuration file accurately represents your setup can save significant troubleshooting time. This command lets users verify that their configuration file will work as expected before starting intensive training processes.
Explanation:
test
: Initiates the configuration testing process.--config_file
: Specifies the path to the configuration file that needs testing.
Example Output:
Testing configuration: path/to/config.yaml
- Configuration is valid
- Distributed initialization successful
Use case 5: Run a model on CPU with Accelerate
Code:
accelerate launch path/to/script.py --cpu
Motivation: Not all users have access to GPUs, and sometimes smaller models can be efficiently run on a CPU. This command allows running scripts directly on CPU, making it convenient for development or smaller-scale experiments.
Explanation:
launch
: Indicates the start of a script execution using the parameters defined in the configuration file.path/to/script.py
: Path to the Python script you wish to run.--cpu
: Enforces model execution on the CPU.
Example Output:
Launching script: path/to/script.py on CPU
- Model execution completed successfully.
Use case 6: Run a model on multi-GPU with Accelerate, with 2 machines
Code:
accelerate launch path/to/script.py --multi_gpu --num_machines 2
Motivation: For large-scale machine learning tasks, utilizing multiple GPUs across different machines can drastically reduce training time and enhance performance. This command streamlines the process of deploying models across such distributed systems.
Explanation:
launch
: Initiates execution of a specified script.path/to/script.py
: Points to the desired Python script.--multi_gpu
: Specifies running across multiple GPUs.--num_machines 2
: Sets the number of machines to distribute the model workload across.
Example Output:
Launching script: path/to/script.py across 2 machines with multi-GPU setup.
- Distributed training commencing
- Epoch 1: 60% complete
Conclusion:
The ‘accelerate’ command provides a crucial bridge for those looking to optimize their machine learning workflows across diverse computational setups. From setting up configurations, validating environments, and running models across CPUs and GPUs, it simplifies and enhances the experience of scaling machine learning tasks, thus empowering researchers and engineers with flexibility and efficiency.