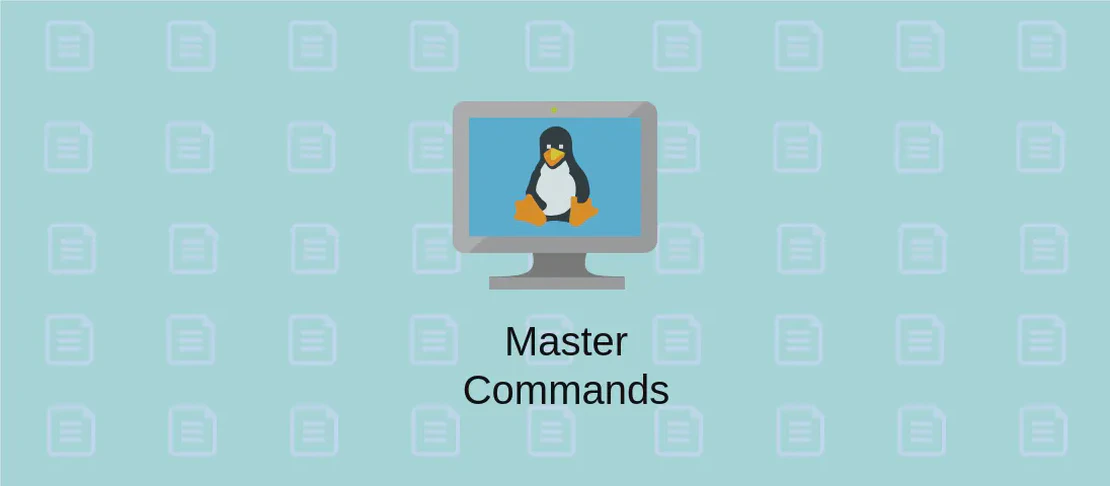
How to use the command 'adb' (with examples)
The Android Debug Bridge (adb) is a versatile command-line tool that allows developers to communicate with an Android device, whether it’s an emulator or a connected hardware device. This tool is essential for Android development as it provides the ability to install and debug apps, transfer files, and execute commands directly on the device. With adb, developers can efficiently interact with devices for various development and testing purposes.
Use case 1: Check whether the adb server process is running and start it
Code:
adb start-server
Motivation:
Starting the adb server is often the first step required before any other adb operations can be performed. The server acts as a bridge to facilitate communication between the computer and the connected Android devices or emulators. Ensuring the server is running is crucial to enabling seamless interactions like app deployments, debugs, and shell executions. By initiating or restarting the server, developers can rectify potential connectivity issues, ensuring all subsequent adb commands function correctly.
Explanation:
adb
: Invokes the Android Debug Bridge tool.start-server
: This argument tells adb to initialize the server process. If the server is not running, this command will start it, allowing future adb commands to communicate with devices.
Example Output:
* daemon not running; starting now at tcp:5037
* daemon started successfully
This output indicates that the adb server was not running initially, and the command successfully started it.
Use case 2: Terminate the adb server process
Code:
adb kill-server
Motivation:
Terminating the adb server can be useful when you want to reset the adb connection, troubleshoot connectivity issues, or ensure a clean exit of the server when no longer needed. Regularly stopping the server can help prevent potential conflicts or persistent connection errors that may arise from a continuously running adb server process.
Explanation:
adb
: Invokes the Android Debug Bridge tool.kill-server
: This command stops the adb server process that manages connections between the computer and Android devices.
Example Output:
* server not running *
This output indicates that the command successfully terminated the adb server, or that it was not running initially.
Use case 3: Start a remote shell in the target emulator/device instance
Code:
adb shell
Motivation:
Accessing a remote shell on Android devices or emulators is invaluable for developers and testers who need to interact with the device’s operating system directly. This can include tasks such as checking file directories, running commands to get device information, managing apps, or troubleshooting issues that require direct command-line intervention.
Explanation:
adb
: Invokes the Android Debug Bridge tool.shell
: This argument opens a terminal session with the Android device’s command-line shell environment, allowing the user to execute shell commands directly on the device.
Example Output:
shell@android:/ $
This output represents a command-line prompt that indicates you have successfully entered the Android device’s shell environment.
Use case 4: Push an Android application to an emulator/device
Code:
adb install -r path/to/file.apk
Motivation:
Installing applications onto an Android device for testing or deployment is a fundamental task in Android development. This use case covers how to automate the deployment of an updated APK onto a device, especially useful during iterative development phases when frequent app installations occur. It makes the process seamless, particularly with the -r
option to reinstall existing apps without the hassle of manual uninstallation.
Explanation:
adb
: Invokes the Android Debug Bridge tool.install
: This command is used to install an APK file onto the Android device.-r
: This flag indicates that the existing APK on the device should be replaced if it’s already installed (reinstall).path/to/file.apk
: This specifies the file path to the APK file that needs to be installed.
Example Output:
Performing Streamed Install
Success
This output signifies that the APK was successfully installed or reinstalled on the device.
Use case 5: Copy a file/directory from the target device
Code:
adb pull path/to/device_file_or_directory path/to/local_destination_directory
Motivation:
Transferring files from an Android device to a local machine is crucial for tasks like retrieving logs, configuration files, or media that needs analysis or backup. Developers often use this process to extract data for debugging, app testing, or other purposes such as recovering user data. The versatility of adb in facilitating these transfers seamlessly makes it an invaluable tool for efficient device file system management.
Explanation:
adb
: Invokes the Android Debug Bridge tool.pull
: This command copies files or directories from the Android device to the local machine.path/to/device_file_or_directory
: Specifies the path on the device from which the file or directory should be pulled.path/to/local_destination_directory
: Designates the destination path on the local machine where the file or directory will be stored.
Example Output:
/sdcard/file.txt: 1 file pulled. 0.3 MB/s (1024 bytes in 0.003s)
This output indicates the successful transfer of the specified file from the device to the local machine.
Use case 6: Copy a file/directory to the target device
Code:
adb push path/to/local_file_or_directory path/to/device_destination_directory
Motivation:
Pushing files or directories from a local machine to an Android device is essential for deployment, app setup, or configuring environments for testing. Developers might need to transfer asset files, configuration settings, or test data to an app directory on the device. Using adb for this purpose ensures quick and reliable file delivery straight into the Android device’s ecosystem, boosting efficiency during the development cycle.
Explanation:
adb
: Invokes the Android Debug Bridge tool.push
: This command copies files or directories from the local machine to the Android device.path/to/local_file_or_directory
: Specifies the local file or directory path that needs to be pushed to the device.path/to/device_destination_directory
: Identifies the destination path on the device where the file or directory will be copied.
Example Output:
file.txt: 1 file pushed. 0.1 MB/s (1024 bytes in 0.010s)
This output confirms the successful transfer of the specified file from the local machine to the Android device.
Use case 7: List all connected devices
Code:
adb devices
Motivation:
Listing connected devices is crucial for ensuring that your computer correctly recognizes and communicates with all plugged-in Android devices or running emulators. This step is especially vital in environments with multiple devices or when diagnosing connectivity issues. Developers and testers rely on this command to verify connections before proceeding with more detailed device interactions or tests.
Explanation:
adb
: Invokes the Android Debug Bridge tool.devices
: This argument outputs a list of all connected Android devices and emulators recognized by adb.
Example Output:
List of devices attached
emulator-5554 device
0123456789ABCDEF device
This output lists the connected device identifiers, confirming their availability for further commands.
Conclusion:
The adb command is a powerful utility that provides essential capabilities required for Android development and device management. Each use case outlined above demonstrates practical tasks that developers frequently encounter, highlighting the versatility of adb in handling various operations such as device communication, app installation, file transfers, and more. With a deep understanding and effective utilization of adb commands, developers can significantly enhance their workflow, ensuring smoother interactions with Android devices.