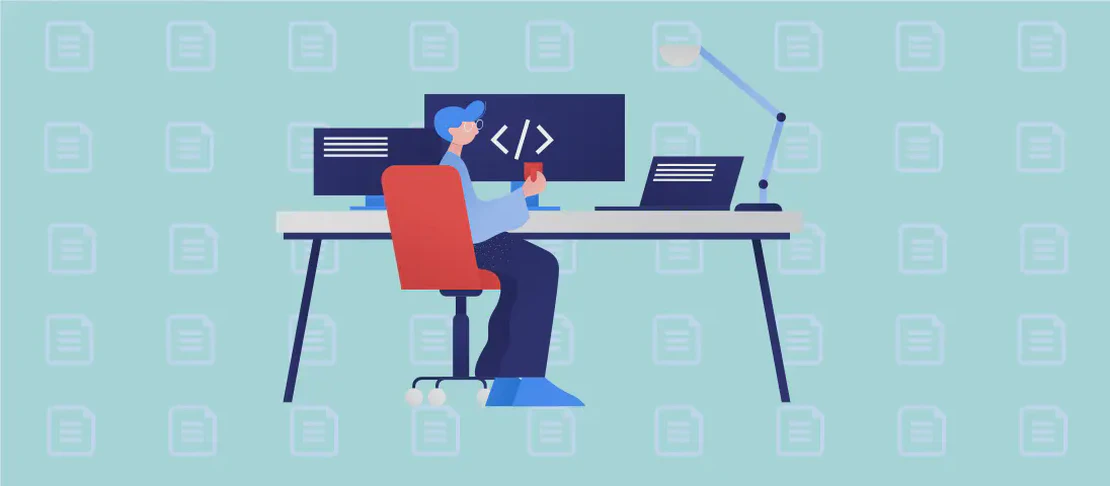
How to use the command 'adb install' (with examples)
The adb install
command is part of the Android Debug Bridge (ADB), a versatile tool allowing developers to communicate and control Android devices. One of its primary functionalities is to install Android applications directly onto an emulator or a physical Android device. This tool is exceptionally useful for developers who need to test applications quickly and efficiently without navigating through the usual install process on an Android device. The command comes with a variety of options to cater to different installation scenarios, making it an indispensable tool for Android developers.
Use Case 1: Push an Android application to an emulator/device
Code:
adb install path/to/file.apk
Motivation:
This is the most straightforward use case where a developer wants to install an Android application (.apk file) onto a connected device or emulator from their development machine. This command is highly beneficial during the app development and testing phase as it simplifies the process of deploying builds directly to the device.
Explanation:
adb
: The Android Debug Bridge command-line tool.install
: The command option specified to install the Android application.path/to/file.apk
: This represents the path to the apk file that needs to be pushed to the device or emulator.
Example Output:
Performing Streamed Install
Success
Use Case 2: Push an Android application to a specific emulator/device (overrides $ANDROID_SERIAL
)
Code:
adb -s serial_number install path/to/file.apk
Motivation:
In scenarios where there are multiple devices and emulators connected to the development machine, it is crucial to specify which device or emulator should receive the installation. By specifying the serial number, developers avoid inadvertently installing the application on an unintended device.
Explanation:
-s serial_number
: This option sets the target for the installation by specifying the serial number of the device or emulator.install
: The subcommand to perform the installation.path/to/file.apk
: The path to the .apk file to be installed.
Example Output:
Performing Streamed Install
Success
Use Case 3: [r]einstall an existing app, keeping its data
Code:
adb install -r path/to/file.apk
Motivation:
During the development process, developers often need to install new builds over the existing version of an application while retaining the user data. This is particularly useful during iterative testing when preserving app settings and user progress is vital.
Explanation:
-r
: The reinstall flag allows the application to be installed over existing instances while preserving the app’s data.install
: Specifies the installation subcommand.path/to/file.apk
: The file path to the new version of the application package.
Example Output:
Performing Streamed Install
Success
Use Case 4: Push an Android application allowing version code [d]owngrade (debuggable packages only)
Code:
adb install -d path/to/file.apk
Motivation:
Sometimes, developers need to test older versions of an application to check for compatibility or bugs introduced by newer features. Utilizing the ability to downgrade versions allows these re-testing efforts without having to uninstall and clear data, ensuring a smoother workflow.
Explanation:
-d
: This option allows the installation of an apk with a lower version code than the one currently installed, but only for debuggable apps.install
: The command option for installation.path/to/file.apk
: The filepath leading to the APK that is to be downgraded.
Example Output:
Performing Streamed Install
Success
Use Case 5: [g]rant all permissions listed in the app manifest
Code:
adb install -g path/to/file.apk
Motivation:
This is increasingly important due to Android’s stringent permission requirements. By granting all permissions, developers ensure that the app behaves as expected during testing without manually navigating through permission requests on the device.
Explanation:
-g
: This flag grants the runtime permissions specified in the app’s manifest file upon installation.install
: Indicates the install operation.path/to/file.apk
: The path to the application package file.
Example Output:
Performing Streamed Install
Success
Use Case 6: Quickly update an installed package by only updating the parts of the APK that changed
Code:
adb install --fastdeploy path/to/file.apk
Motivation:
For developers making frequent small changes, the --fastdeploy
option saves considerable time by only updating parts of the APK that have been modified. This expedites the testing cycle, enhancing productivity and efficiency.
Explanation:
--fastdeploy
: This option optimizes the installation process by only deploying the changed parts of the APK.install
: The command used to trigger installation.path/to/file.apk
: This specifies the location of the APK file.
Example Output:
Performing Incremental Install
Success
Conclusion:
Understanding and utilizing the adb install
command effectively can significantly streamline the Android development process. Whether handling multiple devices, preserving data across app versions, allowing easier permission handling, or performing quick updates, these use cases demonstrate the robustness and flexibility of the tool. Mastery of these commands equips developers to optimize their workflow and productivity during Android application development.