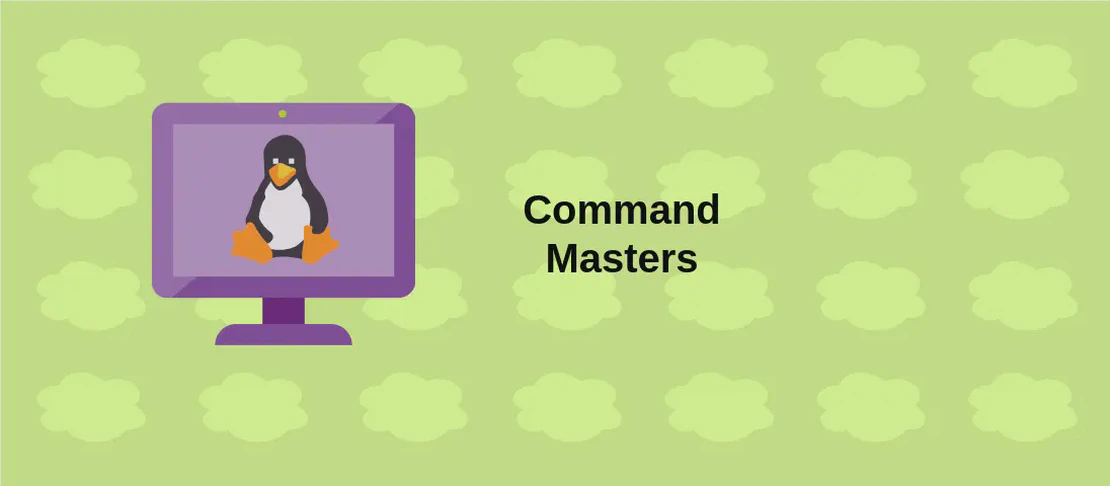
How to Use the Command 'adb logcat' (with Examples)
The Android Debug Bridge (adb) command logcat
is a valuable tool for developers and testers, providing a way to interactively view logs of system messages, including stack traces when your app throws an error, informational messages that you might have publicly available, or other logs that might be useful for debugging purposes. adb logcat
is most commonly used by Android developers for troubleshooting and analyzing application behavior during testing by examining logs generated by an Android device or emulator.
Use case 1: Display System Logs
Code:
adb logcat
Motivation:
Viewing complete system logs is often the first step when troubleshooting issues on an Android device. This comprehensive view allows developers to understand the flow of events before and after an error occurs or while monitoring general system behavior.
Explanation:
adb
: Stands for Android Debug Bridge, a versatile command-line tool that lets you communicate with a device.logcat
: Retrieves and displays logs from the connected Android device. Without additional arguments, it outputs all logs.
Example Output:
The output will be the continuous logging of all system activities and application operations in real-time. Here is a simplified snippet:
01-01 12:00:01.234 1234 5678 I ActivityManager: Start proc com.example.app for activity com.example/.MainActivity
01-01 12:00:02.345 1234 5678 W System.err: java.lang.NullPointerException
Use case 2: Display Lines that Match a Regular Expression
Code:
adb logcat -e regular_expression
Motivation:
Using regular expressions to filter logs is powerful when you want to pinpoint specific events or messages that match certain criteria. For instance, developers can use this to find error messages or debug logs specific to a custom keyword or pattern.
Explanation:
-e
: This flag allows logcat to filter the output so that it only includes lines that match the provided regular expression. It helps to reduce noise by focusing on relevant logs.
Example Output:
When searching for the term “NetworkError”, for instance, the filtered output might look similar to:
01-01 12:05:30.699 2345 6789 E NetworkManager: NetworkError: connection failed
Use case 3: Display Logs for a Tag in a Specific Mode
Code:
adb logcat tag:mode *:S
Motivation:
Tags in logs are identifiers added by developers to easily track different components or actions within an app. By filtering logs by a specific tag and log level (mode), developers can focus on particular operations or issues.
Explanation:
tag:mode
: Filters the logs by a specific tag and log priority. Modes include Verbose (V), Debug (D), Info (I), Warning (W), Error (E), Fatal (F), and Silent (S).*:S
: Silences all other tags to ensure that only the specified tag’s logs are displayed.
Example Output:
For instance, filtering by “MyApp:W” might show:
01-01 12:15:30.499 3456 7890 W MyApp: Warning: Low memory detected
Use case 4: Display Logs for React Native Applications in Verbose Mode, Silencing Other Tags
Code:
adb logcat ReactNative:V ReactNativeJS:V *:S
Motivation:
React Native developers need a way to monitor JavaScript-side logs and detailed operational info. This command isolates React Native-specific logs, making it useful for debugging both the React Native and JS layers.
Explanation:
ReactNative:V
: Verbosely logs all React Native framework-related actions.ReactNativeJS:V
: Captures and displays even the most minor operations and messages from the JavaScript side of a React Native app.*:S
: Ensures that no other logs clutter the focus on React Native activity by silencing all other tags.
Example Output:
Logs might show debug information from both React Native and JS:
01-01 12:20:50.499 4567 8901 V ReactNative: Running application "MyReactApp" with appParams: {...}
Use case 5: Display Logs for All Tags with Priority Level Warning and Higher
Code:
adb logcat *:W
Motivation:
This command is beneficial when you want to see potential issues that have been flagged by the system or application without getting drowned out by lower priority logs like info and debug messages, which tend to be more verbose but less critical.
Explanation:
*:W
: This sets the default log level toWarning
, meaning that all logs of level Warning and above (Error, Fatal) will be displayed, reducing clutter from less concerning log messages.
Example Output:
01-01 12:30:45.123 5678 9012 W BatteryManager: Battery level is low
01-01 12:31:12.321 5678 9012 E SystemService: Unable to fetch data
Use case 6: Display Logs for a Specific PID
Code:
adb logcat --pid pid
Motivation:
Focusing on logs for a specific Process ID (PID) can be crucial when debugging a particular application process without interference from other processes. This is especially helpful when working with apps that generate a large number of logs.
Explanation:
--pid pid
: Filters logs to display only those that are associated with the specified process ID, allowing for isolated examination.
Example Output:
Assuming PID 12345 is your app process PID, the logs would be strictly from processes with this ID:
01-01 12:35:40.678 12345 5678 I MyApp: Application started
Use case 7: Display Logs for the Process of a Specific Package
Code:
adb logcat --pid $(adb shell pidof -s package)
Motivation:
Automatically obtaining and using the PID of a running package streamlines the process of filtering logs for a specific application. This method can quickly direct attention to a particular app, especially useful when working with complex applications with multiple packages.
Explanation:
adb shell pidof -s package
: Fetches the Process ID of the specified package name.--pid
: Filters logs based on this fetched PID to show logs relevant to the app only.
Example Output:
If “com.example.app” is the package, logs may include:
01-01 12:40:50.456 2345 6789 I ActivityManager: Displayed com.example.app/.MainActivity
Use case 8: Color the Log
Code:
adb logcat -v color
Motivation:
Color-coding logs can significantly improve readability by distinguishing between different log levels and log sources visually. This is particularly helpful during long debugging sessions.
Explanation:
-v color
: Verbose mode with color highlights, enabling easier identification and separation of log levels through distinct colors for each log type (INFO, WARN, ERROR, etc.).
Example Output:
Expected output will be similar text logs but with enhanced visual distinction:
- INFO messages in a different hue than WARN or ERROR, making it visually simpler to navigate through logs.
Conclusion:
The adb logcat
command is a critical tool for Android developers and testers. By understanding and utilizing the various options and filters available with adb logcat
, developers can effectively monitor application behavior, diagnose issues, and optimize the testing and development process. Each use case presented offers a different way to precisely target the information needed from the vast array of logs generated by an Android device, thereby enhancing efficiency and effectiveness in app development and debugging sessions.