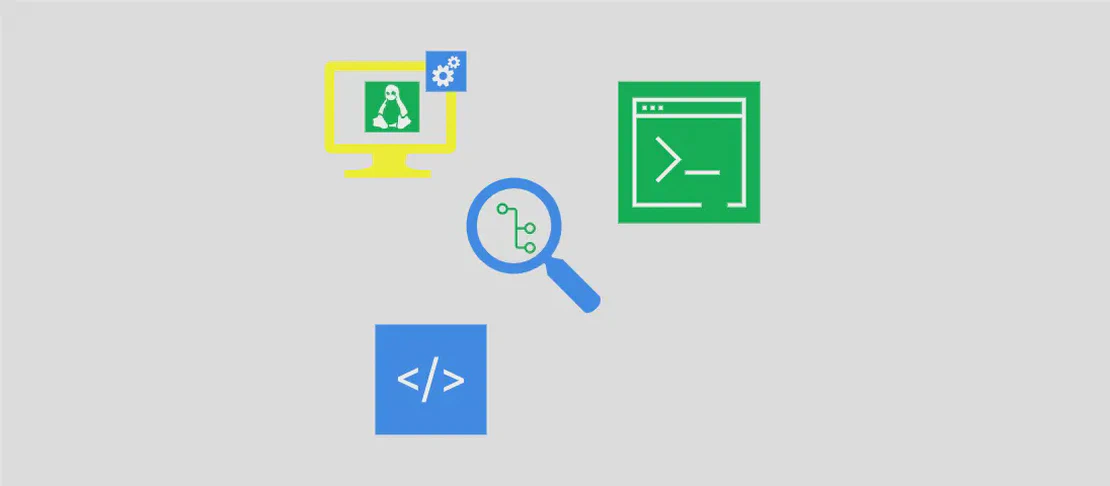
How to Use the Command 'adb shell' (with Examples)
The adb shell
command is an essential tool for Android developers and power users alike. It allows you to run a variety of shell commands on a connected Android device or emulator directly from your computer. This capability enables you to interact with the Android system, execute scripts, automate tasks, and debug applications without directly accessing the device’s screen. The Android Debug Bridge (ADB) is a versatile command-line tool that facilitates communication between your computer and any Android device. Here are some practical use cases demonstrating the effectiveness and versatility of adb shell
.
Use case 1: Start a Remote Interactive Shell on the Emulator or Device
Code:
adb shell
Motivation:
Starting a remote interactive shell allows developers and users to execute commands on the Android device in real time. This means you can change file permissions, view logs, test shell scripts, and do much more as if you were using the terminal directly on the device. It’s particularly useful for debugging and automation purposes.
Explanation:
The basic adb shell
command establishes a connection and opens an interactive shell interface on the device. This enables direct interaction with the Android operating system, providing the same access as accessing a Linux command line on the device.
Example Output:
shell@android:/ $
This prompt indicates that you are now operating in the device’s shell environment, ready to execute commands.
Use case 2: Get All the Properties from Emulator or Device
Code:
adb shell getprop
Motivation:
Retrieving all system properties can offer valuable insights into the device’s configuration, app environment, and performance characteristics. This command is beneficial for developers looking to ensure compatibility or require environmental context while troubleshooting.
Explanation:
The getprop
command fetches a list of all system properties and their values on the device. These include information about network settings, hardware capabilities, system version, and other configuration settings.
Example Output:
[ro.build.version.sdk]: [30]
[ro.product.model]: [Pixel_3a]
[ro.build.fingerprint]: [google/sargo]
Each line provides a key-value pair representing a property name and its corresponding value.
Use case 3: Revert All Runtime Permissions to Their Default
Code:
adb shell pm reset-permissions
Motivation:
Over time, as apps request permissions and settings are modified, it can become cumbersome to ensure that permissions reflect the default or desired state. This command is useful for developers and testers who need to start from a clean state regarding app permissions.
Explanation:
The pm reset-permissions
command resets the permissions that the user has granted to apps back to their original state, as when they were first installed. This allows developers to verify permission request logic in apps.
Example Output:
Package android reset
Package com.example.myapp reset
Each package listed indicates that its permissions have been reverted.
Use case 4: Revoke a Dangerous Permission for an Application
Code:
adb shell pm revoke package permission
Motivation:
When testing or debugging applications, developers might need to revoke specific permissions to observe how their app handles scenarios where certain permissions are denied. This command is critical for ensuring robust application behavior.
Explanation:
pm revoke
: Package Manager (pm) service command to revoke a specific permission.package
: Name of the application package, e.g.,com.example.myapp
.permission
: The permission being revoked, e.g.,android.permission.CAMERA
.
Example Output:
Permission android.permission.CAMERA revoked from package com.example.myapp
This confirms that the specified permission has been successfully revoked.
Use case 5: Trigger a Key Event
Code:
adb shell input keyevent keycode
Motivation:
Simulating key events can be valuable when automating tests or simulating user interactions on a device. It’s particularly useful for navigating the UI without physically interacting with the device.
Explanation:
input keyevent
: Executes a key event command.keycode
: The code of the key event you want to simulate, such asKEYCODE_HOME
for the home button.
Example Output:
There is typically no console output; the device simply registers the key event, such as returning to the home screen if KEYCODE_HOME
was used.
Use case 6: Clear the Data of an Application on an Emulator or Device
Code:
adb shell pm clear package
Motivation:
Clearing an application’s data can be essential for testing app behavior upon fresh installs or resetting the app to a clean state without having to uninstall and reinstall it. This is also useful for freeing up storage space.
Explanation:
pm clear
: Clears all user data associated with a particular app.package
: Refers to the specific application package you’re targeting, such ascom.example.myapp
.
Example Output:
Success
This output indicates that all data associated with the specified app has been successfully cleared.
Use case 7: Start an Activity on Emulator or Device
Code:
adb shell am start -n package/activity
Motivation:
Manually launching an app component like an activity is crucial when testing specific parts of an app without navigating through the entire user interface. This saves time and enhances efficiency for developers and testers.
Explanation:
am start
: Activity Manager (am) tool command to start an activity.-n
: Specifies the target component, formatted aspackage/activity
.
Example Output:
Starting: Intent { cmp=com.example.myapp/.MainActivity }
This communicates that the specified activity within the package has been successfully started.
Use case 8: Start the Home Activity on an Emulator or Device
Code:
adb shell am start -W -c android.intent.category.HOME -a android.intent.action.MAIN
Motivation:
Directing the device to navigate to the home screen serves as a baseline for many automation scripts and tests. It ensures the device interface is in a consistent state before the execution of further commands or tests.
Explanation:
am start
: Uses the Activity Manager to start processes.-W
: Waits for the launch to complete.-c android.intent.category.HOME
: Sets the category to Home, directing the launcher.-a android.intent.action.MAIN
: Sets the action to MAIN, which matches the entry point for launching tasks.
Example Output:
Status: ok
Activity: com.android.launcher/com.android.launcher2.Launcher
Indicates that the launcher activity, effectively displaying the home screen, has been activated.
Conclusion:
The adb shell
command unlocks a vast spectrum of functionalities for interacting with Android devices effectively from the command line. Each use case demonstrates a distinct and practical application of the tool, from debugging to automation, setting development baselines, and more. Understanding these can significantly enhance the productivity and capability of developers working with Android environments.