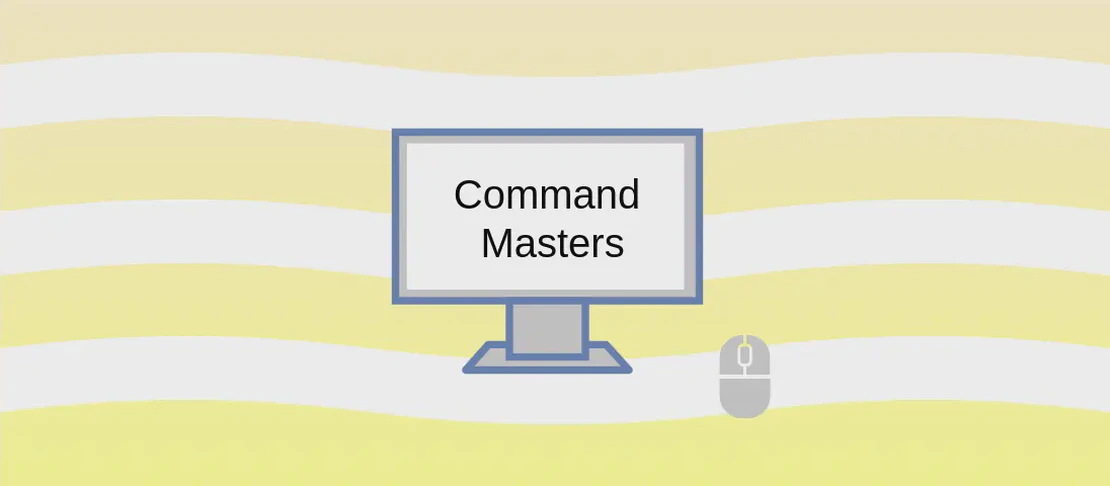
Using addr2line for Source Code Debugging (with examples)
- Linux
- December 17, 2024
addr2line
is a powerful command-line tool used by developers and software engineers to translate program execution addresses into meaningful file names and line numbers within the source code. This tool is particularly useful for debugging purposes, allowing users to trace the origin of a program’s instruction within the source code. By utilizing this tool, developers can quickly identify the location of problematic code, which facilitates efficient debugging and code analysis.
Use Case 1: Display the filename and line number of the source code from an instruction address of an executable
Code:
addr2line --exe=path/to/executable address
Motivation:
When encountering a bug or a crash in an application, it often produces a stack trace that includes a bunch of memory addresses. By using addr2line
, developers can convert these addresses into specific lines of source code, making it easier to pinpoint the exact location of the error. This is essential for debugging complex applications, where manually searching through code files would be labor-intensive and time-consuming.
Explanation:
--exe=path/to/executable
: Specifies the path to the executable file that the addresses need to be translated from. This tellsaddr2line
which compiled binary to use for determining the file and line numbers.address
: The actual memory address that needs to be translated into human-readable format showing the source code line.
Example Output:
/home/user/project/src/main.c:57
This output informs the developer that the instruction at the given memory address corresponds to line 57 in the file main.c
located in the specified path.
Use Case 2: Display the function name, filename, and line number
Code:
addr2line --exe=path/to/executable --functions address
Motivation:
In addition to finding out where in the source code a problem lies, knowing the function name provides extra context that is invaluable during debugging. Understanding which particular function contains the problematic code helps developers quickly grasp the surrounding logic without having to trace back through potentially hundreds of lines of code.
Explanation:
--exe=path/to/executable
: As with the previous example, this pointsaddr2line
to the correct executable file.--functions
: This flag extends the basic functionality ofaddr2line
by including function names in the output alongside file names and line numbers.address
: The memory address that is to be translated to its corresponding location and function name in the source code.
Example Output:
foo_function /home/user/project/src/main.c:57
In this output, not only does the developer know that line 57 in main.c
is relevant, but also that it is within the foo_function
, providing an extra layer of insight.
Use Case 3: Demangle the function name for C++ code
Code:
addr2line --exe=path/to/executable --functions --demangle address
Motivation:
C++ compilers often mangle function names, which means they encode additional information like argument types into the function names. This allows for function overloading, template instantiation, and more. However, it obfuscates function names when viewed in a stack trace or via debugging tools. The --demangle
option is crucial for converting these mangled names back into human-readable C++ identifiers, enabling developers to understand the function signatures and namespaces properly involved.
Explanation:
--exe=path/to/executable
: Directsaddr2line
to the binary file that the addresses are associated with.--functions
: Ensures that the output includes the function name, in addition to file names and line numbers.--demangle
: A specific option for C++ code which translates mangled function names back to their original form so that they are readable and understandable.address
: The address that requires translation to provide detailed source code information including properly readable function names.
Example Output:
myNamespace::FooClass::barFunction(int, float) /home/user/project/src/main.cpp:147
Here, the output shows a demangled function name, myNamespace::FooClass::barFunction(int, float)
, providing clarity on the exact function, its parameters, and its namespace—all critical for C++ developers.
Conclusion:
addr2line
is a potent utility for debugging and understanding the execution of compiled programs by translating machine-specific addresses into human-readable source information. Through various options, such as including function names and demangling them for C++ code, addr2line
ensures that developers can discern with precision where errors occur, thus streamlining the debugging process and enhancing code maintenance and readability.