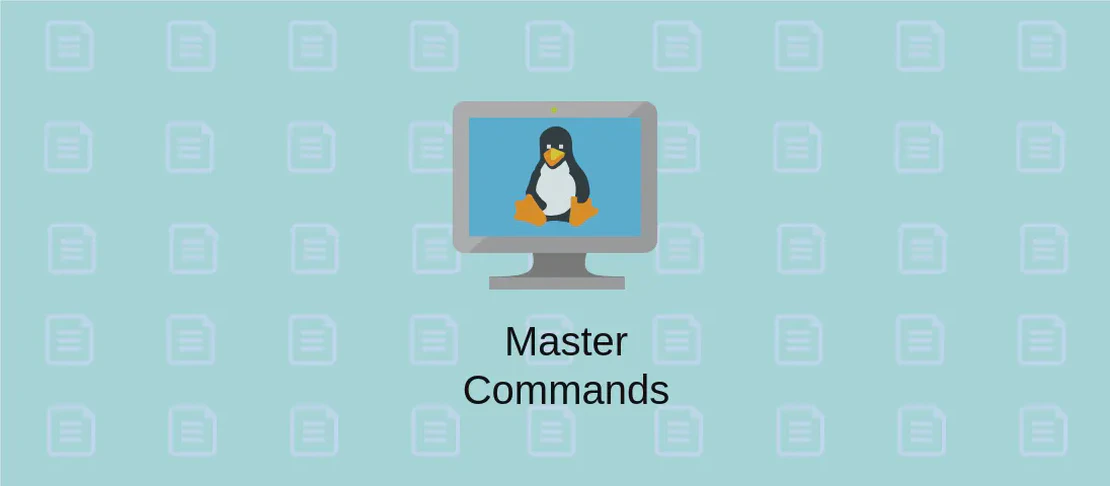
How to use the command 'am' (with examples)
- Android
- December 17, 2024
The am
command, or Android Activity Manager, is a powerful tool used to perform various actions related to Android app tasks, intents, and more. It provides developers and testers with the capability to automate activities on Android devices, allowing them to start apps, send intents, and even convert intents into URIs for deeper integration. Implementing am
commands in Android development aids in testing, automating tasks, and improving operational efficiency when handling various activities or components of an application.
Use case 1: Start the activity with a specific component and package name
Code:
am start -n com.android.settings/.Settings
Motivation for using this example: Imagine you’re a developer testing different settings within an Android application, or perhaps a user who frequently accesses specific settings. Instead of navigating multiple layers within a device, directly launching the desired settings panel using a direct command can save a significant amount of time and clicks—especially beneficial in automated scripts for testing setup.
Explanation:
am start
: This instructs the Activity Manager to begin a new activity.-n com.android.settings/.Settings
: The-n
flag specifies both the package and the specific component name that you’d like to launch. Here,com.android.settings
refers to the entire Settings app, and.Settings
points to a particular component or activity within that app, typically the main settings interface.
Example output: Upon executing the command, the device immediately opens the Android Settings application, specifically landing on the general settings page without requiring any user interaction through the device’s interface.
Use case 2: Start an intent action and pass data to it
Code:
am start -a android.intent.action.VIEW -d tel:123
Motivation for using this example: Developers and testers may need to ensure that an application correctly handles specific intents, such as dialing a call. This can be a powerful way to mimic a user action to verify that applications respond adequately to system intents, enhancing the end-user experience and ensuring robustness of the integration.
Explanation:
am start
: Again, this tells the system to start an activity specified by an intent.-a android.intent.action.VIEW
: The-a
flag defines the action to be performed.android.intent.action.VIEW
is a common action used to request the viewing of something that is shown in another application (here, it’s a phone number).-d tel:123
: The-d
flag is for passing data, such as a URI. In this command,tel:123
indicates a phone number to be used in conjunction with the VIEW action.
Example output: On running this command, the device will open the dialer application and populate the number “123” into the dial pad, ready to be called, simulating part of the call flow process without needing manual input.
Use case 3: Start an activity matching a specific action and category
Code:
am start -a android.intent.action.MAIN -c android.intent.category.HOME
Motivation for using this example: Opening the home screen or launching the primary application screen directly is often required in testing workflows, especially when returning to a known state like the home launcher. It ensures a consistent test environment when applications need to be run from the default state or interacting with the device’s primary functions.
Explanation:
am start
: Directs the Activity Manager to initiate an activity.-a android.intent.action.MAIN
: Here, the-a
flag sets the action to MAIN, which signifies the main entry point for launching an activity.-c android.intent.category.HOME
: Using-c
specifies a category within that action.android.intent.category.HOME
directs the system to open the home screen or launcher, which is useful when resetting to a known state as part of a suite of automated tests.
Example output: Executing this command returns the device to its initial home screen or primary launcher, mimicking the button press of the home key, which is particularly useful during sequential testing sessions.
Use case 4: Convert an intent to a URI
Code:
am to-uri -a android.intent.action.VIEW -d tel:123
Motivation for using this example: URI conversion for an intent can be particularly useful for debugging and logging purposes. By representing an intent as a URI, developers can easily store, modify, or transfer intent data within databases or over networks, providing a versatile and readable format for further processing in applications or troubleshooting procedures.
Explanation:
am to-uri
: This command triggers the conversion of an intent into a URI format.-a android.intent.action.VIEW
: As in previous examples, the-a
flag specifies the action portion of the intent, in this case, VIEW.-d tel:123
: The-d
flag represents the data contained in the intent, here denoting a number to be transformed into URI format for further potential use cases.
Example output:
The command outputs a URI string representing the intent, such as intent:#Intent;action=android.intent.action.VIEW;data=tel:123;end
, which can be readily visualized and leveraged in applications for intent processing or storage.
Conclusion:
The am
command is an extremely versatile tool within the Android ecosystem, providing developers with a means to automate, test, and utilize Android devices more effectively. Each use case—from launching specific activities to converting intents into URIs—demonstrates how am
can be implemented to enhance development workflows, improve testing efficiency, and facilitate seamless integration across Android applications and devices. Through these examples, the range and capability of am
in simplifying complex processes becomes evidently impactful.