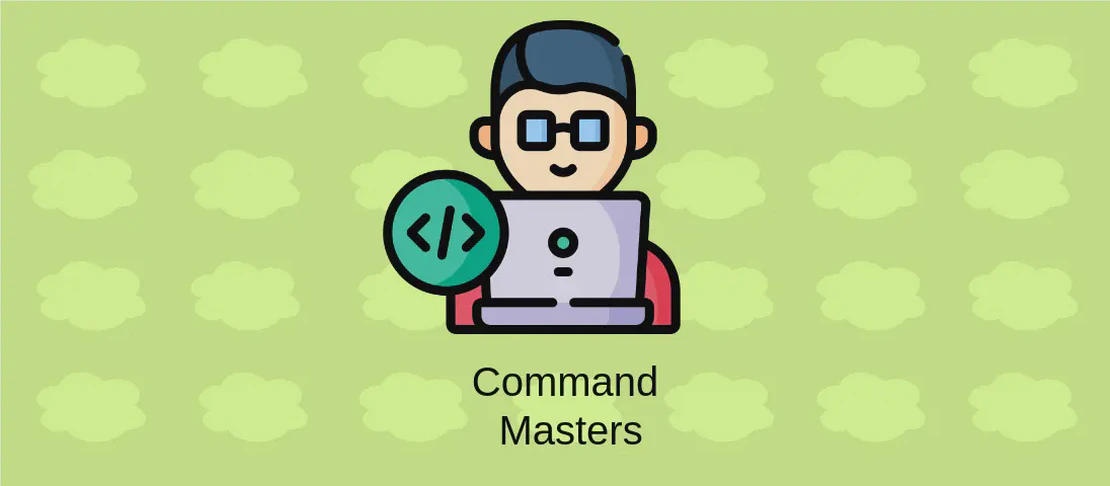
How to Use the Command 'Ansible' (with Examples)
Ansible is a powerful automation tool designed to streamline the management of groups of computers remotely through SSH (Secure Shell). It provides a simple way to automate repetitive IT tasks like configuration management, application deployment, and task automation on Windows and Unix-based systems. It’s agentless, making it lightweight and easy to set up. Ansible is known for its straightforward language and concise syntax, making it accessible for users and organizations that wish to automate infrastructure management.
Use Case 1: List Hosts Belonging to a Group
Code:
ansible group --list-hosts
Motivation:
This command is useful for understanding the composition of a group before performing automation tasks on it. By listing all the hosts in a specified group, an administrator can verify that the inventory is correctly configured and all hosts are accounted for.
Explanation:
ansible
: This is the command that invokes the Ansible tool.group
: The group whose hosts you want to list. You would replace ‘group’ with the actual name of the group.--list-hosts
: This argument instructs Ansible to list all the hosts within the specified group.
Example Output:
hosts (3):
host1.example.com
host2.example.com
host3.example.com
Use Case 2: Ping a Group of Hosts by Invoking the Ping Module
Code:
ansible group -m ping
Motivation:
Pinging a group of hosts is essential to verify connectivity and ensure that Ansible can communicate with all hosts before deploying any changes or running tasks. This ensures the infrastructure is ready for automation processes.
Explanation:
ansible
: Calls Ansible to execute the command.group
: Specifies the group of hosts the command targets.-m ping
: The-m
option specifies the module to run, in this case, the ‘ping’ module, which checks connectivity by sending a simple ping command to each host.
Example Output:
host1.example.com | SUCCESS => {
"changed": false,
"ping": "pong"
}
host2.example.com | SUCCESS => {
"changed": false,
"ping": "pong"
}
host3.example.com | SUCCESS => {
"changed": false,
"ping": "pong"
}
Use Case 3: Display Facts About a Group of Hosts by Invoking the Setup Module
Code:
ansible group -m setup
Motivation:
Gathering facts about your systems is critical for understanding their current configuration, capacity, and state. With this information, administrators can make informed decisions regarding resource allocation, system scaling, and configuration management.
Explanation:
ansible
: Initiates the Ansible session.group
: Denotes the target group of hosts to gather facts from.-m setup
: Uses the ‘setup’ module, which retrieves comprehensive details about the system, including hardware and network configurations, installed software, and more.
Example Output:
host1.example.com | SUCCESS => {
"ansible_facts": {
"architecture": "x86_64",
"distribution": "Ubuntu",
"fqdn": "host1.example.com",
"interfaces": ["eth0", "lo"],
...
}
}
Use Case 4: Execute a Command on a Group of Hosts by Invoking the Command Module with Arguments
Code:
ansible group -m command -a 'my_command'
Motivation:
Executing commands across multiple hosts simultaneously can significantly expedite routine administrative tasks, such as updating packages or restarting services, thereby enhancing operational efficiency.
Explanation:
ansible
: Calls Ansible to start the execution.group
: Targets the specified group of hosts.-m command
: Invokes the ‘command’ module to run a command on the hosts.-a 'my_command'
: Provides arguments to the command module, where ‘my_command’ represents the command you wish to execute.
Example Output:
host1.example.com | CHANGED | rc=0 >>
Output of my_command
host2.example.com | CHANGED | rc=0 >>
Output of my_command
Use Case 5: Execute a Command with Administrative Privileges
Code:
ansible group --become --ask-become-pass -m command -a 'my_command'
Motivation:
Certain administrative tasks require elevated privileges. Using the --become
option allows safe execution of commands that modify critical system configurations, ensuring adherence to security protocols.
Explanation:
ansible
: Starts the Ansible command.group
: Specifies the group of hosts.--become
: Enables privilege escalation, allowing execution with higher privileges (e.g., root).--ask-become-pass
: Prompts for the password necessary to escalate privileges.-m command -a 'my_command'
: Executes ‘my_command’ on all hosts in the group.
Example Output:
BECOME password:
host1.example.com | CHANGED | rc=0 >>
Output of my_command with elevated privileges
Use Case 6: Execute a Command Using a Custom Inventory File
Code:
ansible group -i inventory_file -m command -a 'my_command'
Motivation:
Custom inventory files are handy when managing multiple environments (e.g., development, testing, production) with different sets of hosts. This way, automation tasks are executed precisely on the intended environment.
Explanation:
ansible
: Begins the Ansible session.group
: The group within the custom inventory file to target.-i inventory_file
: Specifies the path to an alternative inventory file.-m command -a 'my_command'
: Instructs the command module to run ‘my_command’ on the hosts.
Example Output:
host1.example.com | CHANGED | rc=0 >>
Output of my_command using custom inventory
Use Case 7: List the Groups in an Inventory
Code:
ansible localhost -m debug -a 'var=groups.keys()'
Motivation:
Listing the groups in an inventory allows administrators to quickly overview and verify the current organizational structure of hosts, which is necessary for efficient system management and planning.
Explanation:
ansible
: Starts the Ansible process.localhost
: Targets the localhost since the task involves reading inventory, not remote execution.-m debug
: Utilizes the ‘debug’ module to display information.-a 'var=groups.keys()'
: Outputs the keys or group names from the inventory.
Example Output:
localhost | SUCCESS => {
"groups.keys()": [
"webservers",
"databases",
"loadbalancers"
]
}
Conclusion:
Ansible provides a versatile and efficient means to manage and automate system configurations across large groups of computers with minimal effort. Its simple syntax and wide-ranging capabilities make it a staple in IT and DevOps environments, promoting consistency, reliability, and speed in managing complex infrastructure. By understanding and utilizing the various Ansible commands demonstrated in this article, system administrators can greatly enhance their productivity and operational efficiency.