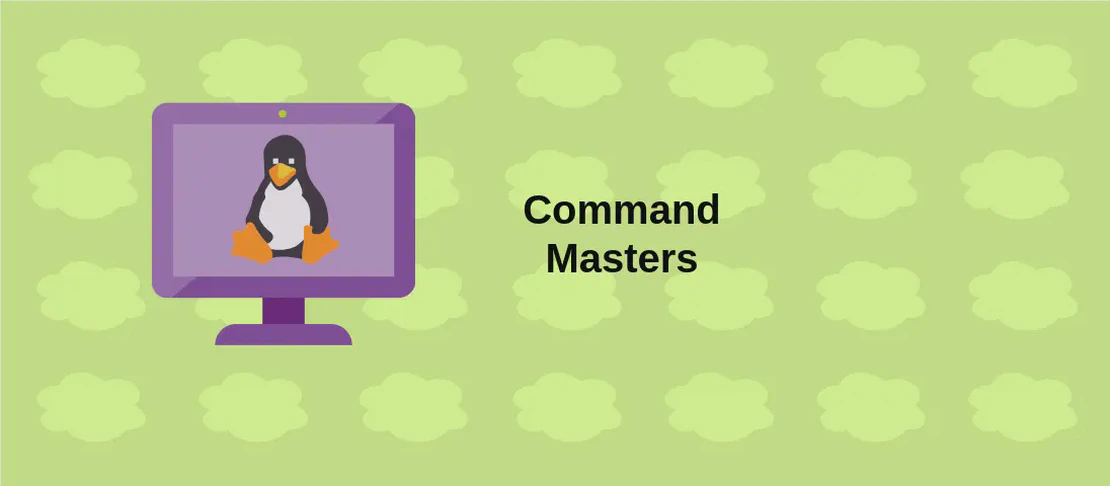
How to Use the Command 'ant' (with Examples)
Apache Ant is a powerful build automation tool primarily used for Java projects. It allows developers to automate the process of building, testing, and deploying Java applications. With Ant, users can streamline their build processes through the use of XML-based configuration files, making project management simpler and more efficient.
Use case 1: Building a Project with Default Build File build.xml
Code:
ant
Motivation:
The default usage of the ant
command is to execute a build process using the build.xml
file located in the current directory. This is the most common scenario when the build file follows the standard naming convention. It simplifies the execution by requiring users to simply run the ant
command without specifying additional parameters, assuming the build file contains all necessary configurations for the default target.
Explanation:
ant
: This invokes the Ant tool to start the build process. By default, Ant looks for a file namedbuild.xml
in the current directory and executes the build targets defined in it.
Example Output:
Buildfile: /path/to/project/build.xml
init:
[echo] Initializing the build process...
compile:
[javac] Compiling source code files...
jar:
[jar] Building the output JAR...
BUILD SUCCESSFUL
Total time: 3 seconds
Use case 2: Building a Project Using a Different Build File
Code:
ant -f buildfile.xml
Motivation:
Projects often have multiple build files for different purposes or environments (e.g., testing, production). The -f
flag allows users to specify an alternative build file, providing flexibility in choosing which configurations to apply. This is particularly useful when working in environments with specialized requirements or settings captured in distinct build files.
Explanation:
-f buildfile.xml
: The-f
option tells Ant to use the specified build file instead of the defaultbuild.xml
. This way, users can tailor the build process to unique project needs without altering the primary build file.
Example Output:
Buildfile: /path/to/project/buildfile.xml
pre-process:
[echo] Preparing environment...
compile:
[javac] Compiling with special classpath...
package:
[jar] Assembling module components...
BUILD SUCCESSFUL
Total time: 2 seconds
Use case 3: Printing Information on Possible Targets
Code:
ant -p
Motivation:
The -p
option helps users quickly ascertain what targets are available in a build file, along with a description of each. This utility is fundamental for developers new to a project, providing insights into the build process without having to manually inspect the build file. It can be especially handy when a build file comprises numerous targets with complex interdependencies.
Explanation:
-p
: This argument instructs Ant to display a list of all targets defined in the build file, along with their respective descriptions, offering a comprehensive overview of the build options available.
Example Output:
Buildfile: /path/to/project/build.xml
Main targets:
build - Compile and assemble the project
clean - Remove all build artifacts
deploy - Deploy artifacts to the production server
test - Run unit tests on the compiled classes
Default target: build
Use case 4: Printing Debugging Information
Code:
ant -d
Motivation:
Debugging builds is crucial when there are errors or unexpected behaviors in the build process. The -d
flag generates comprehensive debugging information, facilitating developers in tracking the precise sequence of events and operations leading to an issue. It’s indispensable for troubleshooting and ensuring that the build process is executing as intended.
Explanation:
-d
: This flag activates debug mode, allowing Ant to produce detailed output about each step of the build process. This level of detail is crucial when diagnosing and resolving issues.
Example Output:
Buildfile: /path/to/project/build.xml
Detected Java version: 1.8 in: /usr/lib/jvm/java-8-openjdk-amd64/jre
Detected OS: Linux
init:
[property] Loading project properties...
[taskdef] Defining custom tasks...
compile:
[mkdir] Creating directory /path/to/project/build
[javac] Compiling 5 source files to /path/to/project/build
BUILD SUCCESSFUL
Total time: 1 second
Use case 5: Executing All Non-Dependent Targets
Code:
ant -k
Motivation:
In complex projects, certain targets may fail due to unresolved dependencies or errors in preceding tasks. The -k
option is handy as it allows the build process to continue with executing independent targets even when some targets fail. This resilience is advantageous in scenarios where partial builds are acceptable, or when developers are continuously integrating code into shared repositories.
Explanation:
-k
: This flag indicates that Ant should proceed with other non-dependent targets even if some targets encounter errors. This is crucial in environments where isolated tasks can continue independently without the success of all prior tasks.
Example Output:
Buildfile: /path/to/project/build.xml
init:
[echo] Initializing build...
errorTarget:
[fail] Sample failure for demonstration
compile:
[javac] Compiling sources...
BUILD FAILED
/path/to/project/build.xml:34: Sample failure for demonstration
After resolving the above:
compile:
[javac] Continuing to compile other sources...
BUILD SUCCESSFUL
Total time: 4 seconds
Conclusion
Apache Ant provides a robust and flexible system for managing Java build processes. By understanding and utilizing the various options such as -f
, -p
, -d
, and -k
, developers can tailor their build experiences to meet the specific needs of their projects, enhancing both productivity and project organization. Whether dealing with large, complex builds or simple projects, Ant’s versatility ensures a streamlined workflow in the Java development ecosystem.