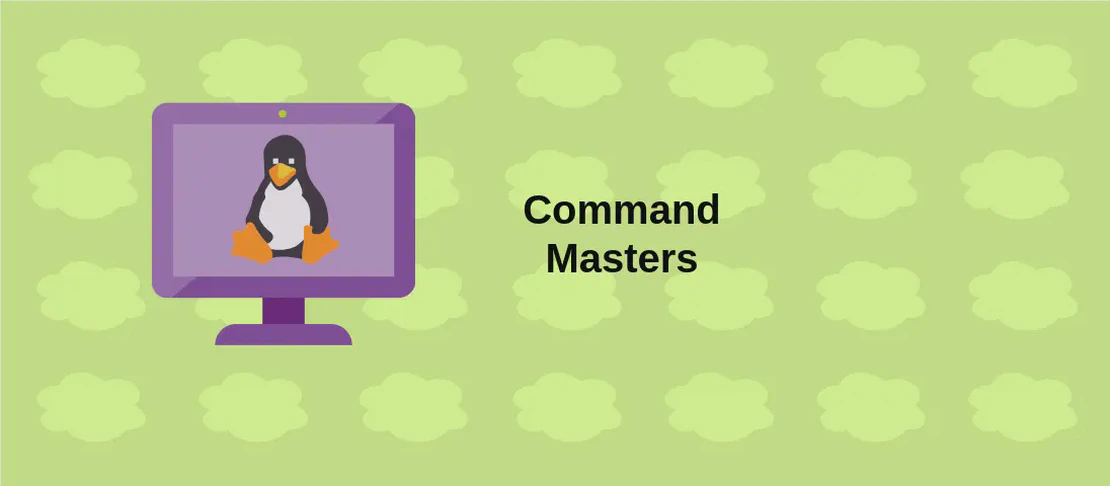
How to use the command 'arduino' (with examples)
The arduino
command is used to interact with the Arduino Studio, which is an Integrated Development Environment (IDE) for the Arduino platform. This command allows you to build, upload sketches (Arduino programs) to Arduino boards, set preferences, install libraries, and perform various other tasks related to Arduino development.
Use case 1: Build a sketch
Code:
arduino --verify path/to/file.ino
Motivation: This use case is helpful when you want to compile your Arduino sketch without uploading it to a board. It allows you to check for any compilation errors before proceeding to upload and run the sketch on a physical Arduino board.
Explanation:
--verify
: This flag tells thearduino
command to only compile the sketch without uploading it.path/to/file.ino
: The path to the Arduino sketch file that you want to compile.
Example output:
Build options changed, rebuilding all
Sketch uses 1014 bytes (3%) of program storage space. Maximum is 32256 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
Use case 2: Build and upload a sketch
Code:
arduino --upload path/to/file.ino
Motivation: This use case is useful when you want to compile and upload an Arduino sketch to a connected Arduino board. It allows you to quickly test your code on a physical device.
Explanation:
--upload
: This flag instructs thearduino
command to compile the sketch and upload it to the connected Arduino board.path/to/file.ino
: The path to the Arduino sketch file that you want to compile and upload.
Example output:
Sketch uses 1014 bytes (3%) of program storage space. Maximum is 32256 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
Use case 3: Build and upload a sketch to an Arduino Nano with an Atmega328p CPU
Code:
arduino --board arduino:avr:nano:cpu=atmega328p --port /dev/ttyACM0 --upload path/to/file.ino
Motivation: This use case is helpful when you have multiple Arduino boards connected to your computer, and you want to specify the specific board and port to upload the sketch to. In this example, we are targeting an Arduino Nano with an Atmega328p CPU connected on the /dev/ttyACM0
port.
Explanation:
--board arduino:avr:nano:cpu=atmega328p
: This argument sets the board type to “arduino:avr:nano” and the CPU to “atmega328p”.--port /dev/ttyACM0
: This argument specifies the port to which the Arduino board is connected.--upload
: This flag tells thearduino
command to both compile and upload the sketch.
Example output:
Sketch uses 1014 bytes (3%) of program storage space. Maximum is 32256 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
Use case 4: Set the preference name
to a given value
Code:
arduino --pref name=value
Motivation: The use case allows you to customize the Arduino IDE’s behavior by setting preferences. This is useful when you want to change specific settings according to your needs.
Explanation:
--pref name=value
: This argument sets the preferencename
to the specifiedvalue
.
Example output: No output is displayed when setting a preference.
Use case 5: Build a sketch and put the build results in the build directory
Code:
arduino --pref build.path=path/to/build_directory --verify path/to/file.ino
Motivation: This use case is helpful when you want to store the build results (compiled files) in a specific directory. It allows you to separate the build artifacts from your source code.
Explanation:
--pref build.path=path/to/build_directory
: This argument sets the build directory path to the specified location.--verify
: This flag instructs thearduino
command to only compile the sketch without uploading it.
Example output:
Build options changed, rebuilding all
Sketch uses 1014 bytes (3%) of program storage space. Maximum is 32256 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
Use case 6: Save any (changed) preferences to preferences.txt
Code:
arduino --save-prefs
Motivation: This use case is useful when you want to save any changes made to the Arduino IDE preferences. It ensures that your preferences are persisted and applied every time you use the arduino
command.
Explanation: No additional arguments are required. The --save-prefs
flag instructs the arduino
command to save any changes made to preferences.
Example output: No output is displayed when saving preferences.
Use case 7: Install the latest SAM board
Code:
arduino --install-boards "arduino:sam"
Motivation: This use case is helpful when you want to install additional board packages for Arduino. In this example, we are installing the latest SAM (Smart Atmel Microcontroller) board.
Explanation:
--install-boards
: This flag tells thearduino
command to install additional board packages."arduino:sam"
: The board identifier for the SAM board package.
Example output: Progress and installation information will be displayed during the installation process.
Use case 8: Install Bridge and Servo libraries
Code:
arduino --install-library "Bridge:1.0.0,Servo:1.2.0"
Motivation: This use case is useful when you want to install additional libraries for Arduino. In this example, we are installing the Bridge and Servo libraries along with their specified versions.
Explanation:
--install-library
: This flag instructs thearduino
command to install libraries."Bridge:1.0.0,Servo:1.2.0"
: The library names and versions to install, separated by commas.
Example output: Progress and installation information will be displayed during the installation process.
Conclusion:
The arduino
command offers a wide range of functionalities to aid in Arduino development. Whether you need to build, upload sketches, set preferences, or install libraries, this command provides a convenient way to interact with the Arduino IDE from the command line.