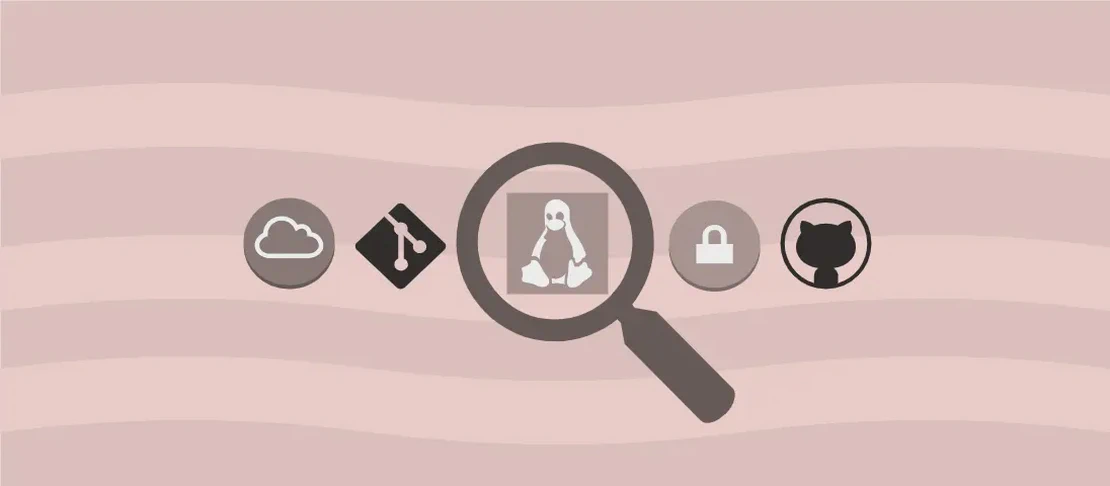
Utilizing the Portable GNU Assembler (as) (with examples)
- Linux
- December 17, 2024
The Portable GNU Assembler, commonly known as as
, serves as an essential component within the GNU toolchain. Its primary function is to transform the assembly language source code, typically generated by the GNU Compiler Collection (GCC), into machine code object files ready for linking. Designed to be portable and versatile, as
offers various options that cater to different assembly needs. Below, we’ll explore some practical use cases of this assembler with detailed examples.
Use case 1: Assemble a file, writing the output to a.out
Code:
as path/to/file.s
Motivation:
The most basic use of the as
command is to assemble an assembly language source file and produce an output file, typically named a.out
. This command is particularly useful for developers who have manually written assembly code and want to convert it into an object file for further processing or execution. This straightforward approach is beneficial in educational contexts where learning the fundamentals of assembly language is necessary.
Explanation:
as
: This invokes the assembler program.path/to/file.s
: This specifies the path to the assembly source file that you wish to compile. The.s
extension is standard for assembly language source files.
Example Output:
Upon successful execution, the assembler will generate an object file named a.out
in the current directory. Assuming file.s
contains a simple program, the command will produce no visible console output unless there are errors during assembly.
Use case 2: Assemble the output to a given file
Code:
as path/to/file.s -o path/to/output_file.o
Motivation:
Sometimes, the default output file name a.out
might not be desirable, especially when working with multiple object files within the same directory. In such cases, specifying an output file name with the -o
option provides clarity and organization. This approach facilitates better control over the build process in larger projects where multiple files are involved.
Explanation:
as
: This is the command to invoke the assembler.path/to/file.s
: Indicates the source assembly file to be assembled.-o
: This option allows you to define a custom name and location for the output object file.path/to/output_file.o
: This specifies the desired path and filename for the output object file.
Example Output:
The assembler will compile file.s
into a machine-understandable object file stored at the specified path, output_file.o
. The process occurs silently, producing no console output unless an error is encountered.
Use case 3: Generate output faster by skipping whitespace and comment preprocessing
Code:
as -f path/to/file.s
Motivation:
In situations where build times are crucial or where the assembler’s input is generated by a trusted source (such as a compiler that ensures no harmful content exists), the -f
option can be used to speed up assembly. This option skips the preprocessing of whitespace and comments, reducing the overall processing time and improving efficiency without sacrificing output integrity from reliable sources.
Explanation:
as
: Means to invoke the assembler operation.-f
: Stands for “fast,” directing the assembler to bypass the preprocessing steps for whitespace and comments. It’s a performance-oriented feature.path/to/file.s
: Refers to the source file to be assembled.
Example Output:
This use case handles source files quickly, producing an optimized assemble time. It outputs an a.out
file with no terminal printout unless errors occur.
Use case 4: Include a given path to the list of directories to search for files specified in .include
directives
Code:
as -I path/to/directory path/to/file.s
Motivation:
When working with assembly code that includes multiple files or library components, managing include paths becomes crucial. The -I
option allows specifying additional directories where the assembler should look for files referenced in .include
directives. This capability is extremely useful in managing complex projects with a modular structure.
Explanation:
as
: Initializes the assembler utility.-I path/to/directory
: Informsas
to add this directory to its search path for.include
directives within the source file.path/to/file.s
: The assembly source file to be compiled that might contain.include
directives referencing additional files.
Example Output:
Upon successful assembly, the assembler checks the specified directory for any included files within file.s
, ensures all dependencies are correctly located, and compiles without error, creating an a.out
file.
Conclusion:
The Portable GNU Assembler as
is an indispensable tool for assembling assembly code efficiently. Whether you are generating object files from source code, improving processing speed, or managing multi-file dependencies, understanding and effectively utilizing as
commands can significantly enhance the software development process. By mastering these use cases, developers can better leverage the powerful capabilities of the GNU assembler in their projects.