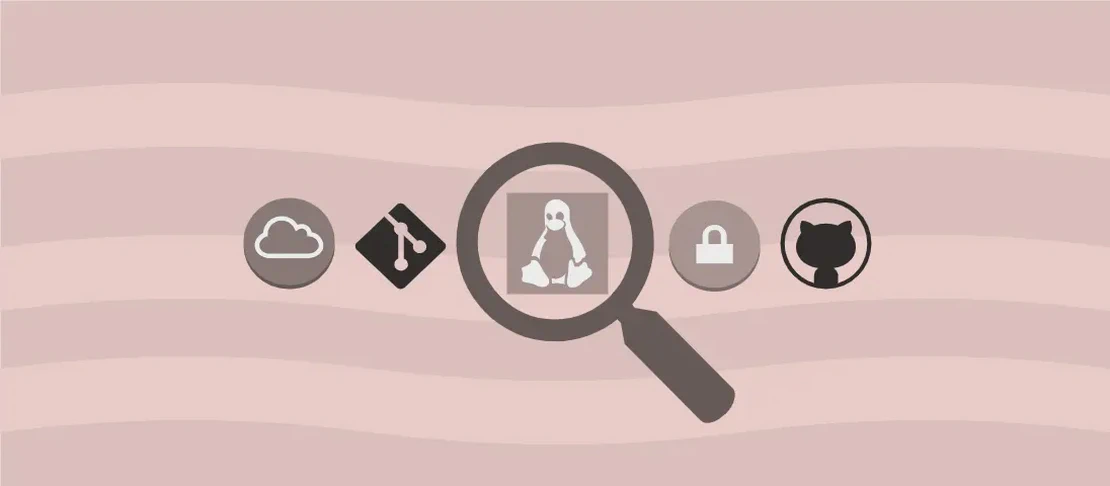
How to Use the Command 'as' (with examples)
- Osx
- December 17, 2024
The ‘as’ command refers to the Portable GNU assembler, which plays a crucial role in the process of compiling programs. The primary function of this command is to take human-readable assembly language code and assemble it into machine code that can be executed by a computer. This command is generally used in conjunction with the output generated by the gcc
compiler, ultimately linking everything together using the ld
linker. In essence, ‘as’ serves as an intermediary step in converting high-level code (such as C or C++) into a directly executable format. Below are a few common use cases of the ‘as’ command, illustrating different ways of executing it to achieve your programming objectives.
Assemble a File, Writing the Output to a.out
Code:
as path/to/file.s
Motivation:
The simplicity of using the as
command in its basic form allows users to quickly assemble an assembly file into machine code. Developers often need to test or debug assembly routines independently of a larger project. By default, when running the ‘as’ command with a specified assembly file (.s
), the resulting machine code is output to a file named a.out
. This default behavior makes it straightforward to generate executable output for testing purposes without specifying additional parameters.
Explanation:
as
: Invokes the GNU assembler.path/to/file.s
: Specifies the path to the source assembly file that needs to be assembled. This file contains the human-readable assembly instructions that the developer wishes to convert to machine code.
Example Output:
After running this command, a new file named a.out
is created in the current directory. This file contains the machine code output of the assembled program and can be executed or further processed as needed.
Assemble the Output to a Given File
Code:
as path/to/file.s -o path/to/output_file.o
Motivation:
Sometimes developers require more control over where and how the assembled output is stored, rather than relying on the default a.out
file format. By explicitly specifying an output filename, developers can more easily manage their build artifacts, potentially organizing them into structured directories or preparing them for integration with other build steps. This ability to redirect output is especially useful in larger projects involving multiple assembly files or when adherence to naming conventions is required for integration with other tools or scripts.
Explanation:
as
: Calls the GNU assembler command.path/to/file.s
: Indicates the path to the source file that contains assembly instructions.-o path/to/output_file.o
: The-o
flag allows you to specify an alternative output file.path/to/output_file.o
is where the assembled machine code will be written. Use this option when you need to maintain organized file structures or specific filenames.
Example Output:
Executing this command results in the creation of a file named output_file.o
at the specified path. This file contains the machine code which can be linked or executed as part of a larger application.
Generate Output Faster by Skipping Whitespace and Comment Preprocessing
Code:
as -f path/to/file.s
Motivation:
In scenarios where the assembler’s speed is of critical importance, and the assembly code is generated from a trusted source, developers might opt to bypass certain preprocessing steps. By disabling whitespace and comment processing using the -f
option, the assembler can operate more rapidly. This optimization is typically only advisable in controlled environments where there is confidence in the source code’s validity and security, such as when the code is automatically generated by a trusted compiler as part of a high-performance build process.
Explanation:
as
: Executes the GNU assembler.-f
: This option tells the assembler to ignore whitespace and comments in the source file, which speeds up the assembly process. It should be used cautiously since omitting these may make debugging more challenging.path/to/file.s
: Refers to the path of the source assembly file to be assembled.
Example Output:
After running the command, a faster produced a.out
is created (or other specified file using -o
if used in conjunction). The speed enhancement comes from reduced parsing overhead, particularly beneficial in large-scale builds.
Include a Given Path for .include
Directives
Code:
as -I path/to/directory path/to/file.s
Motivation:
Assembly language files sometimes need to include code from other files to manage dependencies or to modularly organize code in a manner akin to include statements in C or C++. This feature is facilitated by .include
directives within the assembly source code. By using the -I
option with ‘as’, developers can direct the assembler to look in additional directories for these included files, thereby making the build process more flexible and organized.
Explanation:
as
: Initiates the GNU assembler.-I path/to/directory
: This option augments the list of directories where the assembler will look for files specified by.include
directives. This is critical when assembly source files are spread across various locations.path/to/file.s
: The path of the primary assembly source file being assembled.
Example Output:
When executed, if the source file contains any .include
directives, the assembler successfully locates the files in the specified directory and incorporates them into the output. A resulting a.out
or another specified object file (again using -o
) is produced, combining the various assembly code segments.
Conclusion:
The ‘as’ command is a foundational tool in the process of software development, particularly when working with low-level assembly language. Its flexibility and versatility, as demonstrated by the examples above, provide developers with tools to manage and streamline the assembly of machine code from human-readable instructions effectively. Whether simply assembling a single file, optimizing performance, managing complex file dependencies, or organizing output, the ‘as’ command is instrumental in bridging the gap between assembly language and executable machine code.