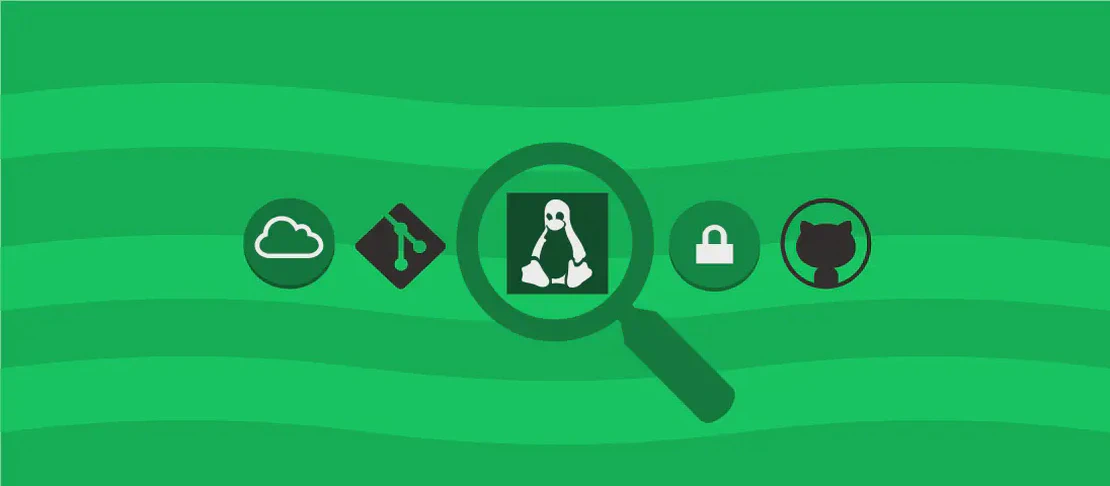
A Guide to Using the 'atoum' Command for Unit Testing in PHP (with examples)
Atoum is a simple, modern, and intuitive unit testing framework designed for PHP developers. It provides a clean and efficient way to create and automate tests for PHP applications, ensuring code reliability and quality. Its command-line usage offers several options for initializing configurations, running tests, and more. This guide will walk you through various use cases to help you leverage the capabilities of atoum effectively.
Use case 1: Initialize a configuration file
Code:
atoum --init
Motivation:
Initializing a configuration file is usually the first step in setting up atoum for a new project. It’s essential because it sets the groundwork for customizing your testing environment, specifying settings and preferences that suit your testing strategy.
Explanation:
atoum
: This is the command to invoke the atoum test framework.--init
: This option generates a default configuration file (.atoum.php
) in your project’s root directory. This file can then be modified to align with your project’s specific testing needs.
Example output:
Configuration file `.atoum.php` has been created.
Use case 2: Run all tests
Code:
atoum
Motivation:
Running all tests in your codebase is crucial for verifying that every part of your application is functioning as expected. It’s a common practice in continuous integration pipelines to ensure no regression issues have been introduced with new changes.
Explanation:
atoum
: Executing the command without additional options runs all the unit tests across the codebase that have been set up according to the configuration file.
Example output:
> Running tests...
> Tests: 10, Assertions: 50, Failures: 0, Errors: 0, Success: 100%.
Use case 3: Run tests using the specified configuration file
Code:
atoum -c path/to/file
Motivation:
Different projects may require different configurations due to varying environments or dependencies. Running tests with a specific configuration file allows developers to easily manage and switch between these setups.
Explanation:
-c
: This flags the use of a custom configuration file.path/to/file
: This is the relative or absolute path to the configuration file you wish to use for the test run.
Example output:
Using configuration from path/to/custom.atoum.php
> Running tests...
> Tests: 12, Assertions: 60, Failures: 0, Errors: 0, Success: 100%.
Use case 4: Run a specific test file
Code:
atoum -f path/to/file
Motivation:
Running a specific test file is useful for targeted testing, especially during development. It saves time and resources by focusing only on the areas of the code that have changed or are currently the subject of debugging.
Explanation:
-f
: This option specifies that a single test file should be run.path/to/file
: This should be the path to the test file you want to execute.
Example output:
Running test file: path/to/MyComponentTest.php
> Tests: 1, Assertions: 5, Failures: 0, Errors: 0, Success: 100%.
Use case 5: Run a specific directory of tests
Code:
atoum -d path/to/directory
Motivation:
Testing all files within a particular directory allows for efficient organization of tests and quick verification of modules, components, or features contained within that folder. This segmentation is particularly helpful in large projects.
Explanation:
-d
: This tells atoum to execute tests in all files within the specified directory.path/to/directory
: This denotes the path to the directory containing the test files you wish to run.
Example output:
Running tests in directory: path/to/moduleTests/
> Tests: 4, Assertions: 20, Failures: 0, Errors: 0, Success: 100%.
Use case 6: Run all tests under a specific namespace
Code:
atoum -ns namespace
Motivation:
Namespaces help organize code and tests according to functionality or logical groupings. Running tests under a specific namespace helps ensure no related pieces of code are affected by changes, providing a more structured testing approach.
Explanation:
-ns
: This option restricts the test suite to only those within the specified namespace.namespace
: This represents the namespace whose tests you wish to execute.
Example output:
Running tests under namespace: MyApp\Components
> Tests: 6, Assertions: 30, Failures: 0, Errors: 0, Success: 100%.
Use case 7: Run all tests with a specific tag
Code:
atoum -t tag
Motivation:
Using tags to categorize tests can be beneficial for executing tests that have a particular focus, such as performance, database interactions, or third-party API integrations. It’s a flexible method for managing and running subsets of tests based on specific attributes.
Explanation:
-t
: This flags the inclusion of tests marked with a particular tag.tag
: This is the identifier used in your test annotations to group specific tests.
Example output:
Running tests with tag: performance
> Tests: 3, Assertions: 15, Failures: 0, Errors: 0, Success: 100%.
Use case 8: Load a custom bootstrap file before running tests
Code:
atoum --bootstrap-file path/to/file
Motivation:
Bootstrap files are scripts that run before your test suite initiates. They are used to set up the test environment, load necessary dependencies, or configure global settings. Using a custom bootstrap file ensures your tests run in the correct context.
Explanation:
--bootstrap-file
: This option specifies a custom script to execute before running tests.path/to/file
: This indicates the path to the bootstrap file that should be loaded prior to your tests.
Example output:
Loading bootstrap file: path/to/init.php
Executing tests...
> Tests: 8, Assertions: 40, Failures: 0, Errors: 0, Success: 100%.
Conclusion:
Atoum is a powerful tool for PHP developers looking to establish a robust unit testing framework. By understanding and utilizing its command-line options, you can tailor your testing strategy to meet the unique demands of your projects, ultimately leading to more reliable and maintainable code.