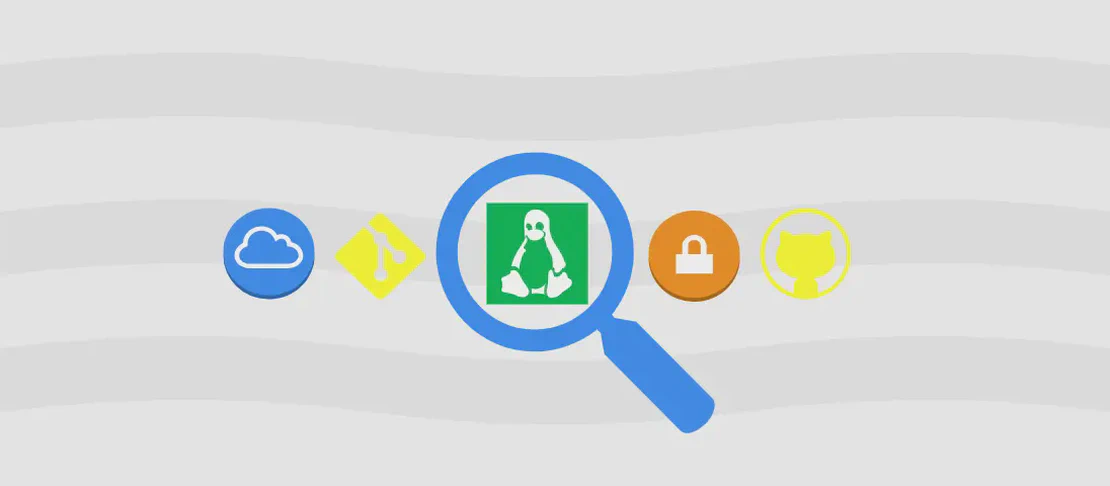
How to Use the Command 'autoflake' (with examples)
Autoflake is a utility that simplifies the process of cleaning up and optimizing Python code by removing unused imports and variables. It helps maintain clean and readable code, ensuring that your scripts run more efficiently without unnecessary clutter. This tool is particularly useful for large projects with multiple files that might contain redundant code segments accumulated over time. It is an essential tool for developers aiming to enhance code quality and maintainability.
Use Case 1: Remove Unused Variables from a Single File and Display the Diff
Code:
autoflake --remove-unused-variables path/to/file.py
Motivation:
In the process of writing or evolving Python scripts, developers often leave behind variables that are no longer in use. These unused variables can lead to confusion and potential misinterpretation of the code’s purpose. By using this command, developers can easily identify and remove such variables from a single file, thereby improving the code’s readability and maintainability.
Explanation:
autoflake
: This command initiates the autoflake tool to perform code cleaning.--remove-unused-variables
: This option specifically targets and removes variables that are defined in the code but never used.path/to/file.py
: This argument specifies the path to the file from which unused variables should be removed.
Example Output:
- unused_var = 5
print("Hello World")
Use Case 2: Remove Unused Imports from Multiple Files and Display the Diffs
Code:
autoflake --remove-all-unused-imports path/to/file1.py path/to/file2.py ...
Motivation:
As projects grow, they often accumulate import statements that are no longer needed because the corresponding modules or functions are not used in the code anymore. This can increase the clutter and reduce the efficiency of the code. Using autoflake to clean up multiple files at once helps keep the import sections clean and organized, which in turn enhances code readability and potentially reduces loading times.
Explanation:
--remove-all-unused-imports
: This argument tells autoflake to remove all unused import statements from the specified files.path/to/file1.py path/to/file2.py ...
: These arguments specify the paths to the files in which unused imports should be removed.
Example Output:
- import os
- import sys
def main():
print("Hello World")
Use Case 3: Remove Unused Variables from a File, Overwriting the File
Code:
autoflake --remove-unused-variables --in-place path/to/file.py
Motivation:
When it is necessary to ensure the source file is immediately updated without generating a separate output or diff, the --in-place
option becomes handy. This might be useful in automated scripts or CI/CD pipelines where the updated file should be directly usable in subsequent steps.
Explanation:
--in-place
: This option overwrites the original file with the cleaned-up version, saving the changes directly to it.- Combining
--remove-unused-variables
with--in-place
ensures that any unnecessary variables are removed, and the original file is replaced with the improved version.
Example Output:
The file path/to/file.py
is updated directly with the unused variables removed.
Use Case 4: Remove Unused Variables Recursively from All Files in a Directory, Overwriting Each File
Code:
autoflake --remove-unused-variables --in-place --recursive path/to/directory
Motivation:
In large projects with numerous files organized within directories, ensuring that all scripts are free of unused variables is a time-consuming task if handled manually. By using the --recursive
flag, developers can efficiently clean up all Python files within a directory and its subdirectories, automating the process of optimizing the entire codebase.
Explanation:
--recursive
: This flag allows autoflake to traverse through all files within the specified directory and its subdirectories.- When
--recursive
is used with--remove-unused-variables
and--in-place
, it ensures that each Python file in the directory hierarchy is checked and updated for unused variables.
Example Output:
All Python files in path/to/directory
are cleaned of unused variables with the changes written directly to each file.
Conclusion:
Autoflake proves to be an invaluable command-line utility in optimizing and maintaining Python code by removing unnecessary imports and variables. Whether working on a single file or a large project with multiple files, autoflake provides flexibility and efficiency, offering different modes and options to suit various development needs. By incorporating autoflake into regular coding practices, developers can ensure cleaner, more efficient, and more readable codebases.