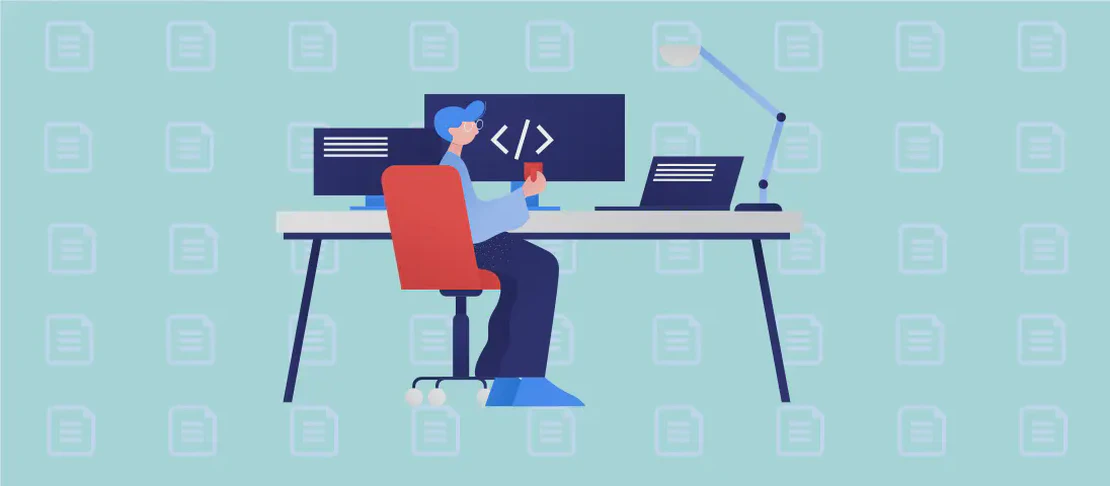
How to Use the Command 'autopep8' (with examples)
autopep8
is a utility for automatically formatting Python code to conform to the PEP 8 style guide. It helps ensure that Python code is neatly formatted, improving readability and maintaining consistency across projects, which can be particularly helpful in collaborative environments or for developers who maintain various projects. The tool handles a variety of formatting concerns, such as line length, whitespace, and indentation, allowing developers to focus on functionality rather than style compliance.
Use case 1: Format a file to stdout
, with a custom maximum line length
Code:
autopep8 path/to/file.py --max-line-length 100
Motivation:
In many development projects, adhering to the PEP 8 style guide is crucial for consistency. However, developers might have specific stylistic needs, such as a custom maximum line length. This use case allows developers to quickly preview how their Python file would look with a specific line length constraint before applying these changes. Displaying the formatted code to stdout
instead of modifying the file directly gives developers a chance to review the changes and ensure that nothing unintended happens to the code structure or logic.
Explanation:
autopep8
: This is the command that initiates the auto-formatting according to PEP 8.path/to/file.py
: Specifies the path to the Python file that needs formatting.--max-line-length 100
: This argument sets a custom maximum line length to 100 characters per line, modifying the default PEP 8 rule of 79 characters. It is particularly useful when working with larger screens or when longer lines are acceptable within the project guidelines.
Example Output:
Suppose the original file contains:
def example_function(): return "This is an example of a function that goes beyond the default line length in PEP 8"
The output would be:
def example_function():
return "This is an example of a function that goes beyond the default line length in PEP 8"
Use case 2: Format a file, displaying a diff of the changes
Code:
autopep8 --diff path/to/file
Motivation:
Before committing to changes, developers often need to see what modifications will be applied to their code. By using the --diff
flag, developers can visualize the precise differences between the original and formatted code. This aids in understanding how autopep8
will alter the file, ensuring no semantic or logical errors are introduced through formatting. It also helps in educational environments where understanding the reasoning behind style changes is beneficial.
Explanation:
autopep8
: This command triggers PEP 8 formatting on the specified file.--diff
: Instead of overwriting the file, this option shows a unified diff of the changes that would be made. This is similar to running a typical diff command, which displays the differences between two files.path/to/file
: The path to the file to be checked and compared.
Example Output:
Assume the code in path/to/file
looks like this:
def foo ( arg ):
print( "misformatted" )
The diff output would appear like:
--- original/path/to/file
+++ fixed/path/to/file.py
@@ -1,2 +1,2 @@
-def foo ( arg ):
- print( "misformatted" )
+def foo(arg):
+ print("misformatted")
Use case 3: Format a file in-place and save the changes
Code:
autopep8 --in-place path/to/file.py
Motivation:
This use case is ideal when a developer is confident about applying the PEP 8 formatting changes directly to their files. The --in-place
flag allows autopep8
to modify the Python file directly, eliminating the need for manual oversight after changes are confirmed to be standard-compliant. This is particularly useful in larger codebases or when making bulk changes, saving a significant amount of time that would otherwise be spent manually editing files.
Explanation:
autopep8
: Initializes the PEP 8-compliant formatting.--in-place
: Directs the tool to overwrite the original file with the PEP 8-formatted version.path/to/file.py
: The target file for in-place formatting.
Example Output:
Given an input file:
def bar( ) :
return 42
After running the command, the file content will be:
def bar():
return 42
Use case 4: Recursively format all files in a directory in-place and save changes
Code:
autopep8 --in-place --recursive path/to/directory
Motivation:
In project environments, there are often numerous Python files spread across multiple directories. Instead of manually formatting each file, this command allows for bulk formatting, which recursively processes every Python file in the specified directory. This is a time-efficient method to enforce the PEP 8 style guide across entire projects or when onboarding new codebases into a standardized format.
Explanation:
autopep8
: Starts the formatting process for Python files.--in-place
: Directs the command to apply changes directly to the files.--recursive
: Tellsautopep8
to process all Python files within the specified directory and its subdirectories.path/to/directory
: The root directory where recursive formatting should begin.
Example Output:
If a directory contains several misformatted Python files, running this command will update each of them to comply with PEP 8, ensuring consistency and readability without manually checking each file.
Conclusion:
The autopep8
command is an invaluable tool for Python developers aiming to maintain consistency and readability according to the PEP 8 style guide. Its versatility, from previewing changes to bulk formatting entire directories, makes it an essential part of a developer’s toolkit. By automating the mundane task of code formatting, autopep8
allows developers to focus on more critical aspects of software development, enhancing productivity and code quality.