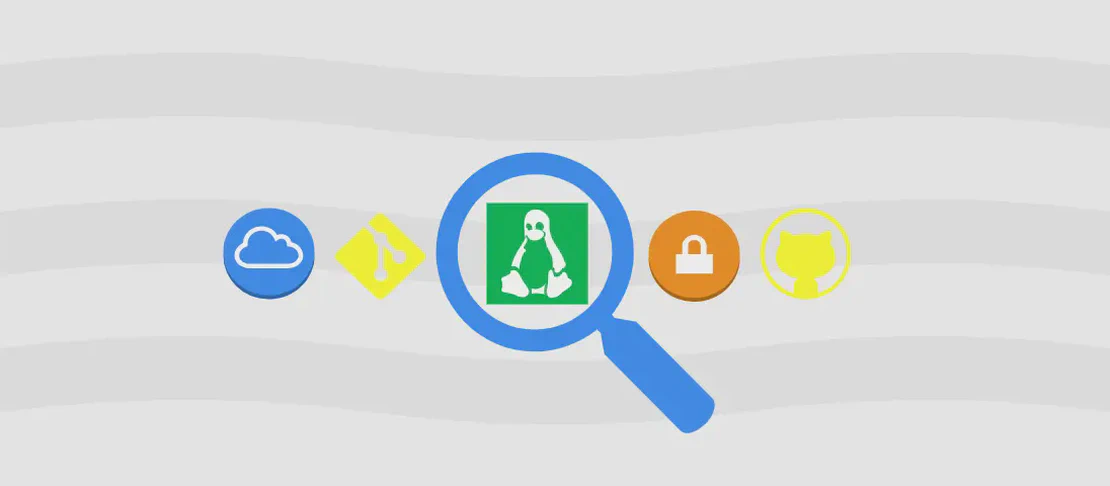
How to Use the Command 'aws acm' (with examples)
The AWS Certificate Manager (ACM) is a service that simplifies the process of provisioning, managing, and deploying SSL/TLS certificates for your AWS applications. These certificates can be used to secure network communications and establish a secure identity by encrypting traffic between the client and the server. The AWS CLI provides a comprehensive set of commands to manage these certificates from the command line efficiently. Here, we will explore various use cases for the ‘aws acm’ command with detailed examples.
Use case 1: Import a Certificate
Code:
aws acm import-certificate --certificate-arn certificate_arn --certificate certificate --private-key private_key --certificate-chain certificate_chain
Motivation:
Importing a certificate is essential when you need to use an existing SSL/TLS certificate with AWS services that are not originally managed by ACM, such as those you’ve purchased from a third-party CA. This ability provides flexibility and control over how certificates are applied within your AWS architecture, enhancing security and compliance.
Explanation:
--certificate-arn certificate_arn
: This argument specifies the Amazon Resource Name (ARN) of the certificate to import, acting as a unique identifier.--certificate certificate
: The actual SSL/TLS certificate file that you want to import, in PEM format.--private-key private_key
: The private key that corresponds to the public key contained in the certificate, also in PEM format.--certificate-chain certificate_chain
: The chain of one or more certificates that establish the trust chain, in PEM format.
Example Output:
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id"
}
This output indicates that the certificate has been successfully imported, and provides the ARN for future reference and actions.
Use case 2: List Certificates
Code:
aws acm list-certificates
Motivation:
Listing certificates allows administrators to view all the certificates currently managed by ACM in a given AWS account. It is particularly useful when assessing the health, expiration dates, and overall security posture of the infrastructure.
Explanation:
This command does not require any additional arguments or options as it retrieves all certificates on record for the account. It queries ACM to provide a list and quick insights into the managed certificates.
Example Output:
{
"CertificateSummaryList": [
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id",
"DomainName": "example.com"
},
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id2",
"DomainName": "example.org"
}
]
}
This output provides a summary of the certificates along with their associated domain names.
Use case 3: Describe a Certificate
Code:
aws acm describe-certificate --certificate-arn certificate_arn
Motivation:
Describing a certificate provides detailed information about a specific SSL/TLS certificate managed within ACM. This information can be critical for debugging, renewal processes, and verifying if it meets the desired security standards and configurations.
Explanation:
--certificate-arn certificate_arn
: The specific ARN of the certificate that you want to describe. It identifies which certificate’s details need to be retrieved.
Example Output:
{
"Certificate": {
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id",
"DomainName": "example.com",
"SubjectAlternativeNames": ["www.example.com"],
"DomainValidationOptions": [
{
"DomainName": "example.com",
"ValidationMethod": "DNS"
}
],
"IssuedAt": "2023-04-01T00:00:00Z",
"Status": "ISSUED"
}
}
This output provides comprehensive information about the certificate, including its status, domain names, and validation methods.
Use case 4: Request a Certificate
Code:
aws acm request-certificate --domain-name domain_name --validation-method validation_method
Motivation:
Requesting a new certificate is a fundamental operation when setting up a new domain or service that requires HTTPS via SSL/TLS encryption. This command initiates the process to create a public or private certificate to secure online communications.
Explanation:
--domain-name domain_name
: This is the domain name you want to secure with the certificate.--validation-method validation_method
: The method used to validate the domain, typically DNS or EMAIL, confirming ownership before certificate issuance.
Example Output:
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id"
}
The output indicates a successful request for a new certificate and provides the ARN for management and follow-up actions.
Use case 5: Delete a Certificate
Code:
aws acm delete-certificate --certificate-arn certificate_arn
Motivation:
Deleting certificates is necessary when they are no longer needed, expired, or compromised. This reduces clutter, ensures security, and lowers the risk of using obsolete certificates.
Explanation:
--certificate-arn certificate_arn
: Identifies the certificate to be deleted using its unique ARN.
Example Output:
There is no output if the command is successful, which silently confirms that the certificate has been removed from ACM management.
Use case 6: List Certificate Validations
Code:
aws acm list-certificates --certificate-statuses status
Motivation:
Listing certificate validations offers insight into the current statuses of domain validations for certificates. This is crucial for diagnosing validation issues and ensuring certificates are active and operational.
Explanation:
--certificate-statuses status
: Filters the results to list certificates with specific statuses, such as PENDING_VALIDATION, ISSUED, etc.
Example Output:
{
"CertificateSummaryList": [
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id",
"DomainName": "example.com",
"Status": "PENDING_VALIDATION"
}
]
}
This output displays certificates that are pending validation, helping to manage actions that may be needed to complete the process.
Use case 7: Get Certificate Details
Code:
aws acm get-certificate --certificate-arn certificate_arn
Motivation:
Retrieving the certificate details in PEM format is essential during deployments or when integrating with applications and services requiring explicit certificate content for configuration.
Explanation:
--certificate-arn certificate_arn
: Specifies the unique ARN of the certificate whose details you want to retrieve.
Example Output:
{
"Certificate": "-----BEGIN CERTIFICATE----- ... -----END CERTIFICATE-----",
"CertificateChain": "-----BEGIN CERTIFICATE----- ... -----END CERTIFICATE-----"
}
This output provides the actual certificate and its chain, which can be used in server configurations and other applications.
Use case 8: Update Certificate Options
Code:
aws acm update-certificate-options --certificate-arn certificate_arn --options options
Motivation:
Updating certificate options allows administrators to apply changes to an existing certificate’s properties, such as enabling or disabling key management features or fine-tuning security parameters without needing to issue a new certificate.
Explanation:
--certificate-arn certificate_arn
: The ARN of the certificate you want to update.--options options
: Key-value pairs for the options to update, like enabling or disabling specific features.
Example Output:
{
"CertificateArn": "arn:aws:acm:region:account-id:certificate/certificate-id"
}
The output confirms that the certificate options have been updated successfully.
Conclusion:
The AWS Certificate Manager (ACM) command-line interface allows comprehensive management of SSL/TLS certificates. Whether importing, listing, describing, requesting, or deleting certificates, these commands facilitate effective certificate lifecycle management to ensure secure communications for AWS-hosted applications. By familiarizing yourself with these specific use cases, you can streamline certificate management tasks and enhance your organization’s cybersecurity posture.