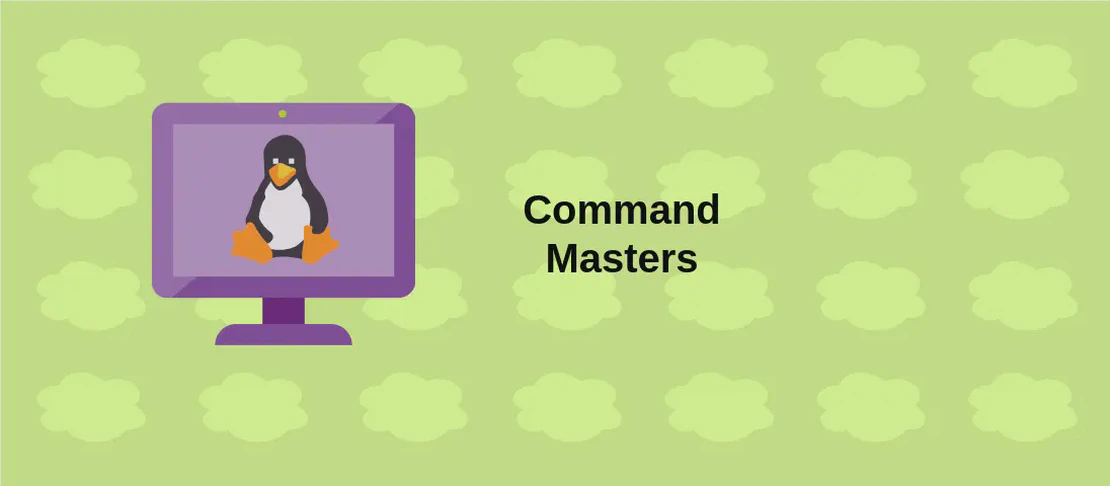
How to use the command 'aws amplify' (with examples)
Amazon Web Services (AWS) Amplify is a development platform designed to simplify the process of building secure and scalable mobile and web applications. With Amplify, developers can rapidly build full-stack applications with seamless integration into AWS services. The AWS CLI (Command Line Interface) provides a comprehensive set of commands that can be used to automate tasks related to AWS Amplify, such as creating and managing applications, backend environments, and more.
Create a new Amplify app (with examples)
Code:
aws amplify create-app --name app_name --description description --repository repo_url --platform platform --environment-variables env_vars --tags tags
Motivation:
Creating a new Amplify app is often one of the first steps for developers looking to take advantage of AWS Amplify’s powerful features. Whether you’re starting a new project or migrating an existing one to AWS, initializing a new Amplify app provides you with all the necessary resources and configurations to begin development immediately.
Explanation:
--name app_name
: Specifies the unique name of your Amplify app. This is essential for identifying and managing your app on the AWS platform.--description description
: Provides information about the app’s purpose or features. This is useful for documentation and team communication.--repository repo_url
: Links your app to a source control repository, such as GitHub, which facilitates version control and collaboration.--platform platform
: Defines the platform(s) the app will support (e.g., Web, iOS, Android). Choosing the right platform ensures compatibility and optimizes your app’s configuration.--environment-variables env_vars
: Sets key-value pairs as environment variables that the app can utilize, which is crucial for managing configurations across different stages and environments.--tags tags
: Attaches metadata to the app for better resource management like billing and access controls by applying custom tags relevant to your organization.
Example Output:
{
"app": {
"appId": "wxyz1234",
"appArn": "arn:aws:amplify:us-east-1:123456789012:apps/wxyz1234",
"name": "app_name",
"defaultDomain": "appname.amplifyapp.com",
...
}
}
Delete an existing Amplify app (with examples)
Code:
aws amplify delete-app --app-id app_id
Motivation:
Deleting an existing Amplify app is a necessary step when a project has reached its end-of-life or when you need to manage costs by eliminating unused applications. It’s a critical task to ensure that resources are not left idly consuming infrastructure space and possibly incurring charges.
Explanation:
--app-id app_id
: Identifies the app to delete from AWS Amplify; this unique identifier is required to safely remove the correct instance and all associated resources.
Example Output:
{
"app": {
"appId": "wxyz1234",
"name": "app_name",
...
}
}
Get details of a specific Amplify app (with examples)
Code:
aws amplify get-app --app-id app_id
Motivation:
Fetching the details of a specific Amplify app is a valuable process when you need to review the configuration and resources associated with your applications. This functionality is crucial for audit logging, troubleshooting, and ensuring environments are set up correctly.
Explanation:
--app-id app_id
: The unique identifier of the Amplify app whose details you want to retrieve; this ensures the command pulls information only for the designated app.
Example Output:
{
"app": {
"appId": "wxyz1234",
"appArn": "arn:aws:amplify:us-east-1:123456789012:apps/wxyz1234",
"name": "app_name",
"description": "description",
...
}
}
List all Amplify apps (with examples)
Code:
aws amplify list-apps
Motivation:
Listing all Amplify apps provides oversight of all the applications currently managed under your AWS account, which is instrumental for understanding the scope of projects your team is handling, managing resources, and budgeting effectively.
Explanation:
This command doesn’t require additional arguments, as it is designed to return a comprehensive list of all Amplify applications within your AWS account, providing visibility into each app’s basic details such as name, ID, and status.
Example Output:
{
"apps": [
{
"appId": "abcd1234",
"name": "AppOne",
...
},
{
"appId": "wxyz5678",
"name": "AppTwo",
...
}
]
}
Update settings of an Amplify app (with examples)
Code:
aws amplify update-app --app-id app_id --name new_name --description new_description --repository new_repo_url --environment-variables new_env_vars --tags new_tags
Motivation:
Updating the settings of an Amplify app is essential for continuous development and environment consistency. Whether changes in the project scope require a new repository or environment variables require alteration for security reasons, keeping app configurations up-to-date ensures smooth and efficient workflow operations.
Explanation:
--app-id app_id
: The identifier of the Amplify app you wish to update.--name new_name
: Updates the existing app’s name to reflect a new project title or modified branding.--description new_description
: Allows the update of descriptive information to provide clarity on the app’s features or changes.--repository new_repo_url
: Changes the link to the source control repository, often needed when the codebase moves repositories.--environment-variables new_env_vars
: Adjusts key-value pairs critical for differentiating environments or deploying updated configurations.--tags new_tags
: Revises or appends new metadata for enhanced organization and management of AWS resources.
Example Output:
{
"app": {
"appId": "wxyz1234",
"name": "new_name",
...
}
}
Add a new backend environment to an Amplify app (with examples)
Code:
aws amplify create-backend-environment --app-id app_id --environment-name env_name --deployment-artifacts artifacts
Motivation:
Adding a new backend environment is crucial for developers who need distinct environments for development, testing, and production. It enables teams to test new features or configurations without affecting the live application, promoting better development workflows and smoother deployments.
Explanation:
--app-id app_id
: Indicates the Amplify app for which the new backend environment is to be created.--environment-name env_name
: Establishes a clear label for the new environment, distinguishing it from existing environments and making it easier to manage different stages of the application lifecycle.--deployment-artifacts artifacts
: Specifies the location of the deployment artifacts necessary for setting up the environment properly, ensuring that backend resources are initialized as per the intended setup.
Example Output:
{
"backendEnvironment": {
"environmentName": "staging",
"stackName": "amplify-appname-staging",
...
}
}
Remove a backend environment from an Amplify app (with examples)
Code:
aws amplify delete-backend-environment --app-id app_id --environment-name env_name
Motivation:
Removing a backend environment is essential when streamlining infrastructure, cleaning up after merges, or discontinuing unused or obsolete environments. This helps in maintaining organized resource allocation and reducing cloud costs.
Explanation:
--app-id app_id
: References the specific Amplify app from which you want to delete the backend environment.--environment-name env_name
: Identifies which backend environment should be removed, ensuring that targeted resources are decommissioned.
Example Output:
{
"backendEnvironment": {
"environmentName": "test-environment",
...
}
}
List all backend environments in an Amplify app (with examples)
Code:
aws amplify list-backend-environments --app-id app_id
Motivation:
Listing all backend environments provides a clear overview of all the different environments set up under a specific Amplify app. It is helpful for managing the development lifecycle, ensuring all environments are accounted for, and making informed decisions on development, testing, and deployment processes.
Explanation:
--app-id app_id
: Specifies the Amplify app whose backend environments you want to list, ensuring that you receive information relevant to the correct application.
Example Output:
{
"backendEnvironments": [
{
"environmentName": "dev",
...
},
{
"environmentName": "prod",
...
}
]
}
Conclusion:
AWS Amplify’s command-line functionalities provide comprehensive control and management over your application’s lifecycle, enabling you to rapidly deploy, manage, and scale applications on AWS infrastructure. Through practical use cases, understanding each command helps streamline tasks and empower development workflows effectively.