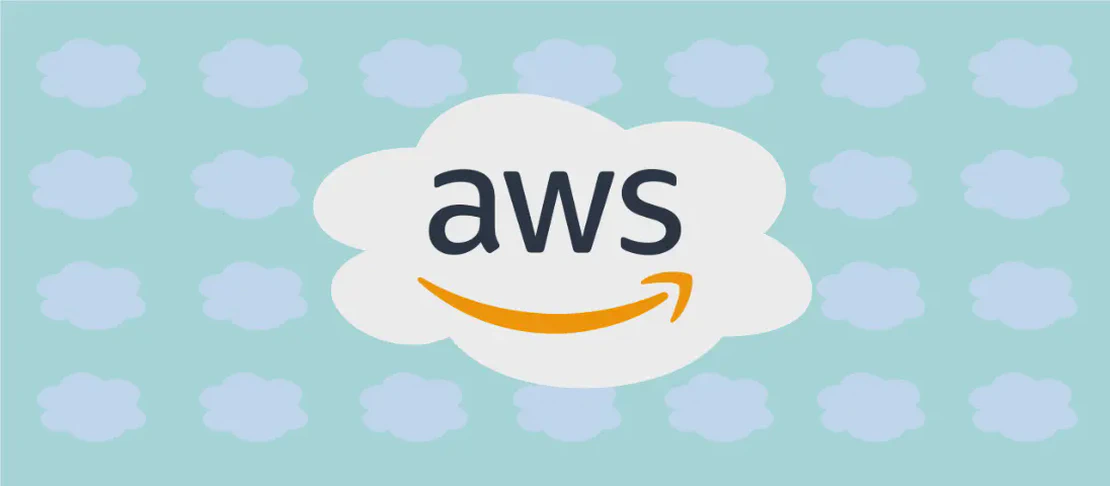
How to use the command 'aws cloudformation' (with examples)
The aws cloudformation
command is a powerful tool within the AWS Command Line Interface (CLI) that allows users to manage and control AWS resources efficiently using a concept called Infrastructure as Code (IaC). By utilizing CloudFormation, users can model, provision, and manage a wide array of AWS and even third-party resources, ensuring that their infrastructure is both scalable and reliable. The tool is particularly valuable in automating deployments, managing cloud configurations consistently, and making resource scaling and optimization a streamlined process. In this article, we will explore several common use cases where the aws cloudformation
command can greatly enhance cloud infrastructure management.
Use case 1: Create a stack from a template file
Code:
aws cloudformation create-stack --stack-name stack-name --region region --template-body file://path/to/file.yml --profile profile
Motivation: Creating a stack from a template file is an essential task for deploying and managing AWS resources collectively as a single unit. By defining your infrastructure and application architecture in a template file, you can automate the deployment process and ensure consistency across multiple environments or regions.
Explanation:
create-stack
: This tells CloudFormation to initiate the creation process of a new stack.--stack-name stack-name
: Specifies a unique name for the stack being created, which is used to identify it within your AWS account.--region region
: Identifies the specific AWS region where you want the stack and its resources to be created.--template-body file://path/to/file.yml
: Refers to the local file path containing the CloudFormation template that defines the AWS resources and their configurations.--profile profile
: Specifies which profile from the AWS credentials file to use when executing the command, allowing the user to manage multiple AWS accounts with ease.
Example Output:
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-name/guid"
}
Use case 2: Delete a stack
Code:
aws cloudformation delete-stack --stack-name stack-name --profile profile
Motivation: Deleting a stack is crucial when you need to tear down AWS resources that are no longer needed to avoid incurring unnecessary costs. This command helps clean up resources effectively, ensuring that you only pay for what you’re actively using.
Explanation:
delete-stack
: This command signals the deletion process for the specified stack.--stack-name stack-name
: Identifies the stack that is to be deleted, as defined in the original creation command.--profile profile
: Determines which AWS credentials to use, facilitating the management of resources across multiple AWS environments.
Example Output: (No explicit output is returned upon successful deletion, but the stack status can be checked through other commands.)
Use case 3: List all stacks
Code:
aws cloudformation list-stacks --profile profile
Motivation: Listing all stacks is beneficial for gaining insight into the current state of resources managed by CloudFormation across your account. This command helps administrators get a quick overview of all deployed stacks for monitoring, auditing, or further management purposes.
Explanation:
list-stacks
: Instructs CloudFormation to list all stacks within the account.--profile profile
: Allows for specification of AWS credentials to list stacks under a particular AWS environment or account.
Example Output:
{
"StackSummaries": [
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-1/guid",
"StackName": "stack-1",
"CreationTime": "2023-01-01T12:34:56.789Z",
"StackStatus": "CREATE_COMPLETE"
},
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-2/guid",
"StackName": "stack-2",
"CreationTime": "2023-01-02T12:34:56.789Z",
"StackStatus": "UPDATE_COMPLETE"
}
]
}
Use case 4: List all running stacks
Code:
aws cloudformation list-stacks --stack-status-filter CREATE_COMPLETE --profile profile
Motivation: Focusing on the running stacks can help in identifying which stacks are currently active and potentially consuming resources. This is particularly useful for cost analysis and to ensure that necessary services are running optimally.
Explanation:
list-stacks
: Asks CloudFormation to return a list of stacks.--stack-status-filter CREATE_COMPLETE
: Filters the stacks based on their creation status, where only stacks that have been created successfully and are running are listed.--profile profile
: Utilizes specific AWS credentials for accessing the account and retrieving the stacks.
Example Output:
{
"StackSummaries": [
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-1/guid",
"StackName": "stack-1",
"CreationTime": "2023-01-01T12:34:56.789Z",
"StackStatus": "CREATE_COMPLETE"
}
]
}
Use case 5: Check the status of a stack
Code:
aws cloudformation describe-stacks --stack-name stack-id --profile profile
Motivation: Checking the status of a stack is an integral part of monitoring and maintaining AWS deployments. It helps administrators verify that resources are in the expected state and to quickly identify any discrepancies or failures in the stack lifecycle.
Explanation:
describe-stacks
: Requests detailed information about one or more stacks.--stack-name stack-id
: Specifies which stack to describe, requiring either the name or unique ID of the stack.--profile profile
: Uses the provided AWS credentials to access and describe the specific stack.
Example Output:
{
"Stacks": [
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-id/guid",
"StackName": "stack-name",
"StackStatus": "CREATE_COMPLETE",
"CreationTime": "2023-01-01T12:34:56.789Z",
"Outputs": [
{
"OutputKey": "Output1",
"OutputValue": "Value1",
"Description": "Description1"
}
]
}
]
}
Use case 6: Initiate drift detection for a stack
Code:
aws cloudformation detect-stack-drift --stack-name stack-id --profile profile
Motivation: Drift detection is crucial for ensuring that the stack resources currently deployed in AWS match the configurations defined in the CloudFormation template. Over time, changes can occur directly in resources without updating the template, creating drift. This command helps detect such inconsistencies and prompt necessary updates or corrections.
Explanation:
detect-stack-drift
: Initiates the process of checking for any drift in the stack resources.--stack-name stack-id
: Identifies the stack to check drift against using its unique stack ID or name.--profile profile
: Specifies which AWS account credentials to apply for drift detection on the stack.
Example Output:
{
"StackId": "arn:aws:cloudformation:region:account-id:stack/stack-id/guid",
"StackDriftDetectionId": "12345678-1234-1234-1234-123456789012"
}
Use case 7: Check the drift status output of a stack using ‘StackDriftDetectionId’ from the previous command output
Code:
aws cloudformation describe-stack-resource-drifts --stack-name stack-drift-detection-id --profile profile
Motivation: Checking the drift status provides insight into which specific resources within the stack are experiencing drift and require attention. This plays a key role in maintaining alignment between the actual deployed infrastructure and its intended state as per the configuration files.
Explanation:
describe-stack-resource-drifts
: Retrieves detailed information about drift status for the specified stack resources.--stack-name stack-drift-detection-id
: Refers to the unique identifier obtained from initiating drift detection.--profile profile
: Determines what credentials and account information to use when checking for drift.
Example Output:
{
"StackResourceDrifts": [
{
"LogicalResourceId": "MyResource1",
"PhysicalResourceId": "resource-id-12345",
"ResourceType": "AWS::S3::Bucket",
"ExpectedProperties": "{\"VersioningConfiguration\":{\"Status\":\"Enabled\"}}",
"ActualProperties": "{\"VersioningConfiguration\":{\"Status\":\"Suspended\"}}",
"PropertyDifferences": [
{
"PropertyPath": "/VersioningConfiguration/Status",
"ExpectedValue": "Enabled",
"ActualValue": "Suspended",
"DifferenceType": "NOT_EQUAL"
}
],
"StackResourceDriftStatus": "MODIFIED"
}
]
}
Conclusion:
By leveraging the aws cloudformation
commands, administrators and developers can efficiently manage, monitor, and maintain their AWS infrastructure as code. Each command serves a specialized purpose, helping users automate deployments, control cloud costs, and ensure that their infrastructure remains consistent and aligned with predefined templates. Whether you’re scaling applications or auditing environments, these tools are essential for effective AWS cloud management.