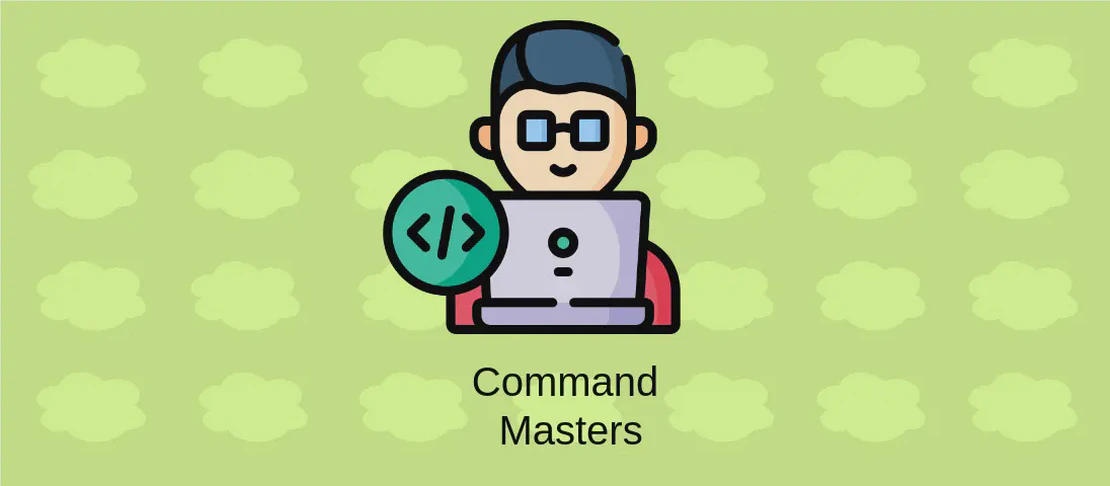
How to Use the Command 'aws dynamodb' (with Examples)
The aws dynamodb
command-line interface (CLI) is an essential tool for managing Amazon DynamoDB, a fast and flexible NoSQL database service provided by AWS. It provides predictable performance with seamless scalability, allowing developers and data engineers to manipulate their databases directly from the command line without needing to access the AWS Management Console. With aws dynamodb
, users can perform a variety of tasks such as creating tables, managing items, and scanning data, which are crucial for the smooth operation and maintenance of any data-driven application.
Create a Table (with Examples)
Code:
aws dynamodb create-table --table-name table_name --attribute-definitions AttributeName=S,AttributeType=S --key-schema AttributeName=S,KeyType=HASH --provisioned-throughput ReadCapacityUnits=5,WriteCapacityUnits=5
Motivation:
Creating a table in DynamoDB is the first step in managing structured data for an application. This table acts as the storage location for all items and their attributes and is akin to a table in a relational database, albeit without a fixed schema. By establishing a table, you set the foundation for future data interactions, which is critical for any business logic or data processing needs.
Explanation:
--table-name table_name
: Specifies the name of the table to be created. It identifies the table within your AWS account and within the specific region you’re operating in.--attribute-definitions AttributeName=S,AttributeType=S
: Defines the attributes for the hash key.AttributeName
is the name of the attribute, whileAttributeType
can beS
(string),N
(number), orB
(binary), determining the type of the attribute.--key-schema AttributeName=S,KeyType=HASH
: Sets up the primary key by specifying the attribute to be used as the key and defining it as a hash key.--provisioned-throughput ReadCapacityUnits=5,WriteCapacityUnits=5
: Declares the read and write capacity for throughput provisioning, enabling you to configure how much DynamoDB should provision for handling requests efficiently.
Example Output:
On executing the command, you should receive a response confirming the creation of the table, sharing details about the table status, key schema, provisioned throughput, and other essential attributes of the table.
List All Tables in the DynamoDB (with Examples)
Code:
aws dynamodb list-tables
Motivation:
Listing all tables is useful for managing and verifying the presence of your databases, especially in environments where multiple databases might be handled by different teams or for different applications. It provides an overview of all the available tables, facilitating inventory management and ease of access when figuring out relationships between different datasets.
Explanation:
Executing this command without any parameters displays all DynamoDB tables within the configured AWS region. It provides a simple inventory list without digging into specific attributes or data contained within the tables.
Example Output:
The command will output the list of all DynamoDB table names, typically in JSON format, showcasing each table’s text identifier.
Get Details About a Specific Table (with Examples)
Code:
aws dynamodb describe-table --table-name table_name
Motivation:
Getting specifics about a table is essential for understanding its current configuration and status. This operation is crucial for database administrators who need to ensure that table settings align with application requirements or for troubleshooting performance or data retrieval issues.
Explanation:
--table-name table_name
: Indicate the particular table whose details you wish to obtain. This parameter ensures that only the specified table is described, thus providing you with detailed metadata including attributes, provisioned throughput, the table’s unique status, and indexes.
Example Output:
You would receive a comprehensive JSON output detailing various attributes of the table such as creation date, item count, key schema, and provisioned throughput information, helping you to keep track of configuration and performance metrics.
Add an Item to a Table (with Examples)
Code:
aws dynamodb put-item --table-name table_name --item '{"AttributeName": {"S": "value"}}'
Motivation:
Adding items is a fundamental operation performed whenever new data needs to be recorded into the database. This action is vital for applications that collect data, requiring the consistent updating of entities such as user profiles, transactions, or logs.
Explanation:
--table-name table_name
: Specifies the table where the new item will be placed.--item '{"AttributeName": {"S": "value"}}'
: This JSON-formatted argument defines the new item to be added, including the attributes and their data types. For instance,AttributeName
defines the name of the attribute andS
specifies that the attribute is a string.
Example Output:
Upon successful execution, the command will exit with no errors, confirming that the specified item has been inserted into the table with the desired attribute values.
Retrieve an Item from a Table (with Examples)
Code:
aws dynamodb get-item --table-name {{table_name}} --key '{"ID": {"N": "1"}}'
Motivation:
Data retrieval is one of the most frequently performed operations within any database system. It allows applications to read and leverage stored data, critical for any feature or functionality that depends on previously recorded information.
Explanation:
--table-name {{table_name}}
: Indicates which table to look through to find the matching item.--key '{"ID": {"N": "1"}}'
: Specifies the primary key of the item to be retrieved. Here,ID
is the attribute being used as the primary key and1
is its numeric value, permitting precise retrieval.
Example Output:
The command will produce output in JSON format, returning the item with all its attributes that match the provided key, which can then be used or displayed by the application.
Update an Item in the Table (with Examples)
Code:
aws dynamodb update-item --table-name {{table_name}} --key '{{{"ID": {"N": "1"}}}}' --update-expression "{{SET Name = :n}}" --expression-attribute-values '{{{":n": {"S": "Jane"}}}}'
Motivation:
Updating items is crucial whenever the stored data needs modifications, whether due to new information or corrections. This operation is essential for maintaining data accuracy and relevance, which underpins any data-driven application’s integrity.
Explanation:
--table-name {{table_name}}
: Names the target table whose item needs updating.--key '{{{"ID": {"N": "1"}}}}'
: Selects the specific item to modify based on its key.--update-expression "{{SET Name = :n}}"
: Describes the transformations to perform on the item, in this case, setting a new value calledName
.--expression-attribute-values '{{{":n": {"S": "Jane"}}}}'
: Maps the placeholder name to the actual value for the update, herein changingName
to “Jane”.
Example Output:
A successful update will be acknowledged without any direct output unless errors occur in the procedure, thereby affirming the alterations made to the item’s attributes.
Scan Items in the Table (with Examples)
Code:
aws dynamodb scan --table-name {{table_name}}
Motivation:
Scanning is an efficient way to retrieve multiple items from a table without using indexes, which is helpful when performing data analysis or batch processing tasks. It reveals all items in the table, which is invaluable for full-table analyses where attributes aren’t predetermined.
Explanation:
--table-name {{table_name}}
: Identifies the table to be scanned for retrieving all included items.
Example Output:
The output renders every item of the specified table, showcasing all attributes in JSON format. Although generally quick, using scan
over vast tables can be computationally expensive, potentially impacting performance.
Delete an Item from the Table (with Examples)
Code:
aws dynamodb delete-item --table-name {{table_name}} --key '{{{"ID": {"N": "1"}}}}'
Motivation:
Deleting items is sometimes necessary to manage storage costs, comply with retention policies, or simply remove outdated and irrelevant data. Performing this operation ensures that only necessary data occupies the database, safeguarding resource efficiency.
Explanation:
--table-name {{table_name}}
: Specifies from which table to remove the item.--key '{{{"ID": {"N": "1"}}}}'
: Targets the exact item to be deleted based on its key, uniquely identifying which data entry to expunge.
Example Output:
Executing the command should eliminate the indicated item from the database without any error notifications, thus confirming the item’s deletion.
Conclusion:
The aws dynamodb
CLI commands provide a comprehensive toolkit for managing DynamoDB databases through efficient, command-line actions. Whether you are automating table creation, retrieving complex datasets, or performing necessary updates and deletions, understanding each command’s nuances enables seamless database interaction and management, ensuring optimal performance and efficient data handling for any application leveraging cloud data services.