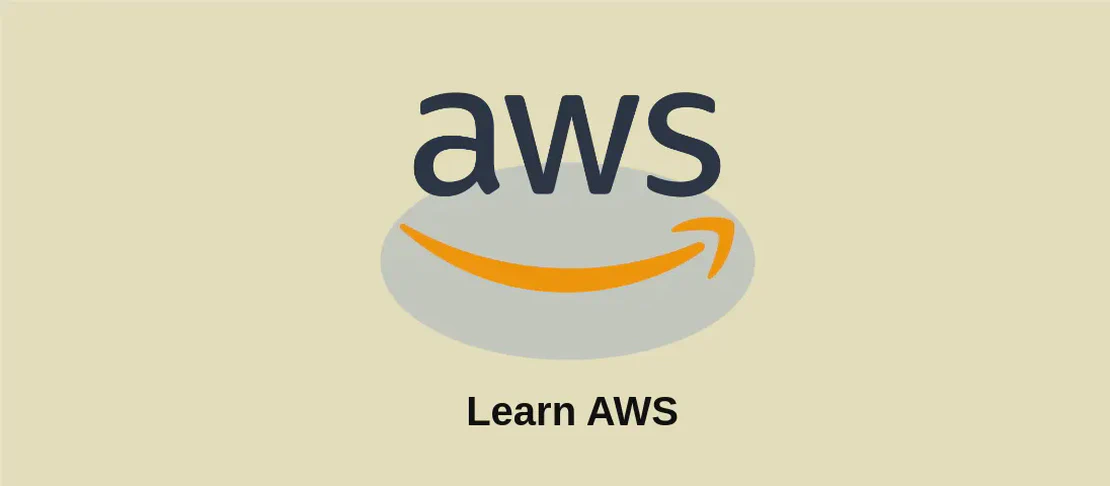
Managing AWS EC2 Instances and Volumes Using the AWS CLI (with Examples)
Amazon Elastic Compute Cloud (EC2) is a web service that provides scalable and resizable compute capacity in the Amazon Web Services (AWS) cloud. It is designed to make web-scale computing easier for developers by offering a variety of instance types and sizes allowing for optimal allocation of resources. The AWS CLI (aws ec2
) simplifies accessing and managing EC2 resources, enabling developers and system administrators to automate infrastructure management tasks efficiently.
Use case 1: Display Information About a Specific Instance
Code:
aws ec2 describe-instances --instance-ids instance_id
Motivation:
Knowing the specific details of an instance is crucial for infrastructure management. This information aids in diagnosing issues, ensuring configurations are correct, and understanding the current status and parameters of the instance. This specific command is frequently used by administrators who manage multiple instances and need to ensure the proper functioning of certain critical workloads.
Explanation:
describe-instances
: This command retrieves configuration details about your EC2 instances.--instance-ids
: This option specifies the unique identifier(s) of the instance(s) you want information about. You replaceinstance_id
with your specific instance ID, such asi-0123456789abcdef0
.
Example Output:
{
"Reservations": [
{
"Instances": [
{
"InstanceId": "i-0123456789abcdef0",
"ImageId": "ami-0abcdef1234567890",
"State": {
"Code": 16,
"Name": "running"
},
"InstanceType": "t2.micro",
...
}
]
}
]
}
Use case 2: Display Information About All Instances
Code:
aws ec2 describe-instances
Motivation:
Managing multiple instances often necessitates a holistic view of all instances to monitor their states, determine resource allocations, or manage configurations. This command is crucial for cloud administrators or developers who need to understand the current landscape of compute resources they have deployed.
Explanation:
describe-instances
: Without specifying aninstance-id
, this command will retrieve details about all instances within the user’s AWS account. This is particularly useful when managing a large number of instances or when wanting to audit resources.
Example Output:
{
"Reservations": [
{
"Instances": [
{
"InstanceId": "i-0123456789abcdef0",
"ImageId": "ami-0abcdef1234567890",
"State": {
"Code": 16,
"Name": "running"
},
"InstanceType": "t2.micro",
...
}
]
},
...
]
}
Use case 3: Display Information About All EC2 Volumes
Code:
aws ec2 describe-volumes
Motivation:
Volumes store data permanently across stop, start, and reboot cycles of EC2 instances. By examining all volumes, administrators and engineers can check for available storage, track utilization, and identify potential issues with volumes, such as running low on space.
Explanation:
describe-volumes
: This command fetches information about all EBS volumes. It helps in understanding the current status and configuration, including size and attachment, of each volume.
Example Output:
{
"Volumes": [
{
"VolumeId": "vol-1234567890abcdef0",
"Size": 8,
"State": "available",
"VolumeType": "gp2",
...
},
...
]
}
Use case 4: Delete an EC2 Volume
Code:
aws ec2 delete-volume --volume-id volume_id
Motivation:
Removing unused or obsolete volumes helps in managing resource usage and reducing unnecessary costs. It can be part of a cleanup process to ensure that only active, required volumes consume resources within your AWS account.
Explanation:
delete-volume
: This command removes an existing EBS volume permanently from your AWS account.--volume-id
: This specifies the unique identifier of the volume you want to delete. Replacevolume_id
with the actual volume ID, such asvol-1234567890abcdef0
.
Example Output:
There is no JSON output, but a successful deletion results in no error message and returns an empty response.
Use case 5: Create a Snapshot From an EC2 Volume
Code:
aws ec2 create-snapshot --volume-id volume_id
Motivation:
Creating snapshots of EBS volumes is essential for data backup, disaster recovery, or maintaining versioned copies of data. Snapshots are an integral part of a robust data management strategy, offering quick restoration points in case of data failure or corruption.
Explanation:
create-snapshot
: This command initiates the process of creating a snapshot of the specified EBS volume.--volume-id
: This specifies the ID of the volume for which you wish to create a snapshot. Replacevolume_id
with the actual volume ID.
Example Output:
{
"SnapshotId": "snap-1234567890abcdef0",
"VolumeId": "vol-1234567890abcdef0",
"State": "pending",
...
}
Use case 6: List Available AMIs (Amazon Machine Images)
Code:
aws ec2 describe-images
Motivation:
AWS offers a variety of pre-configured images allowing quick application deployment; retrieving a list of available AMIs helps users find proper starting points based on their applications’ requirements, organizational policies, or compliance needs.
Explanation:
describe-images
: This command retrieves information about available AMIs. By default, it will list AMIs within the specified region owned by the user, and can be extended to include public or shared AMIs.
Example Output:
{
"Images": [
{
"ImageId": "ami-0abcdef1234567890",
"Name": "my-custom-ami",
...
},
...
]
}
Use case 7: Show List of All Available EC2 Commands
Code:
aws ec2 help
Motivation:
The AWS CLI supports numerous operations and configurations for EC2. Accessing a comprehensive list of all available commands helps users, especially newcomers, to understand the breadth of tasks possible with EC2 and learn specific commands to fulfill their needs.
Explanation:
help
: This argument displays a detailed list of all available EC2 commands, aiding in navigation of the myriad options and utilities the CLI offers for EC2 management.
Example Output:
Output will be a comprehensive list of all EC2 commands, each with a brief description of what it does.
Use case 8: Display Help for Specific EC2 Subcommand
Code:
aws ec2 subcommand help
Motivation:
As the AWS CLI contains many subcommands, understanding their specific arguments and options is crucial for effective use. Accessing command-specific help provides vital information for users to implement and execute commands accurately without errors.
Explanation:
subcommand help
: Replacesubcommand
with the specific EC2 subcommand you seek assistance on, such asdescribe-instances
orcreate-snapshot
. This displays detailed help about the selected subcommand, including options, parameters, and examples.
Example Output:
The output will detail all options, parameters, and usage instructions for the specified subcommand.
Conclusion:
Mastering the aws ec2
command line tool significantly enhances your ability to manage AWS EC2 instances and volumes efficiently. Each use case above provides a glimpse into the robust capabilities offered by EC2 via the AWS CLI, showcasing various administrative tasks—from fetching data to provisioning and deallocating resources. Understanding these tools empowers developers and system administrators to optimize cloud infrastructure use, automate routine tasks, and ensure optimal performance and resource management.