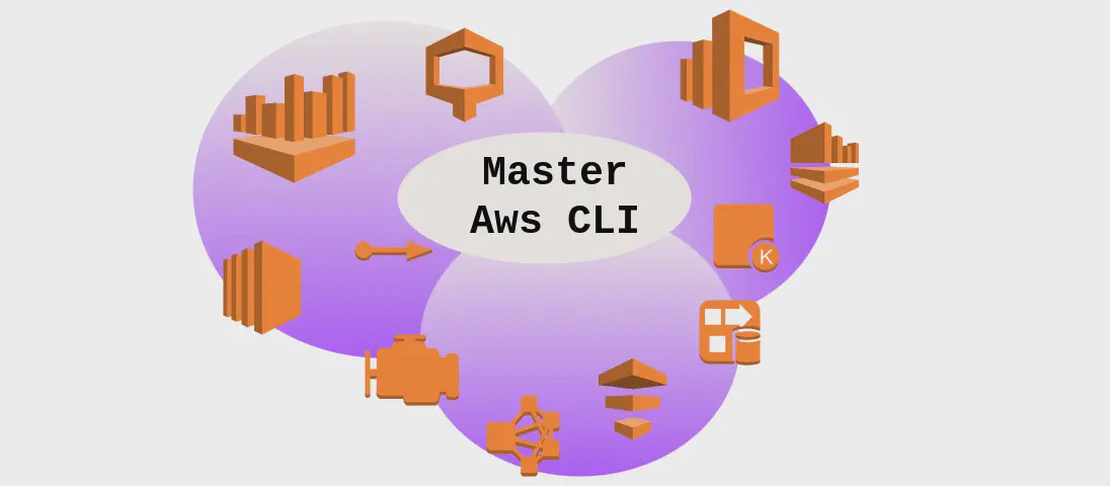
How to Use the Command 'aws ecr' (with Examples)
The AWS Elastic Container Registry (ECR) is a fully managed Docker container registry that makes it straightforward for developers to store, manage, and deploy Docker container images. The aws ecr
command-line interface provides several commands for interacting with the registry, including authentication, creating and deleting repositories, and managing container images. This article illustrates various use cases of the aws ecr
command to help you leverage these functionalities effectively.
Use case 1: Authenticate Docker with the Default Registry
Code:
aws ecr get-login-password --region region | docker login --username AWS --password-stdin aws_account_id.dkr.ecr.region.amazonaws.com
Motivation:
Before you can push or pull images to/from your Amazon ECR registry, Docker needs to be authenticated with the registry. This command provides a simple way to authenticate Docker with the default AWS registry using the AWS CLI. Authentication is crucial to ensure that only authorized users can access and manage your private container images.
Explanation:
aws ecr get-login-password
: This command fetches a password that will be used to log in to the ECR.--region region
: This specifies the AWS region where your ECR is hosted. Replaceregion
with your actual AWS region, such asus-west-2
.docker login --username AWS
: This logs into Docker using the username ‘AWS’, which is the default for ECR.--password-stdin
: This option allows Docker to read the password from the standard input.aws_account_id.dkr.ecr.region.amazonaws.com
: This refers to the endpoint of your ECR, whereaws_account_id
is your AWS account number andregion
corresponds to your specified AWS region.
Example Output:
Login Succeeded
Use case 2: Create a Repository
Code:
aws ecr create-repository --repository-name repository --image-scanning-configuration scanOnPush=true --region region
Motivation:
Creating a repository in AWS ECR is the first step to storing your docker images. It organizes your Docker images based on logical groupings relevant to your projects or applications. This setup helps in managing the lifecycle and distribution of container images efficiently.
Explanation:
aws ecr create-repository
: Initiates the process to create a new repository within ECR.--repository-name repository
: Specifies the name of the repository you wish to create. Replacerepository
with your desired repository’s name.--image-scanning-configuration scanOnPush=true
: This optional flag enables image scanning each time an image is pushed, which increases security by automatically checking for vulnerabilities.--region region
: Indicates the AWS region where the repository will be created, requiring you to substituteregion
with your intended region.
Example Output:
{
"repository": {
"repositoryArn": "arn:aws:ecr:region:aws_account_id:repository/repository",
"repositoryUri": "aws_account_id.dkr.ecr.region.amazonaws.com/repository",
"createdAt": "2023-10-17T12:34:56Z",
"imageTagMutability": "MUTABLE",
"imageScanningConfiguration": {
"scanOnPush": true
}
}
}
Use case 3: Tag a Local Image for ECR
Code:
docker tag container_name:tag aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
Motivation:
Tagging a local Docker image with an ECR-specific tag is a prerequisite to pushing it to the registry. This operation essentially names and prepares the image for the push operation by associating it with your AWS account and the desired repository in ECR.
Explanation:
docker tag container_name:tag
: Thedocker tag
command assigns a new name to an existing image.container_name:tag
should match the current name and tag of your local image.aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
: This new tag links the image to your AWS ECR. Theaws_account_id
is your AWS account number, theregion
denotes your AWS region,container_name
is the desired name of the image, andtag
is the specific version you want to associate with the image.
Example Output:
(There is typically no output for a successful docker tag command)
Use case 4: Push an Image to a Repository
Code:
docker push aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
Motivation:
Pushing images to a repository is crucial for making your locally developed Docker containers available for deployment and sharing across your organization. By storing them in ECR, you can ensure they are easily accessible and that authorized users can deploy them across various AWS services and environments.
Explanation:
docker push
: This command uploads the specified image to the repository.aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
: It refers to the already tagged image you wish to push, whereaws_account_id
is your AWS account ID,region
is your specific AWS region,container_name
is the name of the container, andtag
is the version of the image.
Example Output:
The push refers to repository [aws_account_id.dkr.ecr.region.amazonaws.com/container_name]
digest: sha256:xxxx size: 1234
Use case 5: Pull an Image from a Repository
Code:
docker pull aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
Motivation:
Pulling an image from ECR allows you to retrieve and use container images stored in the registry. This functionality is essential for deploying applications on new environments or systems that need the specific container images.
Explanation:
docker pull
: Fetches an image from a Docker repository.aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
: Specifies the image to be pulled, whereaws_account_id
denotes your AWS account ID,region
is the region,container_name
is the container’s name, andtag
is the version tag of the image.
Example Output:
Using default tag: latest
latest: Pulling from container_name
Digest: sha256:xxxx
Status: Image is up to date for aws_account_id.dkr.ecr.region.amazonaws.com/container_name:tag
Use case 6: Delete an Image from a Repository
Code:
aws ecr batch-delete-image --repository-name repository --image-ids imageTag=latest
Motivation:
Deleting old or unused images from your ECR repository is a best practice for minimizing storage costs and keeping your repository organized. Removing obsolete images also reduces the risk of deploying outdated versions of an application.
Explanation:
aws ecr batch-delete-image
: This command batch deletes specified images from a repository.--repository-name repository
: Indicates the repository from which the image needs to be deleted. Replacerepository
with your target repository’s name.--image-ids imageTag=latest
: Identifies the specific image to delete by its tag. You can also specify an image byimageDigest
.
Example Output:
{
"imageIds": [
{
"imageTag": "latest"
}
],
"failures": []
}
Use case 7: Delete a Repository
Code:
aws ecr delete-repository --repository-name repository --force
Motivation:
Deleting a repository when it is no longer needed helps manage resources and remove unwanted data, thus saving on storage costs. You might want to perform this action when a project is completed, or you’re restructuring your repository organization.
Explanation:
aws ecr delete-repository
: Deletes a specified repository from ECR.--repository-name repository
: Specifies the name of the repository to delete. Replacerepository
with your repository’s name.--force
: This flag deletes the repository even if it contains images. Without this flag, the repository must be empty before it can be deleted.
Example Output:
{
"repository": {
"repositoryArn": "arn:aws:ecr:region:aws_account_id:repository/repository",
"registryId": "aws_account_id",
"repositoryName": "repository",
"repositoryUri": "aws_account_id.dkr.ecr.region.amazonaws.com/repository"
}
}
Use case 8: List Images within a Repository
Code:
aws ecr list-images --repository-name repository
Motivation:
Listing images in a repository provides an overview of available container images and their versions. This can assist in managing versions, deploying specific images, and conducting audits.
Explanation:
aws ecr list-images
: Lists all images contained within a specified repository.--repository-name repository
: Identifies the repository whose images will be listed. Replacerepository
with the name of your repository of interest.
Example Output:
{
"imageIds": [
{
"imageDigest": "sha256:xxxx",
"imageTag": "latest"
},
{
"imageDigest": "sha256:yyyy",
"imageTag": "stable"
}
]
}
Conclusion:
AWS ECR is a crucial service for managing Docker container images securely and efficiently in the cloud. The various commands within the aws ecr
suite provide comprehensive functionalities to authenticate, manage repositories, and handle container images. With these use cases, you should be better equipped to utilize AWS ECR for container management in your cloud-native applications. Whether you’re orchestrating a small project or deploying enterprise-grade applications, understanding these commands will aid in maintaining an organized, cost-effective, and secure image registry.