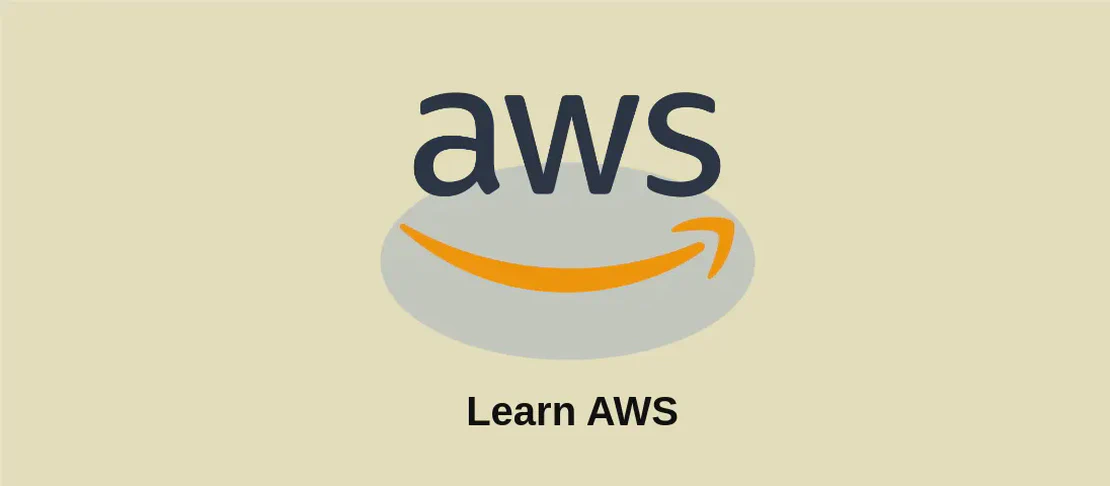
Interacting with AWS IAM Using AWS CLI (with examples)
The AWS Command Line Interface (CLI) allows users to interact with AWS services directly from the command line. One of the core services in AWS is Identity and Access Management (IAM), which enables secure control over access to AWS services and resources for users within your organization. The ‘aws iam’ command provides a range of functionalities for managing users, groups, policies, and access keys in AWS IAM. In this article, we explore various use cases of the ‘aws iam’ command, offering practical examples, motivations, detailed explanations of the command arguments, and example outputs to demonstrate its utility.
Use case 1: List users
Code:
aws iam list-users
Motivation: Listing users is a crucial activity for administrators who need to monitor and manage who has access to an AWS account. By generating a complete inventory of IAM users, the admin can ensure that only authorized personnel have access and that appropriate permissions are in place. This task is essential for auditing and compliance purposes.
Explanation:
aws iam list-users
: This command does not require any additional arguments. It queries AWS IAM and returns a list of all users within the AWS account. The simplicity of the command makes it accessible and straightforward for quickly obtaining user details.
Example Output:
{
"Users": [
{
"Path": "/",
"UserName": "JohnDoe",
"UserId": "AID123EXAMPLE",
"Arn": "arn:aws:iam::123456789012:user/JohnDoe",
"CreateDate": "2020-01-15T12:34:56Z"
},
{
"Path": "/",
"UserName": "JaneSmith",
"UserId": "AID456EXAMPLE",
"Arn": "arn:aws:iam::123456789012:user/JaneSmith",
"CreateDate": "2019-11-23T09:21:22Z"
}
]
}
Use case 2: List policies
Code:
aws iam list-policies
Motivation: Listing policies is essential for understanding and managing which permissions are defined within your AWS account. Policies determine what actions users and services can perform, and knowing existing policies helps in controlling and auditing permissions, ensuring security and compliance measures are adhered to.
Explanation:
aws iam list-policies
: This command lists all the IAM policies available in the AWS account. It does not require any additional arguments, simplifying the process of identifying available policies.
Example Output:
{
"Policies": [
{
"PolicyName": "AdministratorAccess",
"PolicyId": "ABCDEFGHI123456789",
"Arn": "arn:aws:iam::aws:policy/AdministratorAccess",
"Path": "/",
"DefaultVersionId": "v1",
"AttachmentCount": 10,
"IsAttachable": true,
"CreateDate": "2015-06-15T11:20:23Z",
"UpdateDate": "2020-03-18T14:28:56Z"
},
{
"PolicyName": "ReadOnlyAccess",
"PolicyId": "JKLMNOPQ6789054321",
"Arn": "arn:aws:iam::aws:policy/ReadOnlyAccess",
"Path": "/",
"DefaultVersionId": "v3",
"AttachmentCount": 25,
"IsAttachable": true,
"CreateDate": "2014-09-01T09:15:47Z",
"UpdateDate": "2019-12-02T16:17:18Z"
}
]
}
Use case 3: List groups
Code:
aws iam list-groups
Motivation: Listing user groups is beneficial for understanding the organization of permissions and responsibilities within an AWS environment. Groups simplify the management of multiple users, and knowing existing groups allows administrators to efficiently manage user access and policies.
Explanation:
aws iam list-groups
: This command retrieves and lists all IAM groups within the AWS account. Like previous commands, it requires no additional arguments for basic usage, making it straightforward to obtain group information.
Example Output:
{
"Groups": [
{
"Path": "/",
"GroupName": "Admins",
"GroupId": "AGPAI234EXAMPLE",
"Arn": "arn:aws:iam::123456789012:group/Admins",
"CreateDate": "2015-02-01T00:52:34Z"
},
{
"Path": "/",
"GroupName": "Developers",
"GroupId": "AGPHJ678EXAMPLE",
"Arn": "arn:aws:iam::123456789012:group/Developers",
"CreateDate": "2017-06-10T15:22:01Z"
}
]
}
Use case 4: Get users in a group
Code:
aws iam get-group --group-name group_name
Motivation: Understanding which users are part of a specific IAM group is vital for access management and troubleshooting. By identifying users within a group, administrators can audit access permissions, ensure compliance with policy, and make informed decisions about adding or removing members.
Explanation:
aws iam get-group
: The command retrieves details about a specified IAM group, including user membership.--group-name group_name
: Specifies the name of the group for which user membership is being queried. This argument is crucial for targeting the correct group and obtaining relevant data.
Example Output:
{
"Users": [
{
"Path": "/",
"UserName": "Alice",
"UserId": "AIDZ234EXAMPLE",
"Arn": "arn:aws:iam::123456789012:user/Alice",
"CreateDate": "2020-05-18T09:30:45Z"
}
],
"Group": {
"Path": "/",
"GroupName": "Developers",
"GroupId": "AGPHJ678EXAMPLE",
"Arn": "arn:aws:iam::123456789012:group/Developers",
"CreateDate": "2017-06-10T15:22:01Z"
}
}
Use case 5: Describe an IAM policy
Code:
aws iam get-policy --policy-arn arn:aws:iam::aws:policy/policy_name
Motivation: Administrators often need to review the specifics of IAM policies to understand granted permissions and evaluate compliance with security standards. Describing a policy helps in auditing and assessing whether current policies align with organizational security requirements.
Explanation:
aws iam get-policy
: This command retrieves metadata about a specified IAM policy.--policy-arn arn:aws:iam::aws:policy/policy_name
: Provides the Amazon Resource Name (ARN) of the policy being described. The ARN uniquely identifies the policy, ensuring precise retrieval of information.
Example Output:
{
"Policy": {
"PolicyName": "ReadOnlyAccess",
"PolicyId": "ABCDEFGHIJKLMNOP12345",
"Arn": "arn:aws:iam::aws:policy/ReadOnlyAccess",
"Path": "/",
"DefaultVersionId": "v3",
"AttachmentCount": 25,
"PermissionsBoundaryUsageCount": 0,
"IsAttachable": true,
"CreateDate": "2014-09-01T09:15:47Z",
"UpdateDate": "2019-12-02T16:17:18Z"
}
}
Use case 6: List access keys
Code:
aws iam list-access-keys
Motivation: Managing access keys is a fundamental aspect of IAM administration. Listing access keys allows administrators to monitor and control which keys are in use, ensuring that only authorized keys remain active. This action is critical for security and compliance, especially in environments with multiple users and programs accessing AWS resources.
Explanation:
aws iam list-access-keys
: This command returns a list of access keys associated with the IAM user’s AWS account. It aids in simplifying the task of inventorying keys for validation and security purposes.
Example Output:
{
"AccessKeyMetadata": [
{
"UserName": "JohnDoe",
"AccessKeyId": "AKIAIOSFODNNEXAMPLE",
"Status": "Active",
"CreateDate": "2020-01-01T12:00:00Z"
}
]
}
Use case 7: List access keys for a specific user
Code:
aws iam list-access-keys --user-name user_name
Motivation: Focusing on access keys tied to a specific user is crucial for individualized audits and security analysis. Ensuring specific users’ keys are valid, active, and necessary can prevent unauthorized access and reduce the risk of security breaches.
Explanation:
aws iam list-access-keys
: Retrieves a list of access keys.--user-name user_name
: Specifies the user for which the access keys are being listed. This targets the query specifically to that user’s access key data.
Example Output:
{
"AccessKeyMetadata": [
{
"UserName": "JaneSmith",
"AccessKeyId": "AKIAEXAMPLE12345",
"Status": "Active",
"CreateDate": "2019-07-01T11:00:00Z"
}
]
}
Use case 8: Display help
Code:
aws iam help
Motivation: Frequently, users need assistance to understand the full suite of commands and options available within ‘aws iam.’ The help command provides comprehensive guidance, instructions, and examples for efficient use of the tool, enabling users to maximize IAM functionality effectively.
Explanation:
aws iam help
: Upon executing this command, users are presented with detailed information regarding all available subcommands and options within the ‘aws iam’ group, empowering them with needed reference material for all IAM interactions.
Example Output:
I ... N: Use <aws command> - to learn about a specific command. Complete command list:
- aws iam add-user-to-group
- aws iam attach-user-policy
- ...
Conclusion:
Utilizing the ‘aws iam’ command within the AWS CLI empowers administrators to efficiently manage and audit IAM users, groups, policies, and access keys. From auditing access credentials to detailing specific policies, these commands provide robust capabilities for securing and overseeing AWS environments. By understanding each use case, administrators can maintain a secure, compliant, and well-organized AWS environment.