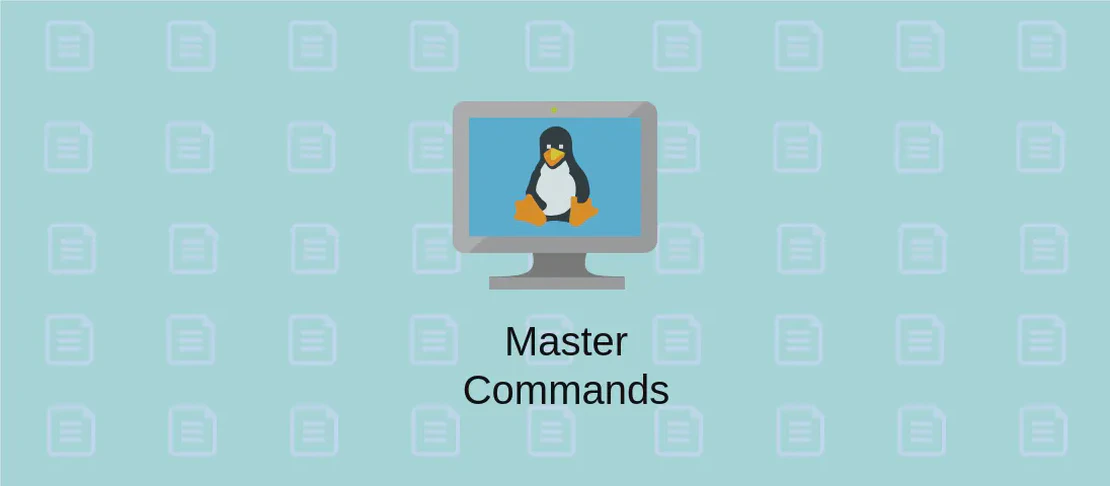
How to use the command 'aws kendra' (with examples)
AWS Kendra is a robust, fully managed enterprise search service powered by machine learning. It enables organizations to index and search their internal data effortlessly across a wide range of content repositories. By leveraging advanced NLP techniques, it provides intelligent search results customized to the user’s query, making it easier to access information within an organization efficiently. The AWS CLI provides a range of commands for AWS Kendra, which allows users to interact programmatically with their Kendra resources.
Use case 1: Create an index
Code:
aws kendra create-index --name MyIndex --role-arn arn:aws:iam::123456789012:role/my-role
Motivation:
Creating an index is a fundamental step in utilizing AWS Kendra, as it allows the service to learn about the data context it will query. Before you can search or query data, AWS Kendra needs a framework—or index—within which it can categorize and manage information. Creating an index sets up this framework and initiates the process by which data sources can be organized and accessed for future search operations. Without an index, the search service couldn’t execute any functions because it wouldn’t have a place to store or navigate the collected information data.
Explanation:
--name MyIndex
: This argument sets a user-friendly name for the new index. It serves as an identifier within the user’s AWS environment and makes it easier for users to reference, manage, and identify the index among potentially many others.--role-arn arn:aws:iam::123456789012:role/my-role
: This argument specifies the ARN (Amazon Resource Name) of the IAM role that has permissions needed for AWS Kendra to perform actions. The role must have sufficient permissions to access the resources needed by Kendra to create and manage the index.
Example output:
Upon successful execution, the command returns details of the created index, most notably the unique Index ID assigned by AWS Kendra.
{
"Id": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111"
}
Use case 2: List indexes
Code:
aws kendra list-indexes
Motivation:
Listing indexes is essential for managing and orchestrating multiple data repositories effectively. By obtaining a list of existing indexes, users can monitor, control, and optimize their Kendra configurations. It allows users to verify the status of indexes, check their existence, and manage them according to their requirements. For instance, this list can be crucial when cleaning up resources, confirming index creation, or when needing to select the correct index to link with specific data sources or search functionalities.
Explanation:
This command does not require any arguments, as it simply retrieves all indexes under the account from the AWS instance.
Example output:
The successful output lists all indexes, including their basic details like Index ID, name, and status.
{
"IndexConfigurationSummaryItems": [
{
"Name": "MyIndex",
"Id": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111",
"Status": "ACTIVE",
"CreatedAt": "2023-01-01T12:00:00.123Z",
"UpdatedAt": "2023-01-02T13:00:00.456Z"
}
]
}
Use case 3: Describe an index
Code:
aws kendra describe-index --id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
Motivation:
Understanding the configuration and status of an index is crucial for maintenance, troubleshooting, and optimization. The describe command provides comprehensive insights into how an index is configured, including specifics around its creation, current status, and key settings. This information is invaluable when diagnosing issues with search performance, validating index settings or when needing to assure stakeholders of the privacy and setup of the indexed data.
Explanation:
--id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
: This argument is the unique identifier of the index you want to describe. It is required to point Kendra to the specific index about which you require detailed information.
Example output:
This command typically returns a detailed description of the index, covering various attributes such as its capacity, roles, and settings.
{
"Name": "MyIndex",
"Id": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111",
"RoleArn": "arn:aws:iam::123456789012:role/my-role",
"Status": "ACTIVE",
"CreatedAt": "2023-01-01T12:00:00.123Z",
"UpdatedAt": "2023-01-02T13:00:00.456Z",
"ServerSideEncryptionConfiguration": {
"KmsKeyId": "arn:aws:kms:region:account-id:key/key-id"
}
}
Use case 4: List data sources
Code:
aws kendra list-data-sources --index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
Motivation:
Managing data sources attached to your Kendra index requires understanding which ones are currently integrated. Listing data sources helps users track and understand the extent of their dataset integration, allowing them to ensure coverage, address gaps, or manage deletions and updates on the sources. This is particularly useful after data sources have been set up over a long period or when multiple collaborators have worked on configuring Kendra.
Explanation:
--index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
: Specifies the ID of the index for which to list the data sources. This confines the result to show only those data sources associated with a given index.
Example output:
The command outputs a list linking to different data sources, their statuses, and types associated with the specified index.
{
"SummaryItems": [
{
"Id": "a1b2c3-datasource-EXAMPLE11111",
"Name": "DataSourceName",
"Type": "S3",
"CreatedAt": "2023-01-01T12:00:00.123Z",
"UpdatedAt": "2023-01-02T13:00:00.456Z",
"Status": "ACTIVE"
}
]
}
Use case 5: Describe a data source
Code:
aws kendra describe-data-source --id a1b2c3-datasource-EXAMPLE11111 --index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
Motivation:
To ensure effective data retrieval, understanding the specifics of each data source is key. Describing a data source gives users the details they need to troubleshoot problems, optimize configurations, or document system setups accurately. The detailed configuration data that this command returns can be integral to recognizing misconfigurations, hacking optimizing settings, or ensuring compliance with security and governance policies.
Explanation:
--id a1b2c3-datasource-EXAMPLE11111
: Specifically, this argument denotes the ID of the data source that’s being described.--index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
: This argument indicates the specific index the data source is attached to, providing context and limiting scope.
Example output:
Successful execution provides a comprehensive description of the data source with its configurations, role, and parameters relevant to the connection.
{
"Name": "DataSourceName",
"Id": "a1b2c3-datasource-EXAMPLE11111",
"IndexId": "a1b2c3d4-5678-90ab-cdef-EXAMPLE11111",
"Type": "S3",
"RoleArn": "arn:aws:iam::123456789012:role/my-role",
"Configuration": {
"S3Configuration": {
"BucketName": "my-bucket"
}
},
"CreatedAt": "2023-01-01T12:00:00.123Z",
"UpdatedAt": "2023-01-02T13:00:00.456Z",
"Status": "ACTIVE"
}
Use case 6: List search queries
Code:
aws kendra list-query-suggestions --index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111 --query-text "example query"
Motivation:
Leveraging query logs is crucial for enhancing search accuracy and relevance. By listing previous search queries, users can discern common search patterns, identify popular information, and spot potential gaps in available data. This insight empowers users to refine their data indexing strategies, enhance machine learning training datasets, and improve the overall usability of the Kendra search application for end-users.
Explanation:
--index-id a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
: This argument references the relevant index containing the query logs and historical search data.--query-text "example query"
: Represents the specific search text or query terms from which to start offering suggestions or analyzing relevant searches.
Example output:
The returned output includes the list of search suggestions or previous query examples based on given text.
{
"QuerySuggestions": [
{
"SuggestionValue": "example query suggestion"
}
]
}
Conclusion:
AWS Kendra, through its comprehensive CLI commands, offers a powerful means for enterprises to effectively build and manage search capabilities across their internal data repositories. Each command and its respective use case provides essential functionality, from initial set-up with index creation to ongoing maintenance and analysis through data source and query management. By making use of these commands, organizations can ensure their information retrieval systems are efficient, accurate, and tailored to their internal needs.