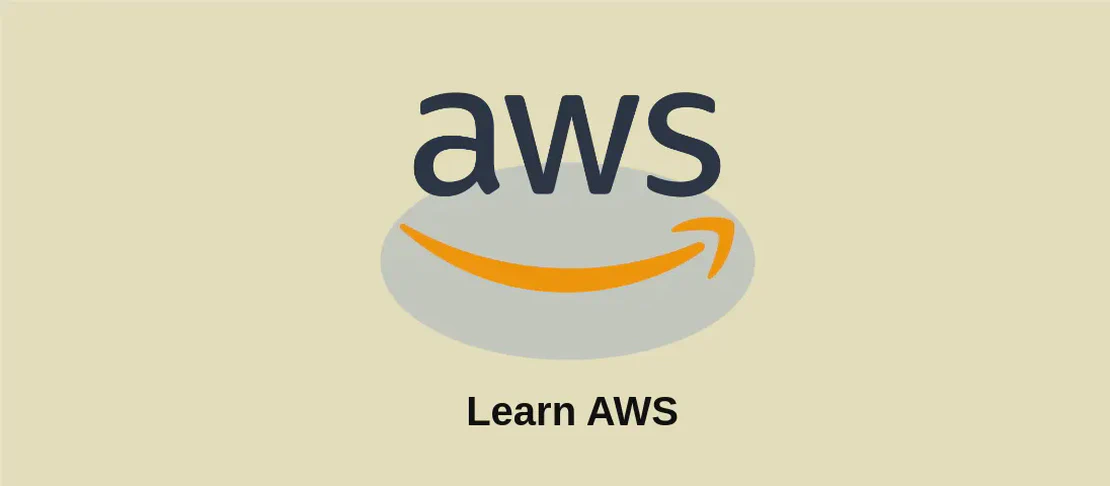
How to Use the Command 'aws lambda' (with examples)
AWS Lambda is a powerful compute service provided by Amazon Web Services that allows you to run code without the need for provisioning or managing servers. It’s particularly useful for running small to medium-sized applications or event-driven functions at scale, providing a flexible solution for executing code in response to various triggers, such as changes to data in an Amazon S3 bucket or updates to a DynamoDB table.
Use case 1: Run a function
Code:
aws lambda invoke --function-name myFunction path/to/response.json
Motivation: Running a Lambda function directly allows you to test deployments and execute code in real-time without needing external triggers. This is helpful for debugging functions or obtaining immediate output results during development cycles.
Explanation:
aws lambda invoke
: This is the base command to invoke a Lambda function.--function-name myFunction
: Specifies the name of the Lambda function you want to execute. Replace “myFunction” with your actual function name.path/to/response.json
: Designates the path to the file where the response from the function call will be stored. This file will contain the output from the Lambda function execution.
Example output: The command generates a response JSON file containing function results, such as:
{
"StatusCode": 200,
"Payload": "Function executed successfully and returned this payload."
}
Use case 2: Run a function with an input payload in JSON format
Code:
aws lambda invoke --function-name myFunction --payload '{"key":"value"}' path/to/response.json
Motivation: Using an input payload allows you to pass custom data or parameters to your Lambda function. This is particularly useful for functions that require user inputs or operational parameters to execute correctly, enabling dynamic and personalized function execution.
Explanation:
aws lambda invoke
: Initiates the invocation of a Lambda function.--function-name myFunction
: States which function to execute by naming it specifically.--payload '{"key":"value"}'
: Specifies the input data (in JSON format) to provide to the function. This payload directly affects the execution of the function and can manipulate its internal operations.path/to/response.json
: The file path where the output response should be save.
Example output: A JSON file at the designated path will contain:
{
"StatusCode": 200,
"Payload": "Processed input: {\"key\":\"value\"}"
}
Use case 3: List functions
Code:
aws lambda list-functions
Motivation: Listing functions in your AWS environment allows you to have an overview of all available Lambda functions within your account, enabling you to manage and organize your services efficiently.
Explanation:
aws lambda list-functions
: A straightforward command without additional parameters, it retrieves and displays a list of all Lambda functions deployed in your AWS account.
Example output: A list of Lambda functions, including their ARNs and configurations:
{
"Functions": [
{
"FunctionName": "myFunction1",
"FunctionArn": "arn:aws:lambda:us-west-2:123456789012:function:myFunction1"
},
{
"FunctionName": "myFunction2",
"FunctionArn": "arn:aws:lambda:us-west-2:123456789012:function:myFunction2"
}
]
}
Use case 4: Display the configuration of a function
Code:
aws lambda get-function-configuration --function-name myFunction
Motivation: Understanding the configuration of a particular Lambda function helps in assessing parameters such as timeout settings, memory allocation, and runtime environment. This information is critical for optimizing your function’s performance and cost efficiency.
Explanation:
aws lambda get-function-configuration
: Base command for retrieving details about function configuration.--function-name myFunction
: Specifies the function whose configuration details you wish to fetch, identified by its unique name.
Example output: The configuration specification returned from the query:
{
"FunctionName": "myFunction",
"Timeout": 3,
"MemorySize": 128,
"Runtime": "python3.8",
"Role": "arn:aws:iam::123456789012:role/lambda-role"
}
Use case 5: List function aliases
Code:
aws lambda list-aliases --function-name myFunction
Motivation: Aliases in AWS Lambda provide a link to a specific function version, acting as pointers that can simplify version management and enable smooth transitions or rollbacks. Listing these can aid in comprehending which versions a given function can be executed under.
Explanation:
aws lambda list-aliases
: Command for obtaining aliases associated with a lambda function.--function-name myFunction
: Specifies the relevant function by name that you are examining for aliases.
Example output: Output showcasing available aliases:
{
"Aliases": [
{
"AliasArn": "arn:aws:lambda:us-west-2:123456789012:function:myFunction:PROD",
"Name": "PROD"
},
{
"AliasArn": "arn:aws:lambda:us-west-2:123456789012:function:myFunction:DEV",
"Name": "DEV"
}
]
}
Use case 6: Display the reserved concurrency configuration for a function
Code:
aws lambda get-function-concurrency --function-name myFunction
Motivation: By examining reserved concurrency configurations, you can ensure that your function does not exceed specific invocation limits, providing a safeguard against excessive resource usage and cost overruns within your operational quotas.
Explanation:
aws lambda get-function-concurrency
: Command to retrieve concurrency settings of a Lambda function.--function-name myFunction
: The specified Lambda function whose concurrency settings are being queried.
Example output: Concurrency limits as configured for the function:
{
"ReservedConcurrentExecutions": 5
}
Use case 7: List which AWS services can invoke the function
Code:
aws lambda get-policy --function-name myFunction
Motivation: Understanding which AWS services possess the ability to invoke your Lambda function is integral to guaranteeing appropriate access controls and maintaining security measures. It assists in confirming that only the necessary services have invocation permissions.
Explanation:
aws lambda get-policy
: Command used to view the resource-based policy associated with a Lambda function.--function-name myFunction
: Identifies the particular function whose policy details are required.
Example output: The output showing services allowed to invoke the function:
{
"Policy": "{\"Version\":\"2012-10-17\",\"Statement\":[{\"Effect\":\"Allow\",\"Principal\":{\"Service\":\"s3.amazonaws.com\"},\"Action\":\"lambda:InvokeFunction\",\"Resource\":\"arn:aws:lambda:us-west-2:123456789012:function:myFunction\"}]}"
}
Conclusion
AWS Lambda functions can be managed and utilized with numerous commands that facilitate a variety of operational and configurational tasks. Whether you are invoking functions directly, understanding configurations, or managing access permissions and concurrency settings, these commands can dramatically enhance your control and efficiency in deploying and maintaining serverless applications.