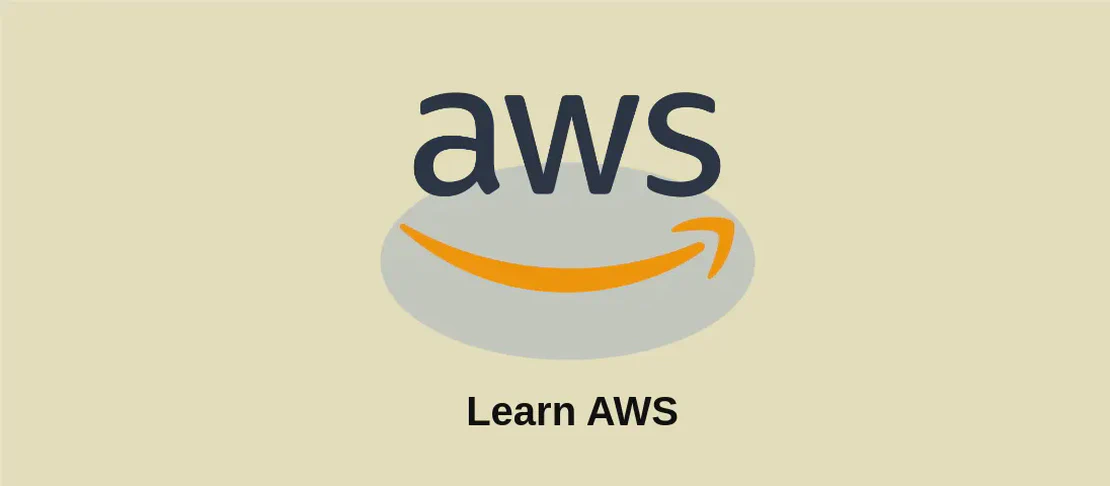
How to use the command 'aws route53' (with examples)
The AWS Route 53 CLI is a powerful tool for interfacing with Amazon’s scalable and highly available Domain Name System (DNS) web service. The command-line interface (CLI) allows administrators to manage DNS records, hosted zones, and health checks efficiently from the terminal. This enables seamless integration into automated workflows, supporting the dynamic needs of modern cloud-based architectures.
Use case 1: List all hosted zones, private and public
Code:
aws route53 list-hosted-zones
Motivation:
Listing all hosted zones is an essential task for administrators who need to manage and review their DNS configurations within AWS. It provides a holistic view of the public and private zones, allowing users to quickly identify all domains managed under their AWS account. This is particularly valuable in environments with multiple hosted zones, ensuring that administrators have a clear understanding of available resources.
Explanation:
aws route53
: This part of the command indicates the use of Amazon’s Route 53 service.list-hosted-zones
: This action within Route 53 requests a list of all the hosted zones associated with the AWS account.
Example output:
{
"HostedZones": [
{
"Id": "/hostedzone/Z1D633PJN98FT9",
"Name": "example.com.",
"Config": {
"PrivateZone": false
}
},
{
"Id": "/hostedzone/Z3AADJGX6KTTL2",
"Name": "example.private.",
"Config": {
"PrivateZone": true
}
}
]
}
Use case 2: Show all records in a zone
Code:
aws route53 list-resource-record-sets --hosted-zone-id zone_id
Motivation:
When managing a particular domain, it’s crucial to monitor and update DNS records efficiently. This command gives users the ability to access all records within a specific hosted zone. Such an overview is helpful for verifying existing configurations, finding misconfigured records, or preparing for updates, ensuring optimal DNS functionality and security.
Explanation:
aws route53
: Specifies the use of AWS Route 53 service.list-resource-record-sets
: Command to list all DNS records in a hosted zone.--hosted-zone-id zone_id
: Identifies which hosted zone to list the records. The zone_id is a unique identifier assigned by AWS.
Example output:
{
"ResourceRecordSets": [
{
"Name": "example.com.",
"Type": "A",
"TTL": 300,
"ResourceRecords": [
{
"Value": "192.0.2.44"
}
]
},
{
"Name": "www.example.com.",
"Type": "CNAME",
"TTL": 300,
"ResourceRecords": [
{
"Value": "example.com."
}
]
}
]
}
Use case 3: Create a new, public zone using a request identifier to retry the operation safely
Code:
aws route53 create-hosted-zone --name name --caller-reference request_identifier
Motivation:
Creating a new DNS hosted zone is a foundational step when setting up a new domain environment within AWS. By specifying a caller reference, users ensure that the operation can be safely retried if interrupted, preventing accidental duplications. This command ensures that a new public zone is seamlessly integrated into your infrastructure, ready for DNS record management.
Explanation:
aws route53
: Indicates the use of the AWS Route 53 service.create-hosted-zone
: Command to create a new hosted zone.--name name
: Specifies the domain name for the new hosted zone.--caller-reference request_identifier
: A unique string used to identify the request, allowing safe retries without creating duplicate zones in case of request lapses.
Example output:
{
"HostedZone": {
"Id": "/hostedzone/Z3M3LMPEXAMPLE",
"Name": "example.org.",
"CallerReference": "myReference001",
"Config": {
"PrivateZone": false
}
}
}
Use case 4: Delete a zone
Code:
aws route53 delete-hosted-zone --id zone_id
Motivation:
Managing DNS zones often requires the removal of outdated or unnecessary hosted zones to maintain a clean and organized configuration. Deleting zones is necessary when restructuring DNS setups, decommissioning services, or transferring domains to other providers. However, it’s important to ensure the zone is no longer in use and doesn’t have essential non-default SOA and NS records, as these will prevent deletion.
Explanation:
aws route53
: Designates the use of AWS Route 53.delete-hosted-zone
: Command triggers the deletion of a hosted zone.--id zone_id
: Specifies the unique identifier for the zone targeted for deletion.
Example output:
{
"ChangeInfo": {
"Id": "/change/C1PA6795EXAMPLE",
"Status": "PENDING",
"SubmittedAt": "2023-10-16T22:31:00.567Z"
}
}
Use case 5: Test DNS resolving by Amazon servers of a given zone
Code:
aws route53 test-dns-answer --hosted-zone-id zone_id --record-name name --record-type type
Motivation:
Testing DNS resolution using Amazon’s servers is crucial for ensuring that DNS records are correctly resolving as expected. This test enables administrators to verify the effectiveness of their DNS configurations without needing to change local DNS settings. It’s an invaluable tool for debugging and confirming changes before full deployment, ensuring that end-user experience is unaffected by DNS misconfigurations.
Explanation:
aws route53
: Points to AWS Route 53 service usage.test-dns-answer
: Command tests DNS resolution for a specified record.--hosted-zone-id zone_id
: Identifier for the hosted zone containing the record.--record-name name
: Specifies the DNS record name to be tested.--record-type type
: Indicates the record type (e.g., A, CNAME, MX) for the query.
Example output:
{
"Nameserver": "ns-2048.awsdns-64.net",
"RecordName": "www.example.com.",
"RecordType": "A",
"RecordData": [
"192.0.2.44"
],
"ResponseCode": "NOERROR",
"Protocol": "UDP"
}
Conclusion:
The AWS Route 53 CLI is an indispensable tool for managing DNS web services, boasting a range of functionalities critical for efficient domain management. From listing hosted zones to testing DNS resolution, each command usage detailed above provides distinct benefits that contribute to robust, scalable, and dynamic DNS management within the AWS ecosystem.