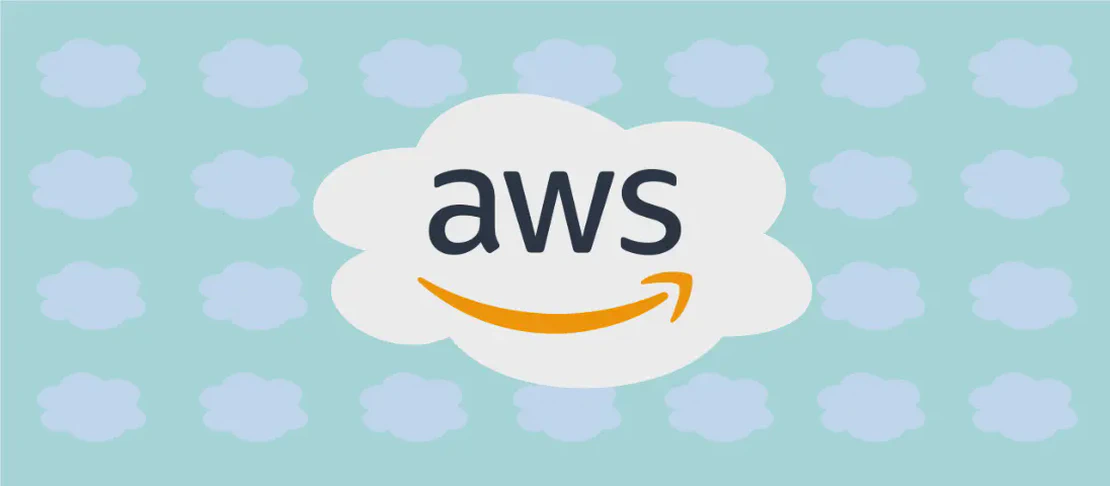
How to Use the Command 'aws s3 presign' (with Examples)
The AWS S3 CLI command aws s3 presign
is a powerful utility designed to generate pre-signed URLs for Amazon S3 objects, which allows temporary, secure access to private S3 resources without sharing AWS credentials. This is particularly useful when you want to share files with users who should not have direct access to your AWS resources but still need to view or download specific files. The command supports a variety of options to tailor the URL generation to specific access requirements, including setting a custom expiration time for the validity of the URL.
Use case 1: Generate a pre-signed URL for a specific S3 object that is valid for one hour
Code:
aws s3 presign s3://bucket_name/path/to/file
Motivation: There are scenarios when you need to share a file with a colleague or client temporarily. For instance, if you have a report stored in a private S3 bucket and you want to give someone access for them to download it without making it public, a pre-signed URL is the way to go. By default, the link will be valid for one hour, meaning that the recipient will have a limited time to use this URL, which enhances security by reducing the risk of unauthorized future access.
Explanation:
aws s3 presign
: This is the base command to initiate the creation of a pre-signed URL.s3://bucket_name/path/to/file
: This specifies the exact S3 object you are generating the URL for. It involves the bucket name where the object is stored and the path to the specific object within the bucket.
Example Output:
https://bucket_name.s3.amazonaws.com/path/to/file?AWSAccessKeyId=ASIAQEXAMPLE&Signature=h123abcdEXAMPLE&Expires=1511376262
This output is a URL allowing access to the specified S3 object. The URL is embedded with the necessary authentication parameters, granting the user temporary access to download the file.
Use case 2: Generate a pre-signed URL valid for a specific lifetime
Code:
aws s3 presign s3://bucket_name/path/to/file --expires-in duration_in_seconds
Motivation: Sometimes, one hour might not be the appropriate timeframe for granting file access. For example, if you are collaborating with a team that requires access over a weekend to review a project file, you might need to adjust the expiration to a longer period like 172800 seconds (which is two days). Specifying a precise validity period gives flexibility and control over how long the file should remain accessible via the generated URL.
Explanation:
aws s3 presign
: This part of the command remains the same as it is the base command to create the URL.s3://bucket_name/path/to/file
: Like the previous case, this indicates the specific file on S3.--expires-in duration_in_seconds
: This option allows you to define how long the pre-signed URL should remain valid. Here,duration_in_seconds
is replaced by an integer value representing the number of seconds the link should be active. This in-depth command customization enhances security management by granting time-sensitive access.
Example Output:
https://bucket_name.s3.amazonaws.com/path/to/file?AWSAccessKeyId=ASIAQEXAMPLE&Signature=h123abcdEXAMPLE&Expires=1511479862
This extended validity URL is appropriate for use cases requiring access periods varying from minutes to several days, depending on the expires-in
parameter specified.
Use case 3: Display help
Code:
aws s3 presign help
Motivation:
The aws s3 presign
command has various options and features that new users may not be fully aware of. By using the help command, users can explore the different functionalities without having to refer to external documentation. This can be particularly useful when troubleshooting or exploring new capabilities directly from the command line interface, boosting user efficiency and command comprehension.
Explanation:
aws s3 presign
: As before, this initiates the presign command.help
: This keyword triggers the display of help information, outlining the available options, detailed usages, and descriptions of what each option does. This ensures that users gain a complete understanding of how the command can be tailored to fit their particular needs.
Example Output:
NAME
s3 presign - Generate pre-signed URLs for Amazon S3 objects.
SYNOPSIS
aws s3 presign <S3Uri> [--expires-in <value>]
DESCRIPTION
The aws s3 presign command generates a pre-signed URL for an object with an optional
expiration time.
...
The output presents detailed instructions and available parameters, enabling users to effectively harness the command’s full capabilities.
Conclusion
Whether you are sharing a document with a coworker for a short period, setting a custom validity for extended access, or seeking guidance on the available command options, aws s3 presign
provides versatile solutions. It balances accessibility with security, enabling temporary, credential-free file sharing. Understanding how to use pre-signed URLs can optimize workflows, allowing controlled access to private S3 content.