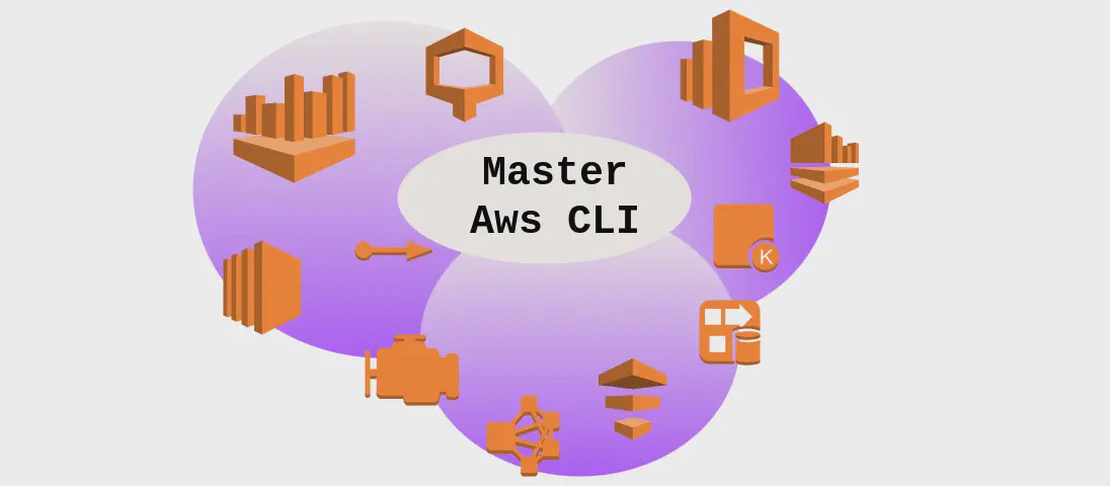
Creating and Managing Amazon S3 Buckets (with examples)
Create bucket in a specific region
Command:
aws s3api create-bucket --bucket bucket_name --region region --create-bucket-configuration LocationConstraint=region
Motivation:
Creating a bucket in a specific region allows the user to store their data in a region that is closest to their intended audience or where they expect to interact with the bucket the most. It helps optimize data transfer speed and reduce latency.
Explanation:
bucket_name
(required): The name of the bucket to be created.region
(required): The AWS Region code where the bucket should be created.LocationConstraint
(optional): Specifies the region where the bucket is created. This constraint is required when creating buckets in regions outside of the us-east-1 region.
Example Output:
{
"Location": "ARN"
}
Delete a bucket
Command:
aws s3api delete-bucket --bucket bucket_name
Motivation:
Deleting a bucket is useful when the bucket is no longer needed, or if you want to free up storage space and reduce costs by removing unused resources.
Explanation:
bucket_name
(required): The name of the bucket to be deleted.
Example Output:
No output is generated if the bucket is successfully deleted. If the bucket does not exist or fails to be deleted, an error message will be displayed.
List buckets
Command:
aws s3api list-buckets
Motivation:
Listing buckets allows you to quickly view all the buckets associated with your AWS account. This information can be useful for managing and organizing your S3 resources.
Explanation:
No arguments are required for this command.
Example Output:
{
"Buckets": [
{
"Name": "bucket1",
"CreationDate": "2021-01-01T00:00:00.000Z"
},
{
"Name": "bucket2",
"CreationDate": "2021-01-02T00:00:00.000Z"
}
]
}
List the objects inside of a bucket and only show each object’s key and size
Command:
aws s3api list-objects --bucket bucket_name --query 'Contents[].{Key: Key, Size: Size}'
Motivation:
Listing the objects inside a bucket helps to validate that the desired files or objects are present before performing any operations on them. It can also be useful for generating reports or summarizing the contents of a bucket.
Explanation:
bucket_name
(required): The name of the bucket from which to list the objects.--query
(required): A JMESPath query string to filter or format the response.
Example Output:
[
{
"Key": "object1.jpg",
"Size": 1024
},
{
"Key": "object2.jpg",
"Size": 2048
}
]
Add an object to a bucket
Command:
aws s3api put-object --bucket bucket_name --key object_key --body path/to/file
Motivation:
Adding an object to a bucket allows you to store files or data in S3. This is useful when uploading files for storage or when provisioning resources that require access to specific files.
Explanation:
bucket_name
(required): The name of the bucket where the object will be added.object_key
(required): The key or name of the object.path/to/file
(required): The path to the file on the local machine.
Example Output:
No output is generated if the object is successfully added. If there is an error, an appropriate error message will be displayed.
Download object from a bucket
Command:
aws s3api get-object --bucket bucket_name --key object_key path/to/output_file
Motivation:
Downloading an object from a bucket allows you to retrieve files or data that have been stored in S3. This is useful when you need to work with the files locally or transfer them to another location.
Explanation:
bucket_name
(required): The name of the bucket where the object is located.object_key
(required): The key or name of the object.path/to/output_file
(required): The path to save the downloaded file on the local machine.
Example Output:
No output is generated if the object is successfully downloaded. If there is an error, an appropriate error message will be displayed.
Apply an Amazon S3 bucket policy to a specified bucket
Command:
aws s3api put-bucket-policy --bucket bucket_name --policy file://path/to/bucket_policy.json
Motivation:
Applying a bucket policy allows you to control access to your S3 bucket and its contents. This is useful for setting fine-grained permissions and implementing security best practices.
Explanation:
bucket_name
(required): The name of the bucket to which the policy will be applied.file://path/to/bucket_policy.json
(required): The path to the JSON file containing the bucket policy.
Example Output:
No output is generated if the bucket policy is successfully applied. If there is an error, an appropriate error message will be displayed.
Download the Amazon S3 bucket policy from a specified bucket
Command:
aws s3api get-bucket-policy --bucket bucket_name --query Policy --output yaml > path/to/bucket_policy.yaml
Motivation:
Downloading the bucket policy allows you to review the current policy settings and make any necessary modifications. This is useful for auditing and maintaining the security and access control of your S3 bucket.
Explanation:
bucket_name
(required): The name of the bucket from which to download the policy.--query Policy
(required): Specifies the element of the response to download.--output yaml
(required): Specifies the output format of the downloaded policy.> path/to/bucket_policy.yaml
(required): Redirects the output to a file namedbucket_policy.yaml
.
Example Output:
A YAML file bucket_policy.yaml
will be created, containing the contents of the bucket policy.