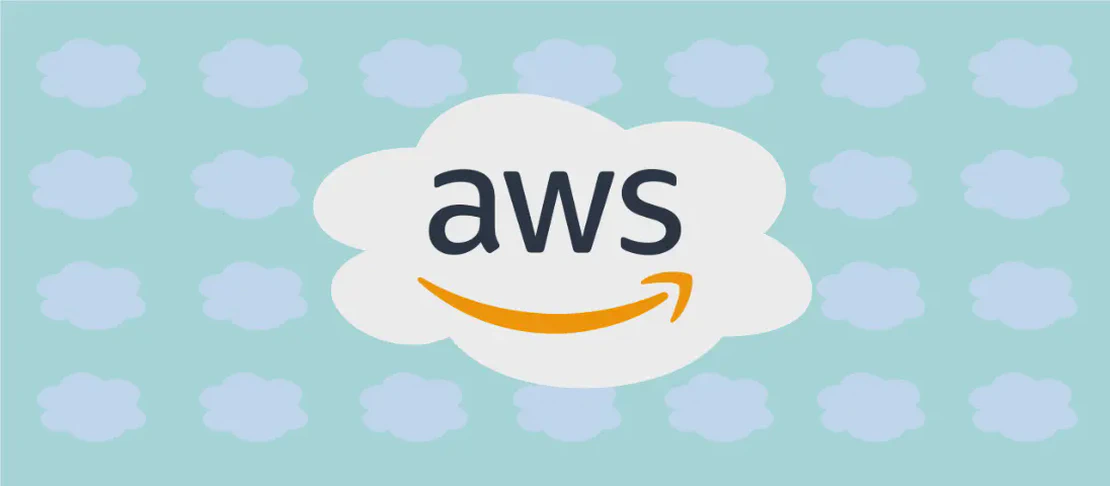
Mastering AWS Secrets Manager (with examples)
AWS Secrets Manager is a powerful service that lets users easily store, manage, and access secrets such as database credentials, API keys, and other sensitive information required by applications. It aids in maintaining compliance by rotating secrets automatically according to designated schedules and granting access only to authorized individuals. This ensures that sensitive data is securely managed with minimal manual intervention.
Use case 1: Show secrets stored by the secrets manager in the current account
Code:
aws secretsmanager list-secrets
Motivation:
Listing the secrets stored in AWS Secrets Manager is essential for inventory management and auditing purposes. By examining what secrets are available, administrators can ensure that all sensitive information is accounted for and that there are no redundant or unnecessary secrets stored, which could pose a security risk.
Explanation:
aws secretsmanager
: This part of the command specifies the AWS Secrets Manager service.list-secrets
: This subcommand is used to list all the secrets stored in the AWS account linked to the command-line interface.
Example Output:
The command will return a list of secrets, each with details like the secret’s name, ARN (Amazon Resource Name), and description.
[
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1",
"Name": "exampleSecret1",
"Description": "Database credentials for production"
},
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret2",
"Name": "exampleSecret2",
"Description": "API key for payment gateway"
}
]
Use case 2: List all secrets but only show the secret names and ARNs
Code:
aws secretsmanager list-secrets --query 'SecretList[*].{Name: Name, ARN: ARN}'
Motivation:
A filtered view focusing on the essential attributes such as secret names and ARNs helps to quickly ascertain the identities of secrets without wading through excessive details. This can be particularly helpful in extensive environments where the volume of secrets is large.
Explanation:
aws secretsmanager list-secrets
: Lists the secrets from the Secrets Manager.--query 'SecretList[*].{Name: Name, ARN: ARN}'
: This flag employs AWS’s JMESPath query language to filter and display only the list of secret names and ARNs, thus streamlining the output for convenience.
Example Output:
The output will contain only the names and ARNs of each secret, presented in a clean, easy-to-read format.
[
{
"Name": "exampleSecret1",
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1"
},
{
"Name": "exampleSecret2",
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret2"
}
]
Use case 3: Create a secret
Code:
aws secretsmanager create-secret --name exampleSecret3 --description "New API credentials" --secret-string 's3cr3t'
Motivation:
Creating a new secret is a fundamental operation within Secrets Manager. This use case is critical for securely storing new sensitive data that needs to be managed and accessed programmatically without exposing the values manually within codebases, thus enhancing security practices.
Explanation:
aws secretsmanager create-secret
: Indicates the command to add a new secret.--name exampleSecret3
: Assigns the name “exampleSecret3” to the new secret being created.--description "New API credentials"
: Provides a human-readable description to help identify the purpose of the secret.--secret-string 's3cr3t'
: Inserts the actual secret value,'s3cr3t'
, that needs to be securely stored.
Example Output:
Upon successful execution, the command will provide metadata about the newly created secret, including its ARN.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret3",
"Name": "exampleSecret3",
"VersionId": "EXAMPLE1-90ab-cdef-fedc-ba987EXAMPLE"
}
Use case 4: Delete a secret
Code:
aws secretsmanager delete-secret --secret-id exampleSecret3
Motivation:
Deleting a secret that is no longer in use reduces the risk of obsolete credentials being stolen or misused. This practice helps maintain an optimal security posture by ensuring that the stored secrets repository contains only current and needed information.
Explanation:
aws secretsmanager delete-secret
: The command to remove a specified secret.--secret-id exampleSecret3
: Specifies the name or ARN of the secret to be deleted. Here, the secret named “exampleSecret3” is chosen for deletion.
Example Output:
Execution of this command indicates that the specified secret has been marked for deletion and provides details like deletion date.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret3",
"Name": "exampleSecret3",
"DeletionDate": 1574371200.0
}
Use case 5: View details of a secret except for secret text
Code:
aws secretsmanager describe-secret --secret-id exampleSecret1
Motivation:
Understanding the metadata of a secret, such as its creation date, last accessed time, and tags, is helpful for management and compliance reporting. By not retrieving the secret text itself, exposure to sensitive data remains minimized.
Explanation:
aws secretsmanager describe-secret
: Provides information about the metadata associated with the secret.--secret-id exampleSecret1
: Identifies the secret whose details are to be fetched, specified by name or ARN.
Example Output:
This command returns metadata about the secret without exposing the actual secret text.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1",
"Name": "exampleSecret1",
"Description": "Database credentials for production",
"LastChangedDate": 1590451200.0,
"LastAccessedDate": 1593072000.0
}
Use case 6: Retrieve the value of a secret
Code:
aws secretsmanager get-secret-value --secret-id exampleSecret1
Motivation:
Retrieving the value of a secret is crucial for applications or services that require access to sensitive information for operation. Fetching the latest version ensures that applications are using up-to-date credentials, which supports security and functionality.
Explanation:
aws secretsmanager get-secret-value
: Command to fetch the actual current value stored in the secret.--secret-id exampleSecret1
: Specifies the unique identifier, either name or ARN, of the secret to retrieve.
Example Output:
The command will reveal the secret content alongside associated metadata. The actual secret text is encrypted but may display as plain text when decrypted on a secure client.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1",
"Name": "exampleSecret1",
"SecretString": "dbPassword123"
}
Use case 7: Rotate the secret immediately using a Lambda function
Code:
aws secretsmanager rotate-secret --secret-id exampleSecret1 --rotation-lambda-arn arn_of_lambda_function
Motivation:
Immediate rotation of a secret is a critical operation for responding to potential or confirmed credential leaks or breaches. The use of AWS Lambda ensures the process is automated and can be seamlessly integrated into the secret management lifecycle.
Explanation:
aws secretsmanager rotate-secret
: Initiates the rotation of the specified secret.--secret-id exampleSecret1
: Indicates which secret to rotate by name or ARN.--rotation-lambda-arn arn_of_lambda_function
: References the AWS Lambda function that will manage the rotation, responsible for updating the secret and relevant applications/users.
Example Output:
An immediate rotation action will return success confirmation and detail the ongoing process.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1",
"Name": "exampleSecret1",
"VersionId": "EXAMPLE2-90ab-cdef-fedc-ba987EXAMPLE"
}
Use case 8: Rotate the secret automatically every 30 days using a Lambda function
Code:
aws secretsmanager rotate-secret --secret-id exampleSecret1 --rotation-lambda-arn arn_of_lambda_function --rotation-rules AutomaticallyAfterDays=30
Motivation:
Setting automatic rotation intervals (e.g., every 30 days) ensures that secrets adhere to security policies and industry best practices without manual effort. Regular rotation reduces the risk of long-lived, potentially compromised credentials being exploited.
Explanation:
aws secretsmanager rotate-secret
: Plans the scheduled rotation of the specified secret.--secret-id exampleSecret1
: Targets the secret to schedule for rotation, specified by name or ARN.--rotation-lambda-arn arn_of_lambda_function
: Invokes a pre-configured AWS Lambda function that manages the secrets’ lifecycle during rotation.--rotation-rules AutomaticallyAfterDays=30
: Sets the interval in days (e.g., 30) after which the secret should be automatically rotated.
Example Output:
Scheduling the rotation will confirm the setup and detail the applied schedule, securing regular credential updates.
{
"ARN": "arn:aws:secretsmanager:region:account-id:secret:exampleSecret1",
"Name": "exampleSecret1",
"VersionId": "EXAMPLE3-90ab-cdef-fedc-ba987EXAMPLE",
"RotationEnabled": true,
"RotationInterval": "30 days"
}
Conclusion:
AWS Secrets Manager offers robust capabilities to manage sensitive information securely. Through these diverse use cases, from listing and creating secrets to implementing rotation strategies, organizations can enhance their secrets management processes and boost overall security postures seamlessly. These examples serve as a foundational guide toward leveraging AWS Secrets Manager effectively.