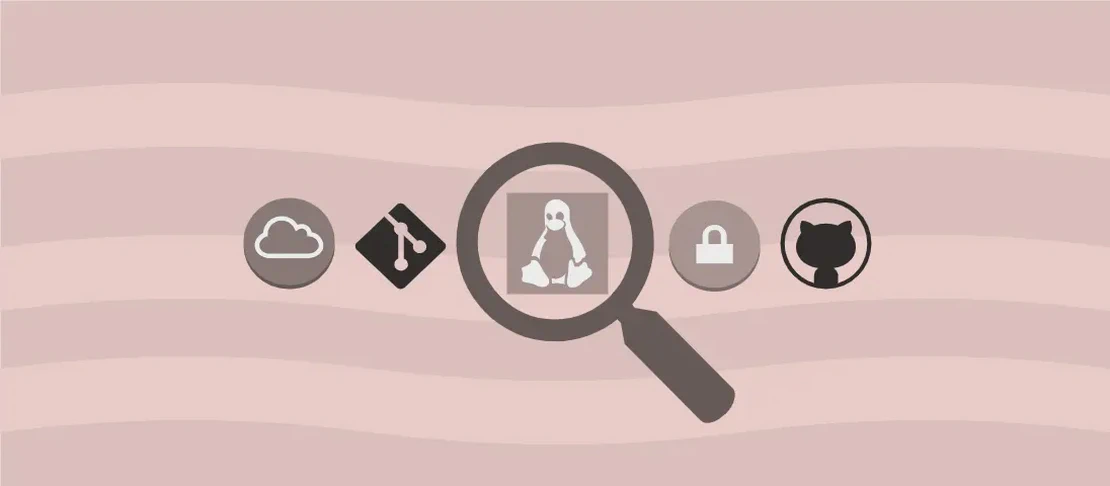
How to use the command 'aws sns' (with examples)
Amazon Simple Notification Service (SNS) is a flexible and highly-reliable cloud messaging service that allows you to send notifications from the cloud. You can configure SNS to send notifications as email, SMS, HTTP/S, and various other formats. Using the AWS Command Line Interface (CLI), you can easily interact with SNS to perform tasks such as creating topics, subscribing to topics, and managing subscriptions. This article explores various use cases related to the ‘aws sns’ command, providing real-world examples and detailed explanations for each.
Use case 1: List all objects of a specific type
Code:
aws sns list-topics
Motivation:
Listing all objects, such as topics or subscriptions, gives an overview of the current resources being managed in AWS SNS. It is especially useful in environments with a large number of topics or subscriptions, where keeping track manually can be cumbersome.
Explanation:
- The command
aws sns list-topics
is used to fetch a list of all topics. - No additional arguments are required for this command.
- This command helps manage and organize notification topics by providing their Amazon Resource Names (ARNs).
Example output:
{
"Topics": [
{
"TopicArn": "arn:aws:sns:us-west-2:123456789012:MyFirstTopic"
},
{
"TopicArn": "arn:aws:sns:us-west-2:123456789012:MySecondTopic"
}
]
}
Use case 2: Create a topic with a specific name and show its Amazon Resource Name (ARN)
Code:
aws sns create-topic --name MyNewTopic
Motivation:
Creating a new topic is the foundation of distributing messages in AWS SNS. Topics act as communication channels, and defining new topics allows organizations to categorize and distribute alerts effectively.
Explanation:
--name MyNewTopic
: Specifies the name of the new topic being created. This name should be unique within your AWS account and region.- The command returns the ARN of the newly created topic, which is necessary for subsequent operations like subscribing clients to the topic.
Example output:
{
"TopicArn": "arn:aws:sns:us-west-2:123456789012:MyNewTopic"
}
Use case 3: Subscribe an email address to the topic with a specific ARN and show the subscription ARN
Code:
aws sns subscribe --topic-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic --protocol email --notification-endpoint example@example.com
Motivation:
Subscribing an email address to a topic is vital for ensuring that messages published to that topic reach the relevant audience. This is especially useful for notification services, alert systems, and newsletters.
Explanation:
--topic-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic
: Specifies the ARN of the topic to which we are subscribing.--protocol email
: Indicates the communication protocol being used, which in this case is email.--notification-endpoint example@example.com
: The email address that will receive the notifications.
Example output:
{
"SubscriptionArn": "arn:aws:sns:us-west-2:123456789012:MyNewTopic:2bcfbf39-05c3-41de-beaa-fcfcc21c8f55"
}
Use case 4: Publish a message to a specific topic or phone number and show the message ID
Code:
aws sns publish --topic-arn "arn:aws:sns:us-west-2:123456789012:MyNewTopic" --message "Hello World!"
Motivation:
Publishing messages to a topic is the core functionality of SNS and is essential for distributing information efficiently. It is used in various scenarios like triggering alerts or sharing updates across teams.
Explanation:
--topic-arn "arn:aws:sns:us-west-2:123456789012:MyNewTopic"
: The ARN of the topic to which the message will be published.--message "Hello World!"
: The actual message content that will be sent to all subscribers of the topic.
Example output:
{
"MessageId": "d290f1ee-6c54-4b01-90e6-d701748f0851"
}
Use case 5: Delete the subscription with a specific ARN from its topic
Code:
aws sns unsubscribe --subscription-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic:2bcfbf39-05c3-41de-beaa-fcfcc21c8f55
Motivation:
Deleting a subscription is necessary when the subscriber no longer needs the information, such as when changing teams or roles or when a service is decommissioned. This keeps the notification system relevant and manageable.
Explanation:
--subscription-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic:2bcfbf39-05c3-41de-beaa-fcfcc21c8f55
: Specifies the unique ARN of the subscription that you want to delete.
Example output:
{
"Result": "Successfully unsubscribed"
}
Use case 6: Create a platform endpoint
Code:
aws sns create-platform-endpoint --platform-application-arn arn:aws:sns:us-west-2:123456789012:app/GCM/MyAndroidApp --token deviceTokenString
Motivation:
Creating a platform endpoint is critical for enabling push notifications to mobile devices. This is particularly useful for mobile application developers who need to send real-time updates to users.
Explanation:
--platform-application-arn arn:aws:sns:us-west-2:123456789012:app/GCM/MyAndroidApp
: The ARN of the platform application that will send notifications to a mobile device.--token deviceTokenString
: A unique identifier assigned to the device, which is necessary to send notifications.
Example output:
{
"EndpointArn": "arn:aws:sns:us-west-2:123456789012:endpoint/GCM/MyAndroidApp/ba4e9619-cd3f-3451-a961-3a5085eaabc9"
}
Use case 7: Add a statement to a topic’s access control policy
Code:
aws sns add-permission --topic-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic --label MyLabel --aws-account-id 123456789012 --action-name Publish
Motivation:
Adding permissions to a topic’s access control policy is essential for ensuring that the right users and applications can interact with your SNS topics. This helps maintain security and operational efficiency.
Explanation:
--topic-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic
: Specifies the ARN of the topic.--label MyLabel
: A unique label for identifying this permission statement.--aws-account-id 123456789012
: The AWS account ID to which permission is granted.--action-name Publish
: The action that is allowed; in this case, it is ‘Publish’.
Example output:
{
"Result": "Successfully added permission to the topic"
}
Use case 8: Add a tag to the topic with a specific ARN
Code:
aws sns tag-resource --resource-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic --tags Key=Environment,Value=Production
Motivation:
Tagging resources in AWS, such as SNS topics, is imperative for organization, billing, and managing resources through automation tools. Tags provide metadata that allows you to group, filter, and identify resources consistently.
Explanation:
--resource-arn arn:aws:sns:us-west-2:123456789012:MyNewTopic
: Specifies the ARN of the resource to tag.--tags Key=Environment,Value=Production
: Indicates the key-value pair for the tag, helping categorize and identify resources by their environment.
Example output:
{
"Result": "Successfully added tags to the resource"
}
Conclusion:
The ‘aws sns’ command in AWS CLI offers an extensive suite of features for managing notifications and communication channels in a cloud environment. By understanding these use cases, from listing topics to adding policy statements, AWS users can leverage SNS effectively to meet their messaging and notification needs. The examples provided in this article demonstrate the flexibility and power of AWS SNS, making it an indispensable tool in any cloud engineer’s toolkit.