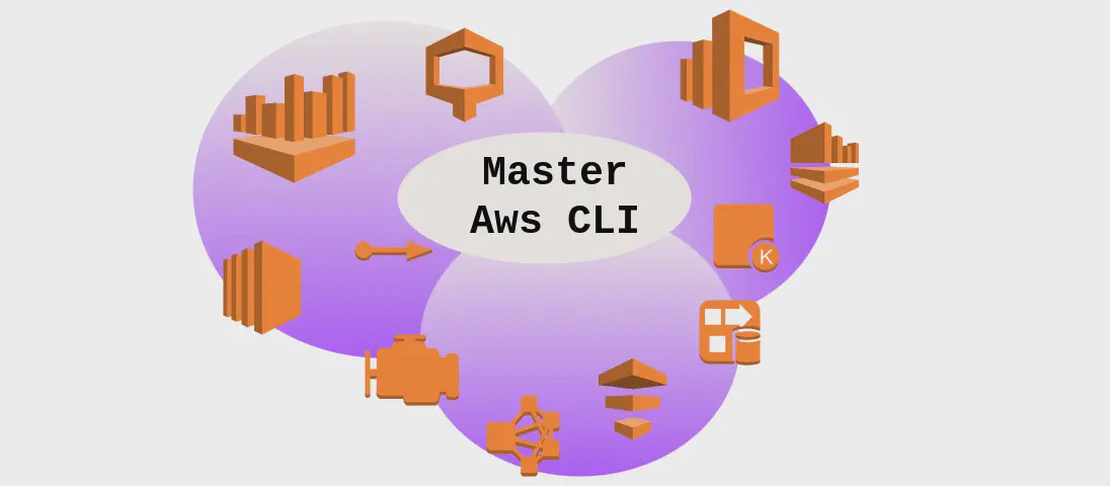
Mastering AWS SQS Commands (with examples)
AWS Simple Queue Service (SQS) is a fully managed message queuing service that allows you to decouple and scale microservices, distributed systems, and serverless applications. It enables seamless message integration within applications by providing buffered, queued, and reliabilities for message-driven architectures. Command-line interaction with AWS SQS can streamline queue management tasks, making automation and remote administration efficient and accessible. This article explores a range of use cases for AWS SQS command-line operations, complete with examples to guide you in practical implementations.
Use case 1: Listing All Available Queues
Code:
aws sqs list-queues
Motivation: Listing all available SQS queues is helpful when you manage multiple queues and need to ascertain which queues are active in your AWS environment. This command provides a quick overview, aiding in administration and troubleshooting tasks.
Explanation: The list-queues
command retrieves the URLs of all SQS queues associated with your AWS account in the region that you’ve configured in the AWS CLI. It doesn’t require any additional arguments, making it straightforward to use.
Example output:
{
"QueueUrls": [
"https://sqs.us-east-1.amazonaws.com/123456789012/Queue1",
"https://sqs.us-east-1.amazonaws.com/123456789012/Queue2"
]
}
Use case 2: Displaying the URL of a Specific Queue
Code:
aws sqs get-queue-url --queue-name myQueue
Motivation: Knowing the URL of a specific queue is essential when performing operations that require precise queue identification, such as sending messages or altering attributes.
Explanation:
get-queue-url
is the operation being performed.--queue-name
specifies the name of the queue for which you want to retrieve the URL. ReplacemyQueue
with the actual queue name.
Example output:
{
"QueueUrl": "https://sqs.us-east-1.amazonaws.com/123456789012/myQueue"
}
Use case 3: Creating a Queue with Specific Attributes from a JSON File
Code:
aws sqs create-queue --queue-name myNewQueue --attributes file://path/to/attributes_file.json
Motivation: When creating a new queue with specific configurations, using a JSON file allows you to define and reuse complex attribute sets, ensuring consistent configuration across multiple environments while saving time.
Explanation:
create-queue
is the operation to create a new queue.--queue-name
specifies the name of the new queue.--attributes
allows for specifying additional attributes for the queue. Usingfile://path/to/attributes_file.json
, you point to a JSON file containing key-value pairs of attributes.
Example output:
{
"QueueUrl": "https://sqs.us-east-1.amazonaws.com/123456789012/myNewQueue"
}
Use case 4: Sending a Specific Message to a Queue
Code:
aws sqs send-message --queue-url https://sqs.us-east-1.amazonaws.com/123456789012/myQueue --message-body "Hello, World" --delay-seconds 10 --message-attributes file://path/to/attributes_file.json
Motivation: Sending messages with attribute specifications and delays is crucial for controlling message flows in distributed systems. Delaying messages can be part of a strategy to manage asynchronous processing effectively.
Explanation:
send-message
is the operation to send a new message.--queue-url
designates the specific queue to which the message is sent.--message-body
is the content of the message.--delay-seconds
delays the message delivery.--message-attributes
provides additional metadata for the message, sourced from a JSON file.
Example output:
{
"MD5OfMessageBody": "5eb63bbbe01eeed093cb22bb8f5acdc3",
"MessageId": "e5a5eb01-2db2-4899-acad-69aa2c7f23f2"
}
Use case 5: Deleting the Specified Message from a Queue
Code:
aws sqs delete-message --queue-url https://sqs.us-east-1.amazonaws.com/123456789012/myQueue --receipt-handle myReceiptHandle
Motivation: Deleting messages from a queue after they have been processed ensures that they do not get reprocessed, maintaining data accuracy and efficiency in queue management.
Explanation:
delete-message
is the operation to remove a specific message.--queue-url
identifies the queue from which the message will be deleted.--receipt-handle
is the unique identifier of the message to be deleted, obtained when the message is received.
Example output:
The command does not return a JSON output for successful execution.
Use case 6: Deleting a Specific Queue
Code:
aws sqs delete-queue --queue-url https://sqs.us-east-1.amazonaws.com/123456789012/myQueue
Motivation: Deleting a queue when it is no longer needed helps optimize resource management and avoid unnecessary costs associated with maintaining idle queues.
Explanation:
delete-queue
is the operation to remove the specified queue.--queue-url
specifies the URL of the queue to be deleted.
Example output:
The command does not return a JSON output for successful execution.
Use case 7: Purging All Messages from the Specified Queue
Code:
aws sqs purge-queue --queue-url https://sqs.us-east-1.amazonaws.com/123456789012/myQueue
Motivation: Purging a queue is useful when you want to remove outdated or irrelevant messages without deleting the queue itself, enabling you to quickly reset the queue’s state.
Explanation:
purge-queue
is the operation to remove all messages.--queue-url
defines the specific queue to purge.
Example output:
The command does not return a JSON output for successful execution.
Use case 8: Enabling a Specific AWS Account to Send Messages to a Queue
Code:
aws sqs add-permission --queue-url https://sqs.us-east-1.amazonaws.com/123456789012/myQueue --label SendMessagePermission --aws-account-ids 987654321098 --actions SendMessage
Motivation: Granting permission to another AWS account to send messages can facilitate cross-account communication and collaboration through SQS, broadening integration possibilities.
Explanation:
add-permission
command allows modifications to a queue’s policy.--queue-url
identifies the target queue.--label
provides a unique name for permission identification.--aws-account-ids
specifies the account granted the permission.--actions
determines the permissions granted, such asSendMessage
.
Example output:
The command does not return a JSON output for successful execution.
Conclusion:
AWS SQS commands via the AWS CLI provide powerful capabilities to manage message queues effectively. The examples provided in this article illustrate various key operations that are essential for building and maintaining robust message-driven architectures. By mastering these commands, you can enhance automation, improve system interactions, and maintain efficient resource management within your AWS environments.