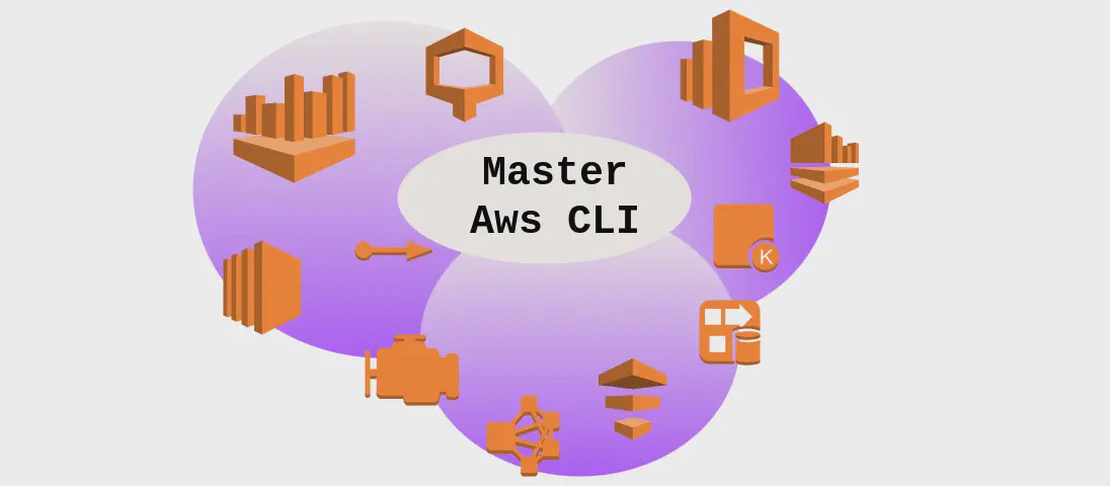
How to use the command 'aws sts' (with examples)
The AWS Security Token Service (STS) is a global service provided by Amazon Web Services that allows clients to request temporary, limited-privilege credentials for users or workloads. These credentials are used to securely access AWS resources without needing long-term AWS access credentials. This service is commonly employed to enhance security by creating short-lived credentials that limit the duration of user sessions.
In this article, we will explore two common use cases for the aws sts
command, showcasing how to obtain temporary security credentials and how to identify the AWS account or IAM user being used to make the API calls.
Use Case 1: Get Temporary Security Credentials
Code:
aws sts assume-role --role-arn arn:aws:iam::123456789012:role/example-role --role-session-name MySession
Motivation:
Imagine a scenario where you have an application that runs on an EC2 instance, and this application needs access to certain AWS resources such as an S3 bucket or an RDS instance. Instead of hardcoding AWS credentials in the application, which can pose a security risk, you can use the assume-role
API to get temporary security credentials. This allows your application to interact with the necessary AWS resources in a secure manner.
Explanation:
assume-role
: This is the API call requesting temporary security credentials. It allows you to act as a different role to carry out the necessary tasks.--role-arn arn:aws:iam::123456789012:role/example-role
: This argument specifies the Amazon Resource Name (ARN) of the role that you want to assume. The ARN uniquely identifies the role within the whole AWS eco-system.--role-session-name MySession
: This is an identifier for the assumed role session. It allows you to distinguish between different sessions. A session name can be helpful in the CloudTrail logs and helps with troubleshooting issues related to role assumption.
Example Output:
{
"Credentials": {
"AccessKeyId": "ASIAIOSFODNN7EXAMPLE",
"SecretAccessKey": "wJalrXUtnFEMI/K7MDENG/bPxRfiCYzEXAMPLEKEY",
"SessionToken": "AQoDYXdzEJr...<remainder of security token>",
"Expiration": "2023-01-01T12:00:00Z"
},
"AssumedRoleUser": {
"AssumedRoleId": "AROACLKWSDQRAOEXAMPLE:MySession",
"Arn": "arn:aws:sts::123456789012:assumed-role/example-role/MySession"
}
}
In this output, you receive temporary credentials that include an access key, a secret access key, and a session token, all tied to the specified role and session. These credentials are valid for a specified duration and end at the expiration time.
Use Case 2: Get IAM User or Role Identity
Code:
aws sts get-caller-identity
Motivation:
For developers and administrators, it’s essential to know which IAM user or role is executing a command. This is particularly useful in scripts or applications running with credentials that are unclear. By using the get-caller-identity
API, you can quickly determine and verify the identity of the caller, helping you debug or audit access permissions in complex environments.
Explanation:
get-caller-identity
: This command retrieves details about the IAM user or role whose credentials are being used to execute a command. It returns the AWS account ID, the ARN of the caller, and the user ID, which uniquely identifies the identity.
Example Output:
{
"UserId": "AROAEXAMPLEID:IAMUser",
"Account": "123456789012",
"Arn": "arn:aws:iam::123456789012:user/IAMUser"
}
In this response, you see the UserId
, which is the identifier associated with the IAM identity, the Account
number which shows the AWS account ID, and the Arn
, which denotes the Amazon Resource Name, indicating the specific IAM user or role making the call.
Conclusion:
The aws sts
command is a powerful tool for managing access to AWS resources securely and efficiently. By using these commands, you can leverage temporary credentials, improve security posture, and better understand the identities interacting with AWS services. Whether you are an application developer or an AWS administrator, familiarizing yourself with these use cases can enhance your AWS resource management strategies incrementally.