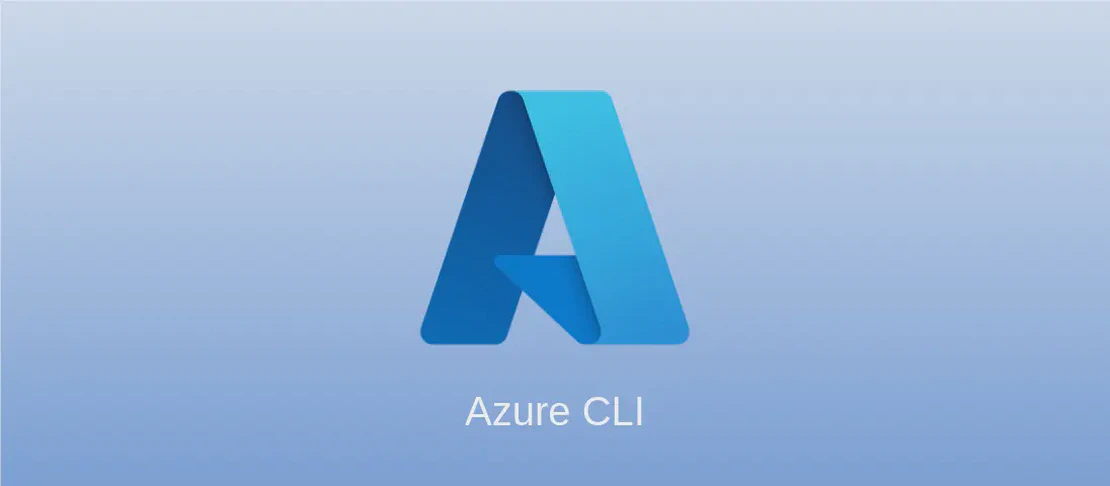
How to Use the Command 'az acr' (with examples)
The Azure Container Registry (ACR) is a managed, private Docker registry service provided by Microsoft Azure. It allows developers to store and manage container images in a private registry, eliminating the hassle of managing and scaling private Docker registries. The az acr
command is a part of the Azure Command-Line Interface (CLI), frequently referred to as az
. It provides numerous capabilities to manage and interact with Azure Container Registries, including creating registries, managing images, and more. Below, we explore various use cases of the az acr
command with detailed examples.
Use case 1: Create a Managed Container Registry
Code:
az acr create --name myRegistry --resource-group myResourceGroup --sku Basic
Motivation:
Creating a managed container registry using Azure ensures that your Docker images are safely stored in a scalable and secure manner. Azure takes care of scaling the registry service as needed, while you remain focused on building containerized solutions.
Explanation:
az acr create
: Initiates the process of creating a new Azure Container Registry.--name myRegistry
: Specifies the unique name for your registry within Azure.--resource-group myResourceGroup
: Indicates the Azure resource group to which the registry belongs. Resource groups help users manage and organize related Azure resources.--sku Basic
: Defines the pricing tier for your registry. Different SKUs offer varying levels of performance and features, from Basic to Premium.
Example Output:
{
"adminUserEnabled": false,
"creationDate": "2023-04-01T12:34:56.789123Z",
"id": "/subscriptions/.../resourceGroups/myResourceGroup/providers/Microsoft.ContainerRegistry/registries/myRegistry",
"location": "eastus",
"name": "myRegistry",
"resourceGroup": "myResourceGroup",
"sku": {
"name": "Basic",
"tier": "Basic"
},
"status": "Succeeded"
}
Use case 2: Login to a Registry
Code:
az acr login --name myRegistry
Motivation:
Logging into your Azure Container Registry allows Docker to interact with it. Once authenticated, you can push or pull images directly to and from the registry, enabling seamless integration into your container deployment pipeline.
Explanation:
az acr login
: Initiates the login process for the specified Azure Container Registry.--name myRegistry
: Specifies the name of the Azure Container Registry to which you want to log in.
Example Output:
Login Succeeded
Use case 3: Tag a Local Image for ACR
Code:
docker tag myApp myRegistry.azurecr.io/myApp:v1
Motivation:
Tagging Docker images is an essential practice. It allows developers to version images and identify them easily. By tagging an image with a specific ACR URL, you prepare it for seamless upload to Azure Container Registry.
Explanation:
docker tag
: A Docker command used to tag an existing local Docker image.myApp
: The local name of the Docker image you wish to tag.myRegistry.azurecr.io/myApp:v1
: Defines the new name and tag for the image, including the registry URL. “v1” represents the version tag.
Example Output:
Upon running this command, you won’t see an output, but the image will be tagged and ready for pushing to your ACR.
Use case 4: Push an Image to a Registry
Code:
docker push myRegistry.azurecr.io/myApp:v1
Motivation:
Pushing images to a registry ensures they are stored in a centralized, accessible location, making them available for deployment across different environments. It’s crucial for distribution and version control.
Explanation:
docker push
: Initiates the process of uploading an image to a specified registry.myRegistry.azurecr.io/myApp:v1
: Specifies the fully qualified image name that includes the registry URL and tag.
Example Output:
The push refers to repository [myRegistry.azurecr.io/myApp]
...
v1: digest: sha256:... size: ...
Use case 5: Pull an Image from a Registry
Code:
docker pull myRegistry.azurecr.io/myApp:v1
Motivation:
Pulling an image from ACR allows you to retrieve a container image onto your local machine for development, testing, or deployment. It helps maintain consistency and traceability across different environments.
Explanation:
docker pull
: Initiates the download of an image from a Docker registry.myRegistry.azurecr.io/myApp:v1
: Specifies the complete name and tag of the image you wish to pull.
Example Output:
v1: Pulling from myApp
...
Status: Downloaded newer image for myRegistry.azurecr.io/myApp:v1
Use case 6: Delete an Image from a Registry
Code:
az acr repository delete --name myRegistry --repository myApp:v1
Motivation:
Deleting images that are no longer needed helps manage storage costs and maintain a clean registry. Removing outdated or unused versions improves clarity and organization.
Explanation:
az acr repository delete
: Initiates the deletion process for a specified image in the registry.--name myRegistry
: Indicates the registry from which the image will be deleted.--repository myApp:v1
: Specifies the name and tag of the image to be removed.
Example Output:
{
"deleted": [
"myApp:v1"
]
}
Use case 7: Delete a Managed Container Registry
Code:
az acr delete --name myRegistry --resource-group myResourceGroup --yes
Motivation:
When a registry is no longer needed, deleting it can free up resources and prevent cost accrual. This is a crucial step in resource clean-up and management.
Explanation:
az acr delete
: Initiates deletion of a specified Azure Container Registry.--name myRegistry
: Defines which registry to delete.--resource-group myResourceGroup
: Ensures the correct resource group containing the registry is specified.--yes
: Automatically confirms and processes the deletion without additional prompts.
Example Output:
{
"status": "Succeeded"
}
Use case 8: List Images Within a Registry
Code:
az acr repository list --name myRegistry --output table
Motivation:
Listing images gives a comprehensive view of contents within your registry, helping track available image versions and organizing resource management effectively.
Explanation:
az acr repository list
: Initiates a command to return the list of repositories/images within a specified registry.--name myRegistry
: Specifies the registry you want to inspect.--output table
: Formats the output in an easy-to-read table for concise review.
Example Output:
Name
----
myApp
anotherApp
sampleApp
...
Conclusion:
The az acr
command provides a powerful set of features for managing Azure Container Registries, facilitating every step from creation to image management. By integrating these tools into your workflows, you can enhance efficiency and security for containerized applications.