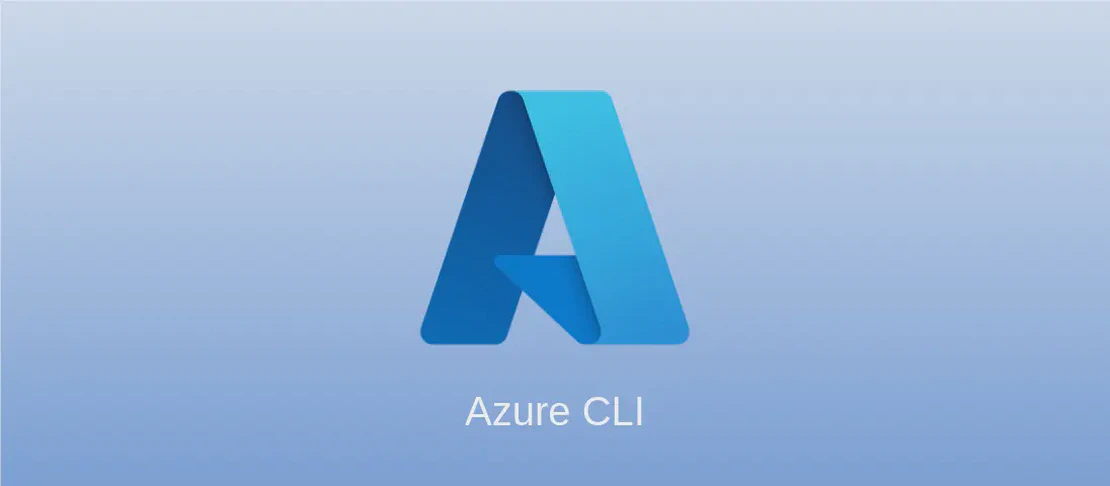
How to Manage Azure Kubernetes Service Clusters using 'az aks' (with examples)
The az aks
command is a part of the Azure Command-Line Interface (CLI) focused on managing Azure Kubernetes Service (AKS) clusters. These clusters are essential for deploying, managing, and scaling containerized applications using Kubernetes on Azure. The az aks
suite provides a variety of options to create, manage, and configure these AKS clusters, allowing developers and system administrators to utilize the power of Kubernetes with the ease of Azure’s cloud platform. Below are several use cases highlighting the versatile utility of the az aks
command.
Use case 1: List AKS Clusters
Code:
az aks list --resource-group resource_group
Motivation:
Listing AKS clusters is crucial for gaining an overview of all running clusters within a specific resource group. This is particularly helpful for administrators managing multiple Kubernetes clusters as it allows them to quickly see what clusters are available, assess their status, and make decisions about further management actions.
Explanation:
az aks list
: Thelist
operation is the primary command to retrieve all AKS clusters.--resource-group resource_group
: This argument specifies the Azure resource group containing the AKS clusters. Resource groups are collections of resources used in Azure services, acting as a container to aggregate resources together.
Example Output:
You may see information similar to this, which includes details about each cluster such as their name, region, and status.
[
{
"name": "MyAKSCluster",
"location": "eastus",
"provisioningState": "Succeeded",
...
}
]
Use case 2: Create a New AKS Cluster
Code:
az aks create --resource-group resource_group --name name --node-count count --node-vm-size size
Motivation:
Creating a new AKS cluster is often the first step in setting up a Kubernetes environment on Azure. This use case allows developers and businesses to deploy containerized applications with seamless scalability and management features provided by Azure.
Explanation:
az aks create
: Thecreate
command initializes a new Kubernetes cluster.--resource-group resource_group
: Denotes the desired resource group where the new cluster should be created.--name name
: Assigns a unique identifier to the new AKS cluster.--node-count count
: Specifies the number of nodes or virtual machines that will be part of the AKS cluster. More nodes equate to better load handling but incur increased costs.--node-vm-size size
: Defines the virtual machine size for the nodes in the cluster. Size affects the compute capabilities and pricing of each node.
Example Output:
A successful cluster creation will yield output containing the new cluster’s details, including its ID, DNS name, and configuration specifications.
{
"id": "/subscriptions/.../resourceGroups/.../providers/Microsoft.ContainerService/managedClusters/MyAKSCluster",
"location": "eastus",
"name": "MyAKSCluster",
"agentPoolProfiles": [
{
"count": 3,
"vmSize": "Standard_DS2_v2",
...
}
],
"fqdn": "myakscluster-dns-xyz.hcp.eastus.azmk8s.io",
...
}
Use case 3: Delete an AKS Cluster
Code:
az aks delete --resource-group resource_group --name name
Motivation:
Deleting an AKS cluster is necessary when a cluster is no longer needed. This can help reduce unnecessary costs and free up resources in the cloud environment. It is especially important for housekeeping, managing costs, and avoiding clutter in your Azure account.
Explanation:
az aks delete
: Thedelete
command removes an existing AKS cluster.--resource-group resource_group
: Indicates the resource group where the target AKS cluster resides.--name name
: Specifies the name of the AKS cluster to be deleted.
Example Output:
Running this command will typically require confirmation before deletion, and the output will confirm the operation’s completion.
Are you sure you want to perform this operation? (y/n): y
- Deleting ...
Use case 4: Get the Access Credentials for an AKS Cluster
Code:
az aks get-credentials --resource-group resource_group --name name
Motivation:
Acquiring access credentials for an AKS cluster is essential for interacting with it using Kubernetes tools like kubectl
. Access to these credentials enables developers and operations teams to manage and deploy applications onto the cluster seamlessly.
Explanation:
az aks get-credentials
: This operation retrieves the necessary authentication details to interact with the cluster.--resource-group resource_group
: Specifies the resource group containing the AKS cluster.--name name
: Identifies the cluster for which access credentials are being fetched.
Example Output:
Upon successful completion, the command will merge the AKS cluster’s credentials into your local kubeconfig
file, allowing secure interaction.
Merged "MyAKSCluster" as current context in /Users/username/.kube/config
Use case 5: Get the Upgrade Versions Available for an AKS Cluster
Code:
az aks get-upgrades --resource-group resource_group --name name
Motivation:
Checking available upgrade versions for an AKS cluster is vital for maintaining security, performance, and access to new Kubernetes features. This ensures that the cluster is running on supported versions and enables planning for future updates.
Explanation:
az aks get-upgrades
: Lists all available Kubernetes versions that the cluster can be upgraded to.--resource-group resource_group
: Points to the resource group encompassing the AKS cluster.--name name
: Specifies the AKS cluster for which upgrade information is requested.
Example Output:
Reviewing this output helps administrators decide on upgrading practices based on available changes.
{
"agentPoolProfiles": [
{
"name": "nodepool1",
"osType": "Linux",
"kubernetesVersion": "1.18.14",
"upgrades": [
{
"kubernetesVersion": "1.19.7"
},
{
"kubernetesVersion": "1.20.5"
}
],
...
}
]
}
Conclusion:
The az aks
command provides a powerful means to manage AKS clusters on Microsoft Azure. By understanding and utilizing these use cases, cloud users can effectively deploy, maintain, and optimize their Kubernetes environments for both robust applications and efficient IT operations. Whether listing clusters, creating new ones, accessing them, or ensuring they’re up-to-date, az aks
is instrumental in harnessing the potential of Azure’s managed Kubernetes service.