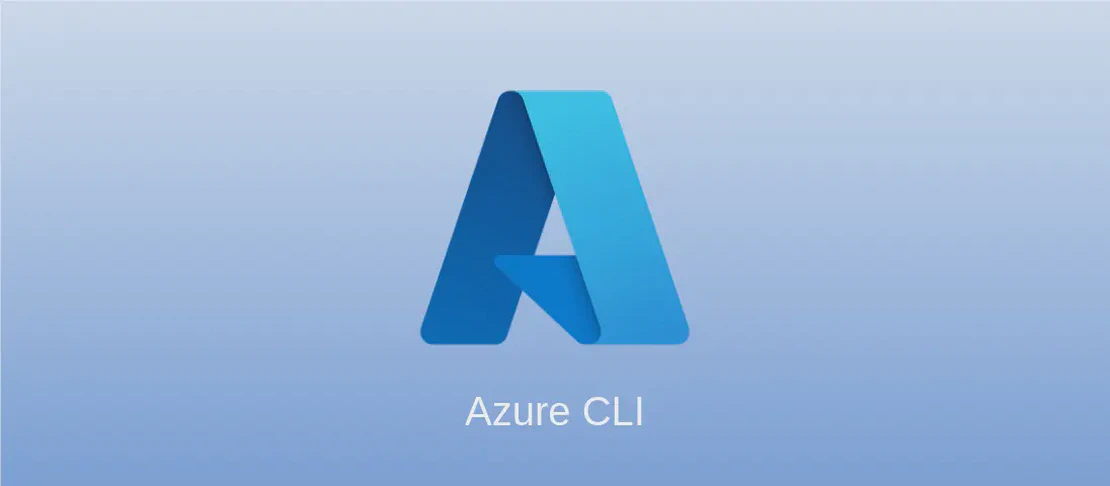
Managing Azure API Management Services Using 'az apim' (with examples)
The Azure API Management (APIM) service is a crucial component for organizations looking to manage their APIs efficiently. It allows teams to publish, secure, transform, maintain, and monitor APIs all from one centralized location. The az apim
command, part of the Azure Command-Line Interface (CLI), provides powerful options to manage API Management instances directly from your terminal. Whether you’re creating a new service instance, updating settings, or reviewing what instances you have, az apim
offers straightforward commands to accomplish these tasks.
Use case 1: List API Management services within a resource group
Code:
az apim list --resource-group myResourceGroup
Motivation:
Before making changes or additions to API Management services, it’s often necessary to get a comprehensive list of what services currently exist within a specific resource group. This command provides a clear view of existing services and assists in planning future configurations or actions.
Explanation:
az apim list
: This is the base command used to list all API Management service instances.--resource-group myResourceGroup
: This flag specifies the Azure resource group of interest. A resource group is a logical container for Azure resources, allowing for management and organization of services within Azure.
Example output:
[
{
"name": "myApiManagement",
"resourceGroup": "myResourceGroup",
"location": "East US",
"sku": { "name": "Developer", "capacity": 1 }
}
]
Use case 2: Create an API Management service instance
Code:
az apim create --name myApiManagement --resource-group myResourceGroup --publisher-email admin@example.com --publisher-name MyAdminName
Motivation:
Creating an API Management service is a foundational task when establishing a new API strategy. This command sets up a bespoke environment tailored to manage numerous APIs under one service instance, providing the necessary framework for secure and reliable API consumption.
Explanation:
az apim create
: Initiates the creation of a new API Management service instance.--name myApiManagement
: Assigns a name to the API Management instance being created. This name will be how the instance is identified within Azure.--resource-group myResourceGroup
: Specifies the resource group where the new service will reside.--publisher-email admin@example.com
: Sets the email address for the API publisher, essential for administrative messages and communication regarding the instance.--publisher-name MyAdminName
: Designates the name of the individual or organization responsible for the management of the API.
Example output:
A successful command execution would end with output similar to the following, confirming the service creation:
{
"name": "myApiManagement",
"resourceGroup": "myResourceGroup",
"location": "East US",
"sku": { "name": "Developer", "capacity": 1 },
"publisherEmail": "admin@example.com",
"publisherName": "MyAdminName"
}
Use case 3: Delete an API Management service
Code:
az apim delete --name myApiManagement --resource-group myResourceGroup
Motivation:
As API strategies evolve, certain service instances might become obsolete, requiring decommissioning. Deleting an outdated or no longer needed API Management service helps optimize costs and maintain a clutter-free cloud environment.
Explanation:
az apim delete
: The primary command to remove an API Management service instance.--name myApiManagement
: Identifies the specific API Management instance to delete.--resource-group myResourceGroup
: Specifies which resource group contains the instance targeted for deletion.
Example output:
Typically, this command will not return an output but will remove the API Management instance silently upon successful execution.
Use case 4: Show details of an API Management service instance
Code:
az apim show --name myApiManagement --resource-group myResourceGroup
Motivation:
Accurate information about an API Management instance is critical for audits, troubleshooting, or when planning further infrastructure deployments. This command fetches detailed metrics and settings associated with a particular service instance.
Explanation:
az apim show
: Command used to display comprehensive details of a specified API Management service instance.--name myApiManagement
: The name of the API Management service for which you want to retrieve details.--resource-group myResourceGroup
: The resource group the specified instance belongs to.
Example output:
A command execution will yield detailed information about the instance in JSON format:
{
"name": "myApiManagement",
"resourceGroup": "myResourceGroup",
"location": "East US",
"sku": { "name": "Developer", "capacity": 1 },
"provisioningState": "Succeeded",
"publisherEmail": "admin@example.com",
"publisherName": "MyAdminName"
}
Use case 5: Update an API Management service instance
Code:
az apim update --name myApiManagement --resource-group myResourceGroup --set publisher-email=newemail@example.com
Motivation:
Over time, configurations or administrative details for API Management may need adjustments to align with organizational changes or improvements in operations. This command allows for updating these settings without needing to recreate the service from scratch.
Explanation:
az apim update
: This keyword signifies that the API Management instance is being updated.--name myApiManagement
: Identifies which service instance to update.--resource-group myResourceGroup
: Indicates the resource group where the service instance resides.--set publisher-email=newemail@example.com
: Specifies the new value for the publisher email address.
Example output:
Output after this successful command execution will indicate the updated settings, often without additional verbose or structured output unless errors occur.
Conclusion:
Mastering the az apim
commands enhances your ability to efficiently manage Azure API Management services, simplifying complex tasks through a versatile command-line approach. Whether listing, creating, updating, consulting, or deleting services, these commands empower users to adapt their API infrastructure seamlessly.