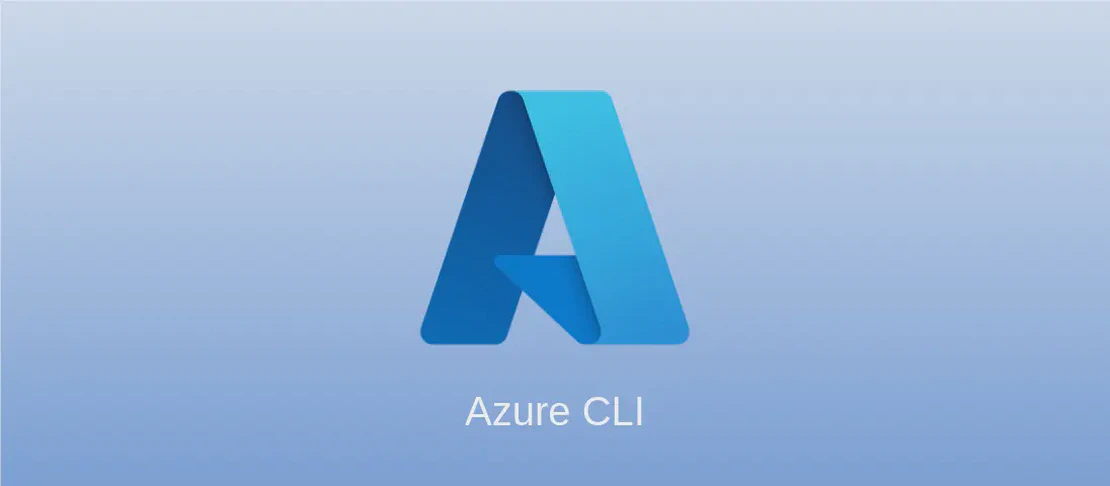
Managing Azure App Configurations with 'az appconfig' (with examples)
Azure App Configuration is a service that provides a centralized location to manage and secure application settings. The command-line utility az appconfig
is part of Azure CLI, designed to facilitate the management of your app configurations on Azure. Whether you are setting up a new configuration or maintaining existing ones, az appconfig
provides a robust set of commands to efficiently handle these tasks.
Use case 1: Create an App Configuration
Code:
az appconfig create --name myAppConfig --resource-group myResourceGroup --location eastus
Motivation:
Creating an Azure App Configuration is a foundational step when setting up an application’s environment. It allows developers to manage application settings in a centralized and secure manner. When building a cloud-native application, establishing an App Configuration ensures that configuration data is distributed and updated efficiently across various application components.
Explanation:
az appconfig create
: Initializes the creation of a new App Configuration service.--name myAppConfig
: Specifies the name for the new App Configuration you are creating.--resource-group myResourceGroup
: Indicates the resource group under which the new App Configuration will be organized. Resource groups help in managing and grouping related Azure resources.--location eastus
: Designates the geographical region where the service will reside. This can affect both latency and availability.
Example Output:
{
"id": "/subscriptions/xxxxxxx/resourceGroups/myResourceGroup/providers/Microsoft.AppConfiguration/configurationStores/myAppConfig",
"location": "eastus",
"name": "myAppConfig",
"provisioningState": "Succeeded",
"resourceGroup": "myResourceGroup",
"sku": {
"name": "Standard"
}
}
Use case 2: Delete a specific App Configuration
Code:
az appconfig delete --resource-group myResourceGroup --name myAppConfig
Motivation:
Over time, as the application landscape evolves, certain configurations might become obsolete or get replaced. Deleting an unused or unnecessary App Configuration is crucial for maintaining a tidy and organized Azure environment. This action not only simplifies management but can also help in optimizing resource usage.
Explanation:
az appconfig delete
: Initiates the deletion of an existing App Configuration.--resource-group myResourceGroup
: Specifies the resource group of the App Configuration that you wish to remove.--name myAppConfig
: Identifies the specific configuration store to delete by name.
Example Output:
The App Configuration 'myAppConfig' has been successfully deleted.
Use case 3: List all App Configurations under the current subscription
Code:
az appconfig list
Motivation:
When managing numerous applications or services, it is advantageous to have a quick overview of all available App Configurations within a subscription. This can be especially useful for audits, ensuring uniformity, or simply getting an inventory of configurations being used.
Explanation:
az appconfig list
: Retrieves a list of all App Configuration instances under the current Azure subscription. It does not require additional parameters, as it defaults to the subscription in use.
Example Output:
[
{
"id": "/subscriptions/xxxxxxx/resourceGroups/group1/providers/Microsoft.AppConfiguration/configurationStores/appConfig1",
"location": "westus",
"name": "appConfig1",
"resourceGroup": "group1",
},
{
"id": "/subscriptions/xxxxxxx/resourceGroups/group2/providers/Microsoft.AppConfiguration/configurationStores/appConfig2",
"location": "eastus",
"name": "appConfig2",
"resourceGroup": "group2",
}
]
Use case 4: List all App Configurations under a specific resource group
Code:
az appconfig list --resource-group myResourceGroup
Motivation:
Sometimes, you need to focus on a particular segment of your application suite, which is neatly arranged into separate resource groups. Listing only the App Configurations pertaining to a specific resource group allows more targeted management and analysis.
Explanation:
az appconfig list
: The command used for listing configurations.--resource-group myResourceGroup
: Filters the list to only show App Configurations that belong tomyResourceGroup
.
Example Output:
[
{
"id": "/subscriptions/xxxxxxx/resourceGroups/myResourceGroup/providers/Microsoft.AppConfiguration/configurationStores/appConfig1",
"location": "eastus",
"name": "appConfig1",
"resourceGroup": "myResourceGroup",
}
]
Use case 5: Show properties of an App Configuration
Code:
az appconfig show --name myAppConfig
Motivation:
Understanding the specific settings and properties of an App Configuration can be necessary when troubleshooting or configuring applications that depend on those settings. Displaying its properties provides valuable insights into its structure and current configuration state.
Explanation:
az appconfig show
: Retrieves details of a specific App Configuration.--name myAppConfig
: Points to the particular configuration store whose details are needed.
Example Output:
{
"id": "/subscriptions/xxxxxxx/resourceGroups/myResourceGroup/providers/Microsoft.AppConfiguration/configurationStores/myAppConfig",
"location": "eastus",
"name": "myAppConfig",
"resourceGroup": "myResourceGroup",
"provisioningState": "Succeeded",
"tags":{},
"sku": {
"name": "Standard"
}
}
Use case 6: Update a specific App Configuration
Code:
az appconfig update --resource-group myResourceGroup --name myAppConfig
Motivation:
Configurations often need to be updated to reflect changes in application requirements or enhance security and performance. Updating an App Configuration is a regular maintenance task that ensures your applications run smoothly with the latest settings applicable.
Explanation:
az appconfig update
: Begins the update of an existing App Configuration’s properties.--resource-group myResourceGroup
: Specifies the group that holds the configuration to update.--name myAppConfig
: Identifies the configuration store that you intend to update.
Example Output:
{
"id": "/subscriptions/xxxxxxx/resourceGroups/myResourceGroup/providers/Microsoft.AppConfiguration/configurationStores/myAppConfig",
"location": "eastus",
"name": "myAppConfig",
"resourceGroup": "myResourceGroup",
"provisioningState": "Succeeded",
"tags":{},
"sku": {
"name": "Standard"
}
}
Conclusion:
The Azure CLI’s az appconfig
command-line tool provides an efficient way to manage application settings in the Azure cloud. From creating and deleting configurations to listing and updating them, it gives developers and IT administrators the tools they need to keep their applications running optimally and securely. Understanding these use cases equips you to utilize Azure App Configuration effectively and maintain control over your application environment.