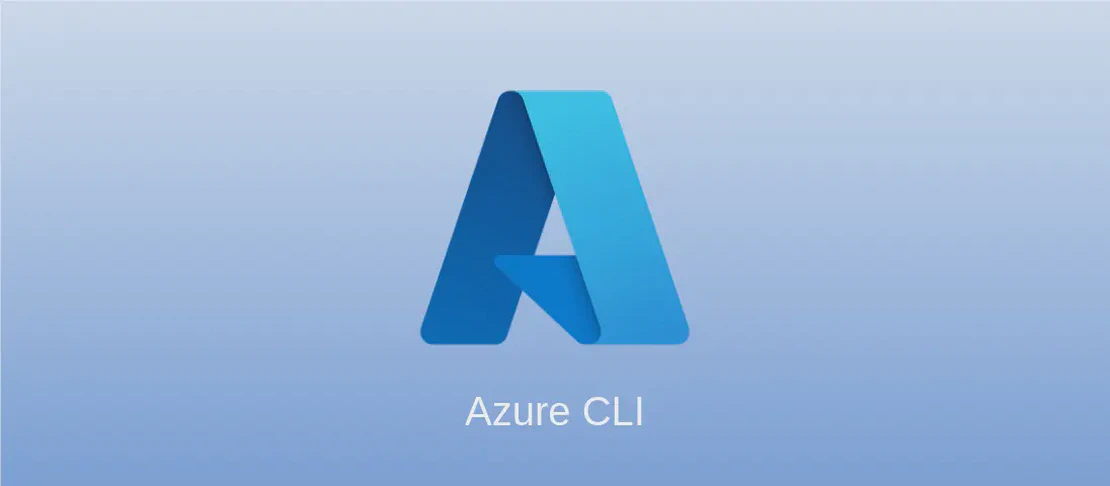
How to Use the Command 'az login' (with examples)
The az login
command is a critical part of the Azure Command-Line Interface (CLI), often referred to simply as az
. This utility enables users to authenticate with Microsoft Azure, allowing them to manage and deploy resources within the Azure cloud platform straight from their terminal or command prompt. Whether you’re an administrator managing multiple cloud environments, a developer deploying applications, or an IT professional configuring networks, the az login
command provides essential access to Azure’s wide suite of cloud services.
Use case 1: Log In Interactively
Code:
az login
Motivation:
The interactive login is ideal for users who prefer a straightforward approach to authenticate to Azure. It’s especially useful when you’re at a computer with GUI capabilities, allowing you to handle everything from the terminal with minimal command-line arguments. This is mostly used for first-time logins or for users who don’t have a complex set of identities.
Explanation:
In this scenario, the az login
is run without additional parameters, prompting you to either use a web browser to authenticate or to follow the device login process. This method leverages your default web browser to guide you through the authentication process by entering your Microsoft credentials. It’s a simple method that offers the most user-friendly experience.
Example output:
If browser is not opened, use the command below:
az login --use-device-code
Opening in existing browser session.
[
{
"cloudName": "AzureCloud",
"homeTenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"isDefault": true,
"managedByTenants": [],
"name": "Subscription-Name",
"state": "Enabled",
"tenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"user": {
"name": "user@example.com",
"type": "user"
}
}
]
Use case 2: Log In with a Service Principal Using a Client Secret
Code:
az login --service-principal --username http://azure-cli-service-principal --password secret --tenant someone.onmicrosoft.com
Motivation:
Utilizing a service principal with a client secret is a common and secure method to allow automated systems to interact with Azure resources. It’s suited for scripts or applications requiring programmatic access to Azure, helping to avoid storing personal account credentials in your automated deployments.
Explanation:
--service-principal
: This flag indicates that the login will be performed using a service principal, which is essentially a non-human identity that allows applications to access Azure.--username
: The service principal name, typically a URL that uniquely identifies the application in Azure Active Directory (AAD).--password
: This is the client secret associated with the service principal, effectively acting as a password.--tenant
: The tenant ID corresponds to your Azure Active Directory instance, helping Azure identify the context in which your service principal exists.
Example output:
[
{
"cloudName": "AzureCloud",
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"isDefault": true,
"managedByTenants": [],
"name": "SP-Subscription-Name",
"state": "Enabled",
"tenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"user": {
"name": "http://azure-cli-service-principal",
"type": "servicePrincipal"
}
}
]
Use case 3: Log In with a Service Principal Using a Client Certificate
Code:
az login --service-principal --username http://azure-cli-service-principal --password path/to/cert.pem --tenant someone.onmicrosoft.com
Motivation:
This method is especially beneficial for situations where security policies forbid the storage of secrets in plain text. By using a certificate, you can ensure an even more secure authentication mechanism that is less prone to being compromised than traditional usernames and passwords.
Explanation:
--service-principal
: Similar to the previous use case, this marks the use of a service principal for login.--username
: The service principal’s unique identifier in Azure AD.--password
: Rather than a secret, this argument now takes the path to the PEM file holding the certificate. Azure uses this certificate to authenticate the service principal.--tenant
: Defines the boundaries within Azure AD for the authentication context.
Example output:
[
{
"cloudName": "AzureCloud",
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"isDefault": true,
"managedByTenants": [],
"name": "Cert-Subscription-Name",
"state": "Enabled",
"tenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"user": {
"name": "http://azure-cli-service-principal",
"type": "servicePrincipal"
}
}
]
Use case 4: Log In Using a VM’s System Assigned Identity
Code:
az login --identity
Motivation:
System-assigned managed identities are useful for logging Azure Virtual Machines (VMs) securely without managing credentials. This identity is tied to the lifecycle of a particular VM, making it an excellent option for services requiring authentication without manual intervention.
Explanation:
--identity
: This argument allows a VM’s system-assigned managed identity to authenticate itself without additional parameters. There’s no need for usernames, passwords, or tenant details, simplifying the process and increasing security for VM-managed operations.
Example output:
[
{
"cloudName": "AzureCloud",
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"isDefault": true,
"managedByTenants": [],
"name": "VM-Subscription-Name",
"state": "Enabled",
"tenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"user": {
"assignedIdentityInfo": {
"id": "XXXXXX"
},
"type": "systemAssignedIdentity"
}
}
]
Use case 5: Log In Using a VM’s User Assigned Identity
Code:
az login --identity --username /subscriptions/subscription_id/resourcegroups/my_rg/providers/Microsoft.ManagedIdentity/userAssignedIdentities/my_id
Motivation:
User-assigned identities provide shared identity capabilities across different resources. This scenario is particularly useful for scenarios where you have multiple VMs (or other resources) that need to share the same managed identity rather than having one assigned per VM.
Explanation:
--identity
: Specifies the use of a managed identity for logging in.--username
: Unlike the system-assigned identity, this requires specifying the full path of the user-assigned identity in Azure, complete with subscription ID and resource group, to inform Azure exactly which identity to use.
Example output:
[
{
"cloudName": "AzureCloud",
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"isDefault": true,
"managedByTenants": [],
"name": "User-Assigned-Sub-Name",
"state": "Enabled",
"tenantId": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"user": {
"assignedIdentityInfo": {
"id": "my_id"
},
"type": "userAssignedIdentity"
}
}
]
Conclusion:
The az login
command is a versatile and powerful tool for managing Azure authentication, adaptable for various scenarios ranging from simple interactive logins to more secure programmatic access. The examples above demonstrate its flexibility in supporting different authentication needs, enabling users and applications to interact seamlessly and securely with Microsoft’s Azure platform.