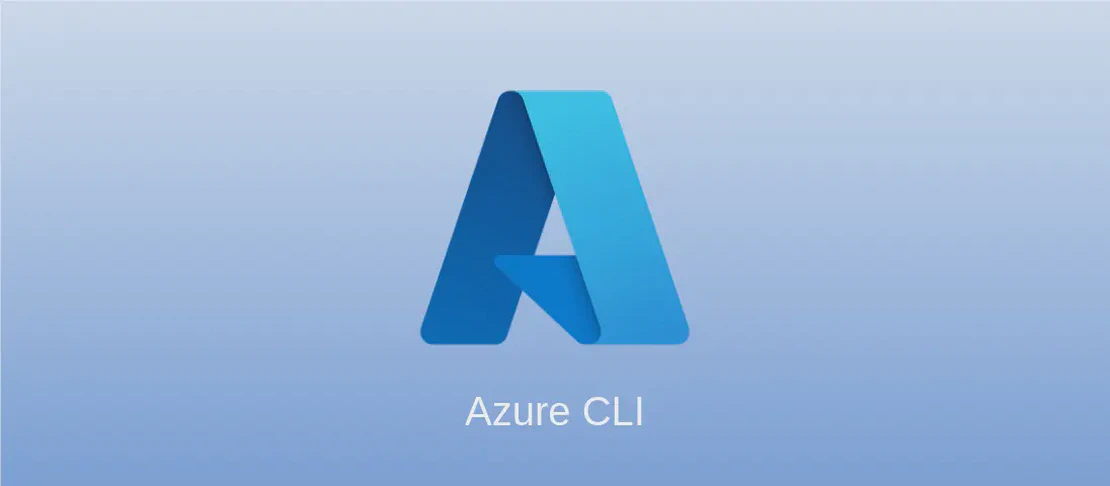
How to use the command 'az pipelines' (with examples)
The ‘az pipelines’ command is a part of the Azure Command Line Interface (CLI), which allows users to manage their Azure Pipelines resources efficiently from the command line. As a powerful DevOps tool offering from Microsoft, Azure Pipelines facilitate building, testing, and deploying with continuous integration and continuous deployment (CI/CD) capabilities on any platform.
Use case 1: Create a new Azure Pipeline (YAML based)
Code:
az pipelines create --org organization_url --project project_name --name pipeline_name --description description --repository repository_name --branch branch_name
Motivation: Creating a new pipeline is the first step in automating your development workflow. By setting up CI/CD pipelines, you can ensure that your project is built, tested, and deployed continuously, thus enhancing efficiency and reducing possible downtime of crucial applications.
Explanation:
--org organization_url
: Specifies the URL of the Azure DevOps organization. It is crucial to provide the correct organization URL to ensure the pipeline is created in the intended DevOps environment.--project project_name
: The name of the project within the organization where the pipeline will be created. Specifying the project ensures that the pipeline is associated correctly with the intended repository and branch.--name pipeline_name
: A unique name for the pipeline. This identifier allows users and the system to easily recognize and manage the pipeline.--description description
: A brief description of the pipeline’s purpose, which helps team members understand its function at a glance.--repository repository_name
: Indicates the code repository that the pipeline will be associated with. This connection allows the pipeline to access the necessary codebase for builds and deployments.--branch branch_name
: Specifies the branch of the repository the pipeline should track for changes. This ensures that the pipeline only triggers on relevant updates, aligning with best practices for branch-based development.
Example output:
Pipeline 'pipeline_name' created successfully in project 'project_name' under the organization 'organization_url'.
Use case 2: Delete a specific pipeline
Code:
az pipelines delete --org organization_url --project project_name --id pipeline_id
Motivation: Over time, certain pipelines may become obsolete as projects evolve or are completed. Deleting these redundant pipelines helps maintain a clean and organized DevOps infrastructure, preventing unnecessary resource usage and potential confusion.
Explanation:
--org organization_url
: Targets the specific Azure DevOps organization where the pipeline exists.--project project_name
: Specifies the project within the organization that houses the pipeline intended for deletion.--id pipeline_id
: The unique identifier of the pipeline you wish to delete. Having the specific ID ensures that you are removing the correct pipeline without affecting others.
Example output:
Pipeline with ID 'pipeline_id' deleted successfully from project 'project_name'.
Use case 3: List pipelines
Code:
az pipelines list --org organization_url --project project_name
Motivation: Listing all existing pipelines in a project helps maintain an overview of current workflows. It is a valuable tool for audits, reports, or when planning updates and optimizations of your CI/CD processes.
Explanation:
--org organization_url
: Directs the command to the respective Azure DevOps organization.--project project_name
: Specifies the project from which the pipelines will be listed. This narrows down the command to the relevant scope, minimizing load time and focusing on pertinent information.
Example output:
[
{
"id": "1",
"name": "Pipeline1",
"status": "Running"
},
{
"id": "2",
"name": "Pipeline2",
"status": "Stopped"
}
]
Use case 4: Enqueue a specific pipeline to run
Code:
az pipelines run --org organization_url --project project_name --name pipeline_name
Motivation: Triggering a pipeline on demand is crucial for situations where new changes need to be integrated immediately, such as hotfixes or time-sensitive updates. It provides control to DevOps engineers for handling urgent code deployments.
Explanation:
--org organization_url
: Identifies the specific Azure DevOps organization.--project project_name
: Specifies the project within the organization.--name pipeline_name
: The name of the pipeline that needs to be run. This name acts as the directive for the system to locate and trigger the specific pipeline.
Example output:
Pipeline 'pipeline_name' enqueued for execution successfully in project 'project_name'.
Use case 5: Get the details of a specific pipeline
Code:
az pipelines show --org organization_url --project project_name --name pipeline_name
Motivation: Fetching and reviewing pipeline details assists in monitoring and optimizing the CI/CD processes. This feature is helpful for debugging, documentation, and ensuring that pipelines meet specified requirements.
Explanation:
--org organization_url
: Points to the Azure DevOps organization hosting the pipeline.--project project_name
: Specifies the project in which the pipeline is located.--name pipeline_name
: Indicates the particular pipeline whose details need to be retrieved.
Example output:
{
"id": "1",
"name": "pipeline_name",
"description": "This pipeline builds and deploys the application.",
"repository": "repository_name",
"branch": "main"
}
Use case 6: Update a specific pipeline
Code:
az pipelines update --org organization_url --project project_name --name pipeline_name --new-name pipeline_new_name --new-folder-path user1/production_pipelines
Motivation: Keeping pipelines updated with changing organizational standards or project goals is essential for smooth and effective DevOps workflows. This use case allows for the renaming and relocation of pipelines, reflecting changes or reorganization within projects.
Explanation:
--org organization_url
: Targets the correct Azure DevOps organization.--project project_name
: Specifies the related project.--name pipeline_name
: The current name of the pipeline that you are updating.--new-name pipeline_new_name
: Proposes a new name for the pipeline, assisting in rebranding or aligning the pipeline name with updated project naming conventions.--new-folder-path user1/production_pipelines
: Sets a new folder path for the pipeline, reorganizing it within the DevOps structure for better management and access.
Example output:
Pipeline 'pipeline_name' updated successfully to 'pipeline_new_name' with new path 'user1/production_pipelines'.
Use case 7: List all agents in a pool
Code:
az pipelines agent list --org organization_url --pool-id agent_pool
Motivation: Agents execute pipelines and therefore managing them effectively is key to maintaining continuous integration efficiency. Listing agents helps assess resource allocation, performance, and readiness metrics.
Explanation:
--org organization_url
: Identifies the Azure DevOps organization.--pool-id agent_pool
: Specifies the identifier of the agent pool whose agents are to be listed. This helps produce a focused list of relevant agents for the selected tasks.
Example output:
[
{
"agentId": "101",
"name": "Agent1",
"status": "Online"
},
{
"agentId": "102",
"name": "Agent2",
"status": "Offline"
}
]
Conclusion:
Utilizing the ‘az pipelines’ command unlocks a versatile strategy for managing Azure Pipelines resources directly from the command line, enhancing DevOps productivity and control. With each use case illustrated above, users can leverage the command’s capabilities to create, update, delete, list, and manage pipelines effectively, resulting in streamlined CI/CD processes and improved application deployments.