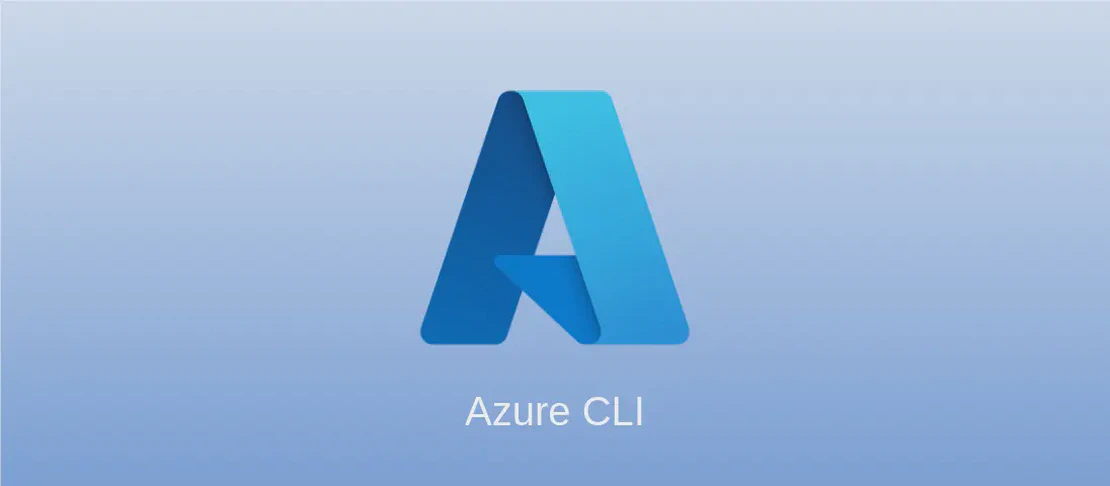
How to use the command az repos (with examples)
The az repos
command is part of the Azure Command-Line Interface (CLI) and is used to manage Azure DevOps repositories. It provides various operations that can be performed on repositories, such as listing repositories, adding policies, and managing pull requests.
Use case 1: List all repos in a specific project
Code:
az repos list --project project_name
Motivation: This command is useful when you want to get a list of all repositories within a specific project in Azure DevOps. It can help in understanding the structure and organization of your projects.
Explanation:
az repos list
: The command to list repositories.--project
: Specifies the name or ID of the project in Azure DevOps.
Example output:
[
{
"id": "repository_id",
"name": "repository_name",
"url": "repository_url"
},
...
]
Use case 2: Add policy on a specific branch of a specific repository to restrict basic merge
Code:
az repos policy merge-strategy create --repository-id repository_id_in_repos_list --branch branch_name --blocking --enabled --allow-no-fast-forward false --allow-rebase true --allow-rebase-merge true --allow-squash true
Motivation: This command allows you to add a merge strategy policy to a specific branch of a repository in Azure DevOps. This policy ensures that only certain types of merges are allowed, providing better control over the development process.
Explanation:
az repos policy merge-strategy create
: The command to create a merge strategy policy.--repository-id
: Specifies the ID of the repository in Azure DevOps.--branch
: Specifies the name of the branch on which the policy should be added.--blocking
: Indicates that the policy should block merging if the conditions are not met.--enabled
: Enables the policy.--allow-no-fast-forward
: Specifies whether to allow non-fast-forward merges.--allow-rebase
: Specifies whether to allow rebase merges.--allow-rebase-merge
: Specifies whether to allow rebase-merge merges.--allow-squash
: Specifies whether to allow squash merges.
Example output: None
Use case 3: Add build validation on a specific repository, using an existing build pipeline, to be triggered automatically on source update
Code:
az repos policy build create --repository-id repository_id --build-definition-id build_pipeline_id --branch main --blocking --enabled --queue-on-source-update-only true --display-name name --valid-duration minutes
Motivation: This command is useful when you want to add build validation to a specific repository in Azure DevOps. Build validation ensures that code changes do not break the build and allows for early detection of issues.
Explanation:
az repos policy build create
: The command to create a build validation policy.--repository-id
: Specifies the ID of the repository in Azure DevOps.--build-definition-id
: Specifies the ID of the build pipeline to be used for validation.--branch
: Specifies the name of the branch on which the policy should be added.--blocking
: Indicates that the policy should block merging if the conditions are not met.--enabled
: Enables the policy.--queue-on-source-update-only
: Specifies whether to trigger the build validation only on source updates.--display-name
: Specifies the display name of the build validation policy.--valid-duration
: Specifies the duration for which the build validation policy is valid.
Example output: None
Use case 4: List all active Pull Requests on a specific repository within a specific project
Code:
az repos pr list --project project_name --repository repository_name --status active
Motivation: This command is useful when you want to get a list of all active pull requests in a specific repository within a project in Azure DevOps. It provides an overview of ongoing development work and allows for easy tracking and management.
Explanation:
az repos pr list
: The command to list pull requests.--project
: Specifies the name or ID of the project in Azure DevOps.--repository
: Specifies the name or ID of the repository in Azure DevOps.--status
: Specifies the status filter for pull requests. In this case, we are filtering for only active pull requests.
Example output:
[
{
"pullRequestId": "pull_request_id",
"title": "pull_request_title",
"status": "active",
...
},
...
]
Conclusion:
The az repos
command in Azure CLI provides a set of powerful capabilities to manage Azure DevOps repositories. With functionalities like listing repositories, adding policies, and managing pull requests, it allows for efficient repository management and collaboration within teams.