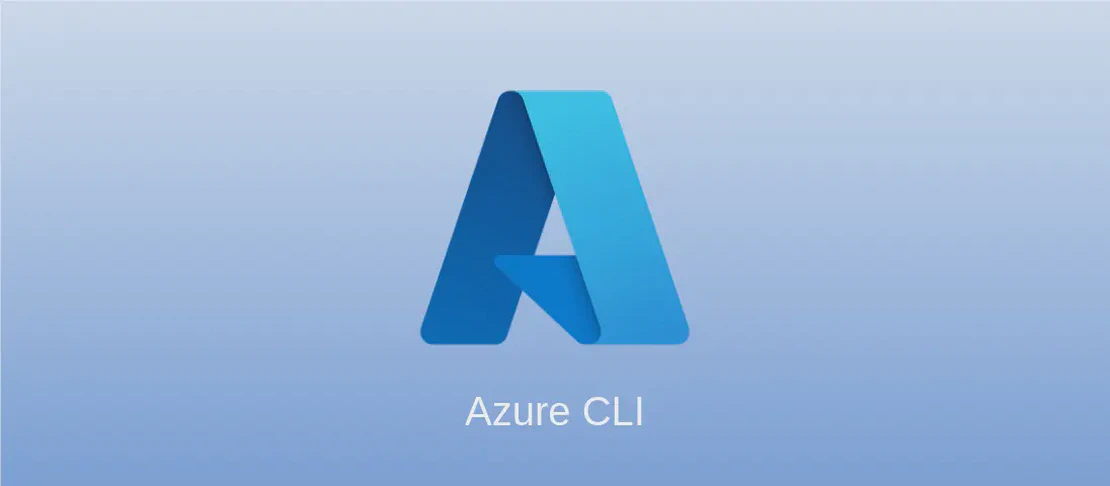
How to Manage SSH Keys with 'az sshkey' (with examples)
The az sshkey
command is a powerful tool within the Azure Command-Line Interface (CLI) that allows users to manage SSH public keys for use with virtual machines on Azure. This makes it easier to securely access your virtual machines by using SSH keys, which are considered more secure than traditional password-based authentication methods. Whether you want to create new SSH keys, upload existing ones, or manage and view your current set of SSH public keys, az sshkey
offers a comprehensive set of commands to handle these tasks efficiently.
Use case 1: Create a New SSH Key
Code:
az sshkey create --name MyNewKey --resource-group MyResourceGroup
Motivation:
Creating a new SSH key directly within Azure’s environment can be an efficient way to manage access controls across your virtual machines. This is particularly useful for system administrators who want to ensure that every virtual machine is equipped with a consistent and unique SSH key, enhancing security and standardization practices across their infrastructure.
Explanation:
az sshkey create
: This is the main command used to create a new SSH key.--name MyNewKey
: Specifies the desired name for the new SSH key. This name should be unique within the resource group to avoid any conflicts.--resource-group MyResourceGroup
: Identifies the resource group in which the SSH key will be stored. A resource group acts as a container that holds related resources, which allows for easy management and organization.
Example Output:
{
"id": "/subscriptions/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/resourceGroups/MyResourceGroup/providers/Microsoft.Compute/sshPublicKeys/MyNewKey",
"name": "MyNewKey",
"publicKey": "ssh-rsa AAAAB3Nz...",
"resourceGroup": "MyResourceGroup"
}
Use case 2: Upload an Existing SSH Key
Code:
az sshkey create --name MyExistingKey --resource-group MyResourceGroup --public-key "@/path/to/your/key.pub"
Motivation:
Uploading an existing SSH key is advantageous when you want to maintain consistency of keys across multiple platforms or when you want to integrate Azure virtual machines into your existing SSH key infrastructure. By uploading a pre-existing key, you can utilize existing public keys that you may already be using with other services or infrastructures, saving time and maintaining a uniform security approach.
Explanation:
az sshkey create
: This command is utilized to create or upload an SSH key.--name MyExistingKey
: The designated name you want for your SSH key in Azure, similar to labeling a file for organizational purposes.--resource-group MyResourceGroup
: Indicates the resource group location for the SSH key, aiding in resource categorization and management.--public-key "@/path/to/your/key.pub"
: Points the command to the location of the public key file you wish to upload. The@
symbol indicates that you’re referencing a file path, which should point to a.pub
file containing your public key.
Example Output:
{
"id": "/subscriptions/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/resourceGroups/MyResourceGroup/providers/Microsoft.Compute/sshPublicKeys/MyExistingKey",
"name": "MyExistingKey",
"publicKey": "ssh-rsa AAAAB3Nz...",
"resourceGroup": "MyResourceGroup"
}
Use case 3: List All SSH Public Keys
Code:
az sshkey list
Motivation:
Listing all SSH public keys is crucial for auditing and managing your SSH keys, especially when you’re maintaining multiple virtual machines or dealing with a large-scale cloud infrastructure. This command provides a quick overview and verifies which keys are available, helping administrators track which keys are active and eliminating obsolete or duplicate keys to enforce access security.
Explanation:
az sshkey list
: A straightforward command that queries Azure for a list of all the SSH keys currently stored.
Example Output:
[
{
"id": "/subscriptions/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/resourceGroups/MyResourceGroup/providers/Microsoft.Compute/sshPublicKeys/MyNewKey",
"name": "MyNewKey",
"publicKey": "ssh-rsa AAAAB3Nz...",
"resourceGroup": "MyResourceGroup"
},
{
"id": "/subscriptions/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/resourceGroups/MyResourceGroup/providers/Microsoft.Compute/sshPublicKeys/MyExistingKey",
"name": "MyExistingKey",
"publicKey": "ssh-rsa AAAAB3Nz...",
"resourceGroup": "MyResourceGroup"
}
]
Use case 4: Show Information About an SSH Public Key
Code:
az sshkey show --name MyNewKey --resource-group MyResourceGroup
Motivation:
This use case is important for inspecting the details of a specific SSH key. By displaying nuanced information about each key, such as its associated resource group and public key data, administrators can validate key configurations and ensure that resources are properly secured, thus preventing unauthorized access to virtual machines.
Explanation:
az sshkey show
: The core command to display detailed information on a specific SSH key.--name MyNewKey
: This is the name of the SSH key you want to investigate. Providing the correct name ensures that you retrieve data for the intended SSH key.--resource-group MyResourceGroup
: The specific resource group that houses the SSH key, which helps the command locate the correct key among potentially many.
Example Output:
{
"id": "/subscriptions/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/resourceGroups/MyResourceGroup/providers/Microsoft.Compute/sshPublicKeys/MyNewKey",
"name": "MyNewKey",
"publicKey": "ssh-rsa AAAAB3Nz...",
"resourceGroup": "MyResourceGroup"
}
Conclusion:
Managing SSH keys is an integral part of securing access to your Azure virtual machines. The az sshkey
command provides essential functions to create, upload, list, and inspect SSH keys, aiding administrators and users in maintaining a robust and secure access system. By following the examples provided, users can efficiently manage their SSH keys in Azure, ensuring both security and ease of access.