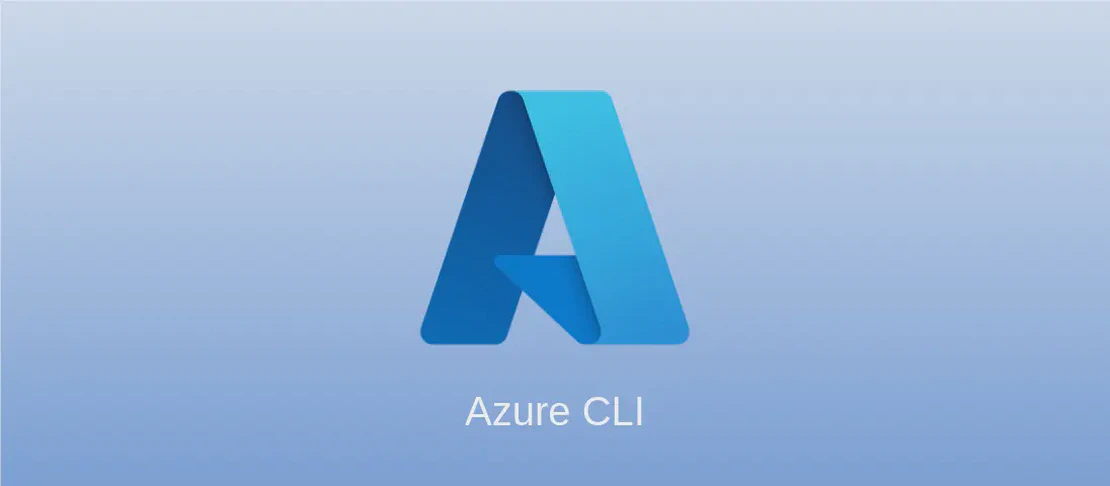
Managing Azure Storage Accounts using Azure CLI (with examples)
The Azure CLI command az storage account
is a powerful tool that allows users to manage their Azure storage accounts directly from the command line. As part of the Azure Command-Line Interface (CLI), often referred to simply as az
, this command offers flexibility and convenience for automating tasks, managing storage resources, and integrating storage solutions within larger cloud infrastructures. The Azure CLI provides a comprehensive interface for interacting with Azure services without having to access the Azure Portal, making it an invaluable resource for developers, system administrators, and DevOps engineers alike.
Use case 1: Create a storage account
Code:
az storage account create --name storage_account_name --resource-group azure_resource_group --location azure_location --sku storage_account_sku
Motivation:
Creating a new storage account in Azure sets the foundation for storing and managing data. Whether you’re deploying a new application, setting up an environment for development, or preparing to work with large datasets, a storage account is where you’ll store objects, files, queues, and tables. Automating this task through the CLI helps streamline processes, reduce human error, and integrate seamlessly with deployment scripts.
Explanation:
--name storage_account_name
: This specifies the unique name for your storage account. It’s a crucial identifier in Azure and must be globally unique.--resource-group azure_resource_group
: Resource groups in Azure serve as containers for multiple resources, allowing you to manage them as a single entity. Specifying a resource group helps in organizing the storage account along with related resources.--location azure_location
: The physical location or region where the data will be stored. Choosing the appropriate location can optimize performance and ensure compliance with data residency requirements.--sku storage_account_sku
: This defines the service tier of the storage account. It determines the performance, cost, and data redundancy solutions available (e.g., Standard_LRS, Premium_LRS).
Example output:
Upon successful execution, the command will return a JSON-like output containing the details of the newly created storage account, including its name, type, location, and resource group among other properties.
Use case 2: Generate a shared access signature for a specific storage account
Code:
az storage account generate-sas --account-name storage_account_name --name account_name --permissions sas_permissions --expiry expiry_date --services storage_services --resource-types resource_types
Motivation:
A Shared Access Signature (SAS) is a powerful mechanism that provides delegated access to resources in your storage account. It allows you to grant limited, time-bound permissions to access your Azure storage resources without exposing your account keys. This is especially useful in scenarios where you need to share resources with external entities or temporary users securely.
Explanation:
--account-name storage_account_name
: The name of the storage account for which you are generating the SAS.--name account_name
: Typically refers to the storage name context for the operation.--permissions sas_permissions
: This parameter defines the level and type of access the SAS token is granting (e.g., read, write).--expiry expiry_date
: Sets the expiration for the SAS, ensuring that the permissions are only available for a limited time.--services storage_services
: Specifies the storage services (blob, file, queue, table) that the SAS will cover.--resource-types resource_types
: Indicates the level of resources being accessed such as service, container, or object.
Example output:
The command will output a SAS token string that can be used to authenticate and access specified resources within the storage account.
Use case 3: List storage accounts
Code:
az storage account list --resource-group azure_resource_group
Motivation:
Listing storage accounts is a fundamental administrative task that allows users to view all the storage accounts within a specific resource group. This is essential for auditing, resource management, and planning purposes. It provides insight into the available resources, helping you make informed decisions about storage optimization and cost management.
Explanation:
--resource-group azure_resource_group
: Limits the scope of the list operation to a particular resource group, providing a more manageable list by filtering out irrelevant entries if you manage multiple resource groups.
Example output:
The output will include a list of storage accounts within the specified resource group, presented in a JSON format. Each entry in the list contains vital information about the storage account, such as its name, location, and status.
Use case 4: Delete a specific storage account
Code:
az storage account delete --name storage_account_name --resource-group azure_resource_group
Motivation:
Deleting a storage account may be necessary when decommissioning an application or environment, reducing resource costs, or organizing resources more efficiently. Using the CLI for this task ensures that the process is swift, controlled, and can be integrated into clean-up scripts for automated resource management.
Explanation:
--name storage_account_name
: The specific storage account you want to delete. It must be identified correctly to avoid accidentally deleting the wrong storage.--resource-group azure_resource_group
: Ensures that the command targets the correct instance by specifying the associated resource group. This can help avoid conflicts or errors in environments with multiple resource groups.
Example output:
Successful execution of this command will not return any output, but it will remove the specified storage account and its contents, effectively freeing up associated resources.
Conclusion
Understanding and utilizing the az storage account
commands effectively allows users to manage their Azure storage accounts with precision and efficiency. From creating storage accounts to managing access through SAS tokens and removing unneeded resources, these capabilities form an integral part of cloud resource management. By automating these tasks through the Azure CLI, users can enhance productivity, reduce manual effort, and maintain a consistent cloud infrastructure.