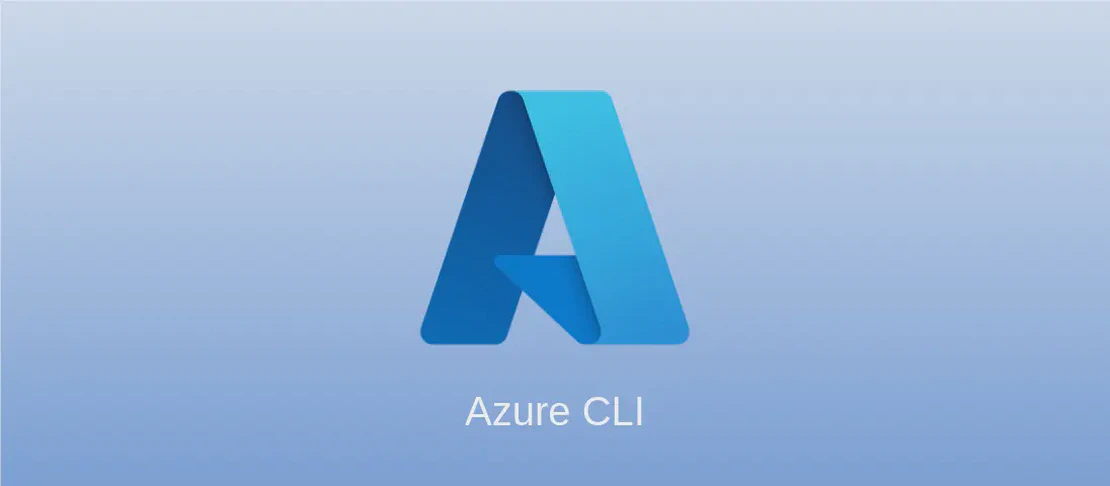
Mastering Azure CLI for Cloud Storage Management (with examples)
Azure CLI, specifically the az storage
command, offers a robust suite of options for managing Azure Cloud Storage resources efficiently. By utilizing these commands, users can seamlessly create, maintain, and configure storage accounts, providing a flexible and scalable cloud storage solution. The versatility of these commands enables tailored solutions for diverse business needs, ensuring data storage and accessibility are both effective and secure.
Use Case 1: Creating a Storage Account with a Specific Location
Code:
az storage account create --resource-group group_name --name account_name -l location --sku account_sku
Motivation:
Creating a storage account in a specific location is fundamental to optimizing geographic performance and compliance with data residency requirements. For businesses operating in multiple regions, having storage accounts geographically near their operations can dramatically reduce latency and improve application performance. Additionally, some organizational policies or governmental regulations may require data to be stored within specific regions, necessitating location-specific storage strategies.
Explanation:
az storage account create
: Initiates the creation of a new storage account.--resource-group group_name
: Specifies the resource group in which the storage account will be created. Resource groups help organize and manage Azure resources efficiently.--name account_name
: Defines a unique name for the storage account. It will be used to reference this particular account in subsequent operations.-l location
: Sets the physical data center location for the storage account. Options include various regions around the world, such as ‘EastUS’, ‘WestEurope’, etc.--sku account_sku
: Determines the performance tier of the storage account, such as Standard_LRS (Locally Redundant Storage) or Premium_LRS, which influences cost and durability.
Example Output:
{
"id": "/subscriptions/your_subscription_id/resourceGroups/group_name/providers/Microsoft.Storage/storageAccounts/account_name",
"sku": { "name": "Standard_LRS" },
"name": "account_name",
"location": "eastus"
// Additional configuration details...
}
Use Case 2: Listing All Storage Accounts in a Resource Group
Code:
az storage account list --resource-group group_name
Motivation:
Listing all storage accounts within a specific resource group is essential for monitoring and management tasks. It provides a concise summary of the available storage resources, aiding in inventory checks, usage auditing, and quick assessments of account configurations. This is particularly useful for large organizations needing to maintain comprehensive oversight across numerous environments and processing demands.
Explanation:
az storage account list
: Command used to retrieve a list of storage accounts.--resource-group group_name
: Filters the list to display only those accounts contained within the specified resource group.
Example Output:
[
{
"name": "account1",
"location": "westus",
"resourceGroup": "group_name",
"sku": { "name": "Standard_LRS" },
// Other details...
},
{
"name": "account2",
"location": "eastus",
"resourceGroup": "group_name",
"sku": { "name": "Premium_LRS" },
// Other details...
}
]
Use Case 3: Listing Access Keys for a Storage Account
Code:
az storage account keys list --resource-group group_name --name account_name
Motivation:
Access keys are crucial for securing and managing access to a storage account. Listing access keys is a regular operation for developers and IT administrators to configure applications and services that require authentication to store or retrieve data from a storage account. It ensures that authorized users can connect to storage accounts while maintaining security practices, such as updating keys periodically.
Explanation:
az storage account keys list
: Fetches a list of keys for specified account, used to securely access the storage services.--resource-group group_name
: Specifies the resource group that contains the targeted storage account.--name account_name
: Identifies the exact storage account from which to list the access keys.
Example Output:
[
{
"keyName": "key1",
"permissions": "Full",
"value": "abc123secretkey1value=="
},
{
"keyName": "key2",
"permissions": "Full",
"value": "xyz789secretkey2value=="
}
]
Use Case 4: Deleting a Storage Account
Code:
az storage account delete --resource-group group_name --name account_name
Motivation:
Deleting a storage account is necessary when an account is no longer required or needs to be retired as part of cost-saving measures. This operation is critical for resource management and governance, ensuring unnecessary storage costs are avoided and simplifying the management of active resources across the Azure ecosystem. However, caution is advised as this action is irreversible, resulting in permanent data loss from the account.
Explanation:
az storage account delete
: Executes the deletion operation on the specified storage account.--resource-group group_name
: Identifies the resource group that contains the account to be deleted.--name account_name
: Specifies the storage account to be removed.
Example Output:
No output is typically returned for a successful delete operation. If the command runs successfully, the storage account will be removed from the Azure environment.
Use Case 5: Updating the Minimum TLS Version Setting for a Storage Account
Code:
az storage account update --min-tls-version TLS1_0|TLS1_1|TLS1_2 --resource-group group_name --name account_name
Motivation:
Updating the TLS version for a storage account enhances security by ensuring communications to the account are encrypted using modern, secure protocols. Compliance with current security standards necessitates updating older systems to use newer TLS versions, thus protecting data in transit from potential cyber threats. This configuration is vital for organizations aiming to align with cybersecurity best practices and regulatory requirements.
Explanation:
az storage account update
: Modifies existing configurations for the specified storage account.--min-tls-version TLS1_0|TLS1_1|TLS1_2
: Sets the minimum allowed TLS version for secure communications. Raising the TLS version helps to mitigate vulnerabilities found in older versions.--resource-group group_name
: Determines which resource group the operation targets.--name account_name
: Specifies the exact storage account to be updated.
Example Output:
{
"name": "account_name",
"location": "eastus",
"properties": {
"minimumTlsVersion": "TLS1_2"
// Other account settings...
}
}
Conclusion:
Azure CLI offers a powerful interface for managing Azure Storage accounts effortlessly. Through the examples discussed, one can efficiently create, modify, and manage accounts across various scenarios, facilitating enhanced operation performance, security, and compliance within the Azure ecosystem. By mastering these commands, businesses can streamline their cloud storage needs, hence optimizing cost and operational efficiency.