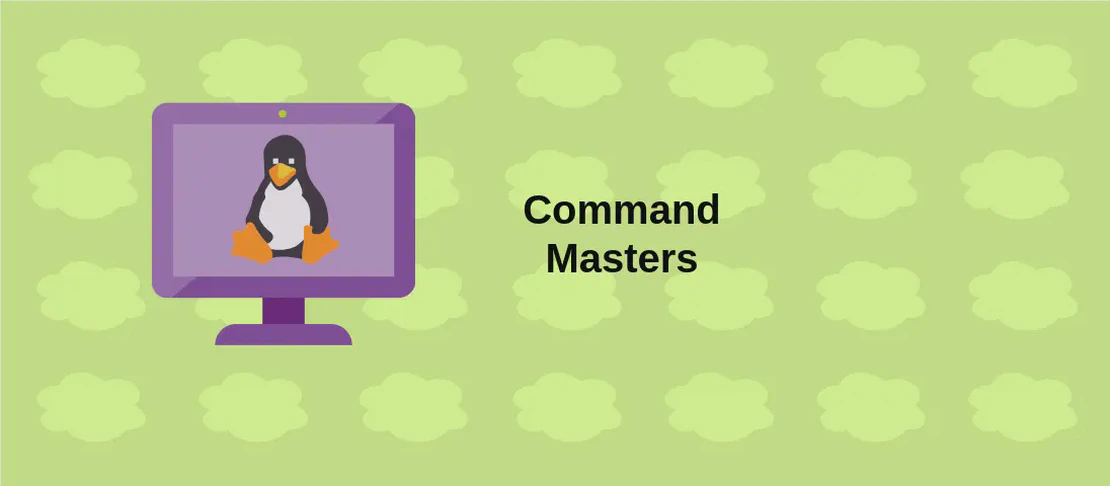
How to Use the Command 'babel' (with Examples)
Babel is a powerful tool used in the world of JavaScript development. As a transpiler, it transforms cutting-edge JavaScript (ES6/ES7) into the more universally accepted ES5 syntax, ensuring compatibility with environments that do not yet support modern syntax features. The utility of Babel extends beyond mere transformation; it provides the foundation for implementing current language features across different browsers and environments, thus broadening the accessibility and functionality of JavaScript code.
Transpile a Specified Input File and Output to stdout
Code:
babel path/to/file
Motivation:
When you’ve written a piece of JavaScript code in ES6+ syntax, you might want to quickly check the ES5 equivalent for testing or learning purposes. Running Babel without any output file specified sends the transpiled code directly to your terminal, providing an immediate look into the transformation process.
Explanation:
babel
: This is the command used to invoke the Babel transpiler.path/to/file
: This is the path to the JavaScript file you wish to transpile. By specifying this path, you tell Babel which file to read and transpile.
Example Output:
Running this command outputs the ES5 version of your JavaScript code directly to the terminal, allowing you to see the transpiled syntax without writing to a file.
Transpile a Specified Input File and Output to a Specific File
Code:
babel path/to/input_file --out-file path/to/output_file
Motivation:
Sometimes, you want to save the transpiled code into a file for later use, debugging, or further processing. This command allows you to keep a permanent record of the transpilation output.
Explanation:
babel
: Invokes the Babel transpiler.path/to/input_file
: Specifies the input file containing ES6+ syntax.--out-file path/to/output_file
: Directs Babel to save the transpiled code in the specified output file path. This is particularly useful when you want to integrate the output file into other workflow processes or applications.
Example Output:
You will find the transpiled ES5 code saved in the file you’ve designated as the output path.
Transpile the Input File Every Time It Is Changed
Code:
babel path/to/input_file --watch
Motivation:
In active development, code changes frequently. Having the transpilation process automatically trigger with each source file modification ensures that the latest changes are always reflected in the transpiled output. This can dramatically improve efficiency in a development environment.
Explanation:
babel
: Executes the transpilation process.path/to/input_file
: The path to the input file that Babel will watch.--watch
: Tells Babel to monitor the specified file for changes continuously. Upon detecting any modification, Babel will re-transpile the file.
Example Output:
The command keeps running until manually stopped, automatically updating the transpiled output whenever you save changes to the input file.
Transpile a Whole Directory of Files
Code:
babel path/to/input_directory
Motivation:
When working on larger projects with multiple JavaScript files, it’s efficient to transpile an entire directory rather than individual files. This ensures that all your files are processed in one go, maintaining consistency across the project.
Explanation:
babel
: The command to start Babel.path/to/input_directory
: The directory containing all the JavaScript files you wish to transpile. Babel will process each file within this directory.
Example Output:
All files in the designated directory will be transpiled, with the results printed in the terminal or saved in a common output location if specified.
Ignore Specified Comma-Separated Files in a Directory
Code:
babel path/to/input_directory --ignore ignored_file1,ignored_file2,...
Motivation:
When transpiling a directory, you might have files that don’t need transpilation, such as configuration files or already transpiled code. Ignoring these files saves processing time and avoids unnecessary overwrites.
Explanation:
babel
: Initiates the Babel command.path/to/input_directory
: Indicates the directory to transpile.--ignore
: This flag allows you to specify a list of files that should be skipped during the transpilation process. Providing a comma-separated list of filenames ensures Babel does not process unneeded files.
Example Output:
The specified files will be omitted from the transpilation results, while the rest of the files in the directory are processed as expected.
Transpile and Output as Minified JavaScript
Code:
babel path/to/input_file --minified
Motivation:
In deployment environments, reducing file size can significantly improve load times and performance. Minifying your transpiled JavaScript removes unnecessary whitespace, comments, and potentially includes other optimizations, resulting in a leaner file.
Explanation:
babel
: Starts the transpiling using Babel.path/to/input_file
: The source file to be transpiled.--minified
: This option tells Babel to produce a minified version of the output, stripping out unnecessary elements to reduce size.
Example Output:
The command outputs a minified ES5 JavaScript code, which is compact and optimized for performance.
Choose a Set of Presets for Output Formatting
Code:
babel path/to/input_file --presets preset1,preset2,...
Motivation:
Babel supports various presets that cater to different environments and project requirements. Using presets tailors the transpilation process to ensure compatibility or apply specific syntax transformations.
Explanation:
babel
: Invokes Babel for transpilation.path/to/input_file
: The file you intend to transpile.--presets
: This option specifies which Babel presets to use. Presets are predefined configurations that determine how Babel should transpile the code, managing plugins and settings according to target environments.
Example Output:
The command produces transpiled code formatted according to the specified presets, optimizing for compatibility and feature support as intended by the presets used.
Display Help
Code:
babel --help
Motivation:
With the vast array of commands and options Babel offers, understanding its full capabilities is crucial. The help command provides a comprehensive list of available options, commands, and descriptions to guide users in utilizing Babel effectively.
Explanation:
babel
: The Babel CLI command.--help
: Instructs Babel to display its help text, outlining usage instructions.
Example Output:
Running this command displays a comprehensive guide to Babel’s commands and options, helping users understand how to apply various flags and configurations effectively.
Conclusion
Babel is an indispensable tool in modern JavaScript development, offering a suite of options to seamlessly transpile code for broader compatibility and enhanced functionality. By understanding and leveraging the different use cases provided, developers can effectively integrate Babel into their workflows, ensuring their JavaScript projects benefit from the latest language features while maintaining compatibility with older environments.