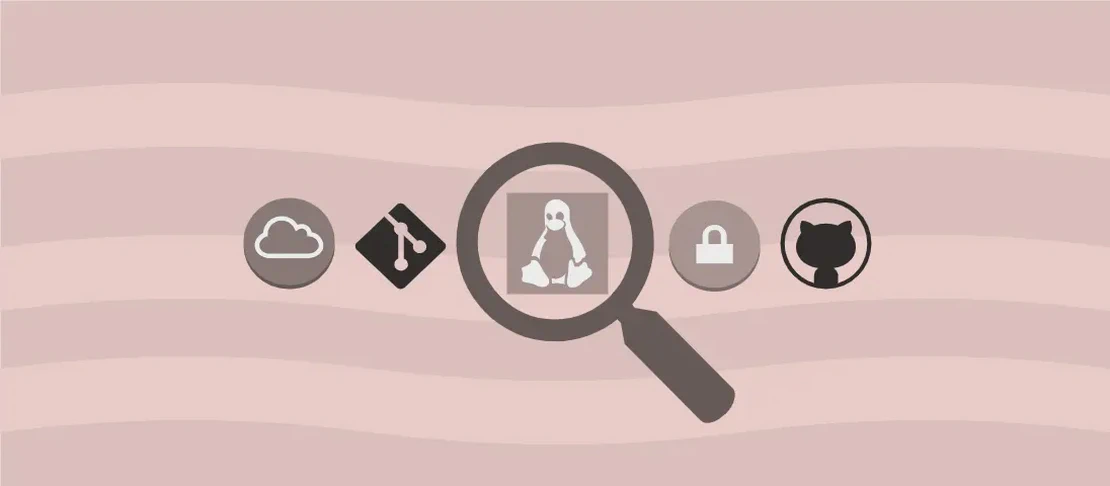
Encoding and Decoding with the 'base32' Command (with examples)
Base32 is a command-line utility used to encode and decode data in Base32, a base-32 numeral system that uses 32 digits represented by the characters A-Z and 2-7. This is useful in various scenarios, such as encoding binary data as text for safe transmission over text-based protocols. The command reads from files or standard input and writes to standard output, providing flexibility in how it’s used.
Use case 1: Encode a File
Code:
base32 path/to/file
Motivation:
Encoding a file in Base32 is a useful way to prepare binary data for email transmission, or any other system that only supports text data. By converting binary files to a Base32 representation, you ensure that the data remains intact and is safely transmitted.
Explanation:
base32
: This is the command to invoke the Base32 encoding tool.path/to/file
: This argument specifies the path to the file you wish to encode.
Example output:
Assuming path/to/file
is a text file containing the string “Hello”, the command outputs something like this:
JBSWY3DP
This is the Base32 encoded form of “Hello”.
Use case 2: Wrap Encoded Output at a Specific Width
Code:
base32 -w 76 path/to/file
Motivation:
Wrapping encoded output at a specific width is beneficial when you need to present encoded data in a more readable format, for example, when including it in a document or report. Specifying a wrap width can also help match line-length requirements of certain protocols or legacy systems.
Explanation:
base32
: Invokes the Base32 encoding tool.-w 76
: This option sets the wrap width to 76 characters per line. The number 76 is an arbitrary choice and can be adjusted based on requirements.path/to/file
: The path to the file to be encoded and wrapped.
Example output:
If the input file has enough data, the output will appear like this:
JBSWY3DPEHPK3PXPJIFGEZDFEBTGY3DP
MZZG623EF5ZQ======
In this example, each line is wrapped after 76 characters, though actual output may vary based on input content.
Use case 3: Decode a File
Code:
base32 -d path/to/file
Motivation:
Decoding a Base32 file is essential when you receive data that has been encoded in this format. By decoding it back to its original form, you can access and utilize the data as it was intended before being encoded.
Explanation:
base32
: Executes the Base32 decoding process.-d
: This flag indicates that the operation is decoding rather than encoding.path/to/file
: Path to the Base32 encoded file that needs to be decoded.
Example output:
For a file containing the encoded text “JBSWY3DP”, the command will output:
Hello
This is the decoded content, assuming it was previously encoded from the original string “Hello”.
Use case 4: Encode from stdin
Code:
echo "Hello, World!" | base32
Motivation:
Encoding directly from standard input is useful when you want to encode data generated by a command-line process or script without first saving it to a file. This is especially handy in scripting or automation tasks.
Explanation:
echo "Hello, World!"
: This command outputs the text “Hello, World!” directly to standard input.| base32
: This pipe sends the standard input to the base32 command to be encoded.
Example output:
JBSWY3DPFQQFO33SNRSCC===
This represents the Base32 encoded value of “Hello, World!”.
Use case 5: Decode from stdin
Code:
echo "JBSWY3DPFQQFO33SNRSCC===" | base32 -d
Motivation:
Decoding from standard input allows you to directly decode Base32 data streamed or piped from another process, which can be extremely efficient in processing large data streams or integrating with other programs.
Explanation:
echo "JBSWY3DPFQQFO33SNRSCC==="
: This sends the Base32 encoded string to standard input.| base32 -d
: This pipes the input directly to the base32 command with the decode flag, which decodes it back to its original form.
Example output:
Hello, World!
The output is the decoded text of the original input.
Conclusion:
The base32
command is a powerful tool for encoding and decoding data between binary and text, allowing data to be securely transmitted and stored in text-based environments. Whether you are working with files or leveraging the power of standard input/output in command-line scripts, understanding these use cases provides practical ways to manage and manipulate encoded data efficiently.