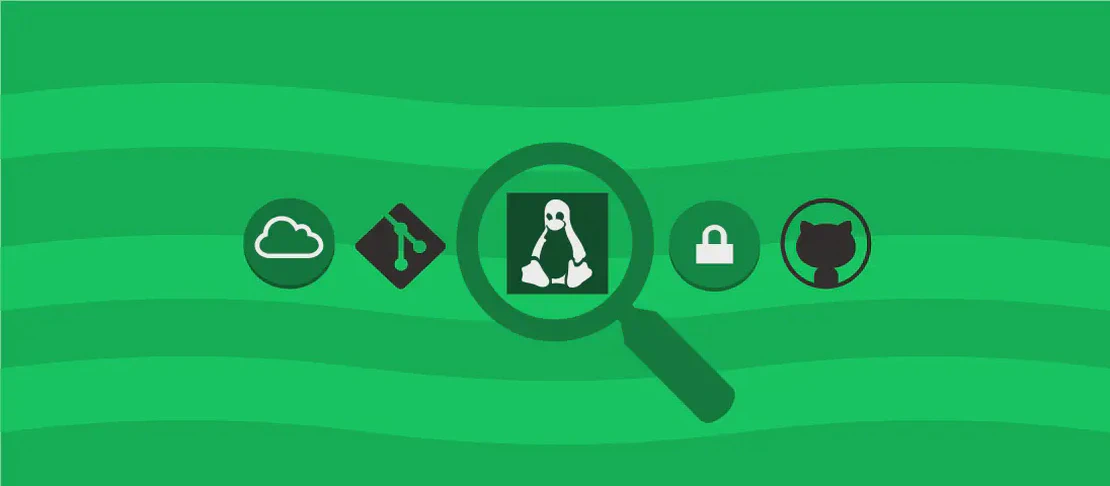
How to use the command 'bash' (with examples)
Bourne-Again SHell, or bash
, is a powerful command-line interpreter used primarily in Unix-based operating systems. It emulates the functionalities of the original Bourne shell (sh
), while incorporating many useful improvements. As a highly flexible tool, bash
is commonly used for executing commands, running scripts, and automating tasks. This guide explores various command-line use cases for bash
, showcasing its versatility.
Use case 1: Start an interactive shell session
Code:
bash
Motivation:
Starting an interactive shell session is fundamental to using any Unix-based operating system. It provides you with a command-line interface where you can execute various commands, run scripts, and perform file manipulations. It serves as a daily interface for users and sysadmins to communicate with the system.
Explanation:
bash
: Invokes thebash
shell, launching an interactive session where commands can be entered one-by-one, and where the environment settings are initialized from configuration files like.bashrc
or.bash_profile
.
Example output:
user@hostname:~$ bash
user@hostname:~$
Use case 2: Start an interactive shell session without loading startup configs
Code:
bash --norc
Motivation:
When troubleshooting or testing new settings, it may be necessary to start a shell session without applying user-specific configuration files (such as .bashrc
). This helps ensure that environment issues are not caused by personalized configurations or scripts that automatically execute.
Explanation:
bash
: Invokes thebash
shell.--norc
: Prevents the startup file (~/.bashrc
) from being read and executed, providing a clean shell environment for testing or debugging.
Example output:
user@hostname:~$ bash --norc
bash-4.3$
Use case 3: Execute specific [c]ommands
Code:
bash -c "echo 'bash is executed'"
Motivation:
Executing a command directly via bash
is useful for scripting or when a specific command or set of commands needs execution without starting an interactive session. This can be part of a larger script or used in system automation tasks.
Explanation:
bash
: Invokes thebash
shell.-c
: Allows you to specify a command string to execute."echo 'bash is executed'"
: The string command thatbash
will execute. Here, it simply prints the text “bash is executed”.
Example output:
bash is executed
Use case 4: Execute a specific script
Code:
bash path/to/script.sh
Motivation:
Executing scripts is a daily operation for developers and sysadmins who need to automate tasks, run batch jobs, or execute complex processes in a controlled environment.
Explanation:
bash
: Invokes thebash
shell.path/to/script.sh
: The path to the script file that contains a series of commands to be executed by the shell.
Example output (depends on the script):
Assuming script.sh
contains:
echo "Hello from script"
Output:
Hello from script
Use case 5: E[x]ecute a specific script, printing each command before executing it
Code:
bash -x path/to/script.sh
Motivation:
When debugging a script or understanding its execution flow, it’s helpful to see each command as it executes. This can assist in identifying erroneous behavior or logical errors within the script.
Explanation:
bash
: Invokes thebash
shell.-x
: Enables a mode of the shell where it prints each command before executing it, making visible the internal workings of the script.path/to/script.sh
: The script file to be executed.
Example output:
Assuming script.sh
contains:
echo "Hello"
echo "World"
Output:
+ echo 'Hello'
Hello
+ echo 'World'
World
Use case 6: Execute a specific script and stop at the first [e]rror
Code:
bash -e path/to/script.sh
Motivation:
It is crucial for maintaining stable environments and consistent results to halt script execution if any command fails. This prevents a script from continuing in an erroneous state which could lead to further complications or system damage.
Explanation:
bash
: Invokes thebash
shell.-e
: Instructsbash
to exit immediately if any command within the script returns a non-zero status, effectively halting execution upon encountering an error.path/to/script.sh
: The script file to be executed.
Example output:
Assuming script.sh
contains:
echo "Start"
false
echo "This won't be printed"
Output:
Start
Use case 7: Execute specific commands from stdin
Code:
echo "echo 'bash is executed'" | bash
Motivation:
Piping commands into bash
via stdin
allows for quick, dynamic execution of commands, which can be particularly useful in pipelines or when generating commands programmatically.
Explanation:
echo "echo 'bash is executed'"
: Theecho
command outputs a string, which is abash
command in this case.| bash
: Pipes the output ofecho
as input tobash
, which executes it as a command.
Example output:
bash is executed
Use case 8: Start a [r]estricted shell session
Code:
bash -r
Motivation:
For system security and control, especially on multi-user systems, it’s sometimes necessary to limit the user’s capabilities in a shell session. A restricted shell can prevent users from performing certain potentially harmful actions.
Explanation:
bash
: Invokes thebash
shell.-r
: Starts bash in restricted mode, where certain critical operations are limited, such as changing the working directory or setting/unsetting shell functions or PATH.
Example output:
bash: cd: restricted
Conclusion:
The bash
command-line interpreter is an essential tool in the toolkit of Unix/Linux users. Its numerous options and modes accommodate a broad range of tasks, from simple command execution and script automation to debugging and enforcing security measures. Understanding its functionalities enables users to harness its full potential in diverse environments.