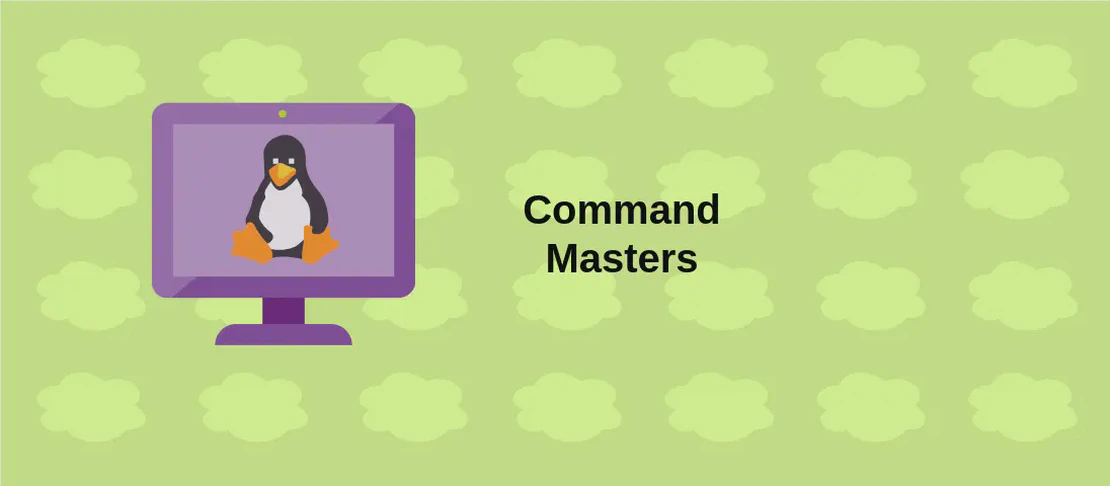
How to Use the Command 'bat' (with Examples)
The bat
command is a modern replacement for the classic cat
command, designed to improve file viewing and concatenation tasks. With features like syntax highlighting, integration with version control systems, and customizable output, bat
enhances productivity for developers and system administrators who frequently work with code and text files. The command is maintained by the open-source community and provides a more visually appealing and informative way to display file contents on the terminal.
Use case 1: Pretty Print the Contents of One or More Files to stdout
Code:
bat path/to/file1 path/to/file2 ...
Motivation:
Developers and system administrators often need to quickly view the contents of files directly in their terminal. Using bat
to pretty print files ensures that the contents are displayed with syntax highlighting, making code easier to read and understand at a glance. This is particularly useful for reviewing script files or code snippets, where structure and keywords are clarified through varied color codes.
Explanation:
bat
: The command being executed.path/to/file1 path/to/file2 ...
: A series of paths to the files you want to display.bat
prints each file’s content while applying syntax highlighting appropriate for the file type.
Example Output:
───────┬────────────────────────────────────────────────────────────────────
│ File: example.py
───────┼────────────────────────────────────────────────────────────────────
1 │ def hello_world():
2 │ print("Hello, World!")
───────┴────────────────────────────────────────────────────────────────────
Use case 2: Concatenate Several Files into the Target File
Code:
bat path/to/file1 path/to/file2 ... > path/to/target_file
Motivation:
There are times when you need to combine multiple file contents into one consolidated file. Using bat
with output redirection (>
) allows you to concatenate the specified files into a single target file. This is especially useful when creating log files, aggregating reports, or merging script files for batch processing.
Explanation:
bat
: The command for printing file content.path/to/file1 path/to/file2 ...
: The files whose contents you want to concatenate.>
: Redirection operator used to specify the output destination.path/to/target_file
: Path to the file where the concatenated result will be saved.
Example Output:
Upon inspection, path/to/target_file
might contain:
def foo():
pass
def bar():
pass
Use case 3: Remove Decorations and Disable Paging
Code:
bat --style plain --pager never path/to/file
Motivation:
Sometimes, you may want to view file content without any additional formatting details like line numbers, headers, or syntax highlighting, especially when focusing on plain data rather than structured code. This command allows for a clean and unadorned view of the file content, similar to what cat
would produce.
Explanation:
bat
: The command for printing file content.--style plain
: Disables all styling; outputs raw text.--pager never
: Disables pagination of the output.path/to/file
: The file whose content is to be displayed.
Example Output:
This is a sample text file.
It has several lines but no decoration.
Use case 4: Highlight a Specific Line or a Range of Lines
Code:
bat -H 5:10 path/to/file
Motivation:
Highlighting specific lines in a file can be crucial when debugging or reviewing code, as it allows users to focus on particular sections of interest. By specifying a line or range of lines, bat
highlights these lines with a distinct background color, enabling quick identification and assessment.
Explanation:
bat
: The command for printing file content.-H 5:10
: Tellsbat
to highlight lines 5 through 10.path/to/file
: Path of the file to examine, with lines 5 to 10 highlighted in the output.
Example Output:
───────┬────────────────────────────────────────────────────────────────────
│ File: example.log
───────┼────────────────────────────────────────────────────────────────────
4 │ Info: Process started
5 │ Warning: Deprecated function used
6 │ Critical: Out of memory error
7 │ Info: Process completed
───────┴────────────────────────────────────────────────────────────────────
Use case 5: Show Non-Printable Characters
Code:
bat -A path/to/file
Motivation:
Displaying non-printable characters like spaces, tabs, and newlines can be very helpful in debugging formatting issues in text files, particularly when whitespace errors are not immediately obvious. By revealing these characters, bat
assists users in recognizing and rectifying subtle faults in file formatting.
Explanation:
bat
: The command for printing file content.-A
: Option to display all non-printable characters.path/to/file
: Specifies the file to analyze for non-printable characters.
Example Output:
This·is·a·sentence.↵
Another·line↵
Use case 6: Remove All Decorations Except Line Numbers
Code:
bat -n path/to/file
Motivation: Removing all decorations except line numbers provides a minimalistic view suitable for scenarios where users need help tracking the line numbers of a document without additional syntax highlighting or headers. This approach effectively balances simplicity and readability in lengthy files.
Explanation:
bat
: The command for printing file content.-n
: Option to include only line numbers in the output decoration.path/to/file
: File path for which stripped down content with only line numbers will be displayed.
Example Output:
1 This is a line with code.
2 Another important line.
Use case 7: Syntax Highlight a JSON File by Explicitly Setting the Language
Code:
bat -l json path/to/file.json
Motivation:
Forcing syntax highlighting for a specific language is useful when working with files that don’t have standard file extensions. By explicitly specifying the language, bat
ensures that the correct syntax highlighting rules are applied, enhancing the readability of the file’s content, particularly JSON files that can be deeply nested and complex.
Explanation:
bat
: The command for printing file content.-l json
: Sets the language for syntax highlighting to JSON, regardless of the file extension.path/to/file.json
: The file whose content is to be displayed with JSON syntax highlighting.
Example Output:
───────┬────────────────────────────────────────────────────────────────────
│ File: config.json
───────┼────────────────────────────────────────────────────────────────────
1 │ {
2 │ "key": "value",
3 │ "array": [1, 2, 3]
4 │ }
───────┴────────────────────────────────────────────────────────────────────
Use case 8: Display All Supported Languages
Code:
bat -L
Motivation:
Users might want to understand which languages bat
can recognize for syntax highlighting to efficiently work with diverse file types. Listing all supported languages provides an overview of bat
’s capabilities and helps ensure that the user can leverage syntax highlighting for the languages they work with.
Explanation:
bat
: The command for printing file content.-L
: Option to list all languages supported bybat
for syntax highlighting.
Example Output:
Languages supported:
- ActionScript
- Ada
- Apache
- Bash
- C
- C++
- ...
Conclusion:
The bat
command significantly enhances the capabilities of the traditional cat
command by incorporating syntax highlighting, version control integration, and flexible options for viewing content. Whether you need to quickly display files, highlight specific lines, or find out which languages are supported, bat
provides an elegant and efficient solution for developers and system administrators to manage and inspect their files more effectively.