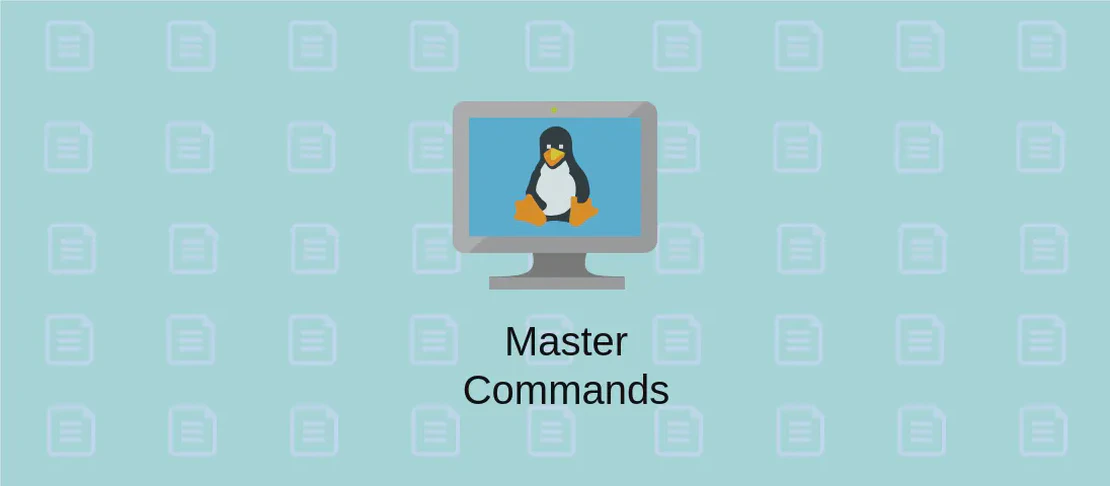
How to Use the Command 'bats' (with examples)
Bash Automated Testing System (BATS) is a TAP-compliant testing framework specifically designed for the Bash scripting environment. It allows users to write tests in a reasonably straightforward syntax and is often used for testing scripts and applications that rely on Bash. The versatility of BATS lies in its ability to output results in various formats, make use of recursion, and handle parallel execution of test jobs, among other features. Test scripting can become a vital component of both development and deployment processes, ensuring functionality and reliability across software environments.
Use Case 1: Run a BATS Test Script and Output Results in the TAP Format
Code:
bats --tap path/to/test.bats
Motivation:
The TAP (Test Anything Protocol) format is a language-agnostic format which provides a standard way of recording test results. By using TAP, users are not only able to aggregate and analyze results easily, but are also able to integrate with other tooling systems that understand TAP. This usage ensures that BATS results are extensible and useful beyond the immediate scope of Bash script testing.
Explanation:
bats
: This is the command to invoke the BATS test runner.--tap
: This flag instructs BATS to output test results in the TAP format.path/to/test.bats
: This is the specification of the test script file to be executed. The.bats
extension signifies a BATS test script.
Example Output:
1..3
ok 1 first test
not ok 2 second test # failed
ok 3 third test
Use Case 2: Count Test Cases of a Test Script Without Running Any Tests
Code:
bats --count path/to/test.bats
Motivation:
Counting test cases without executing them is particularly useful when there is a need to understand the scope of a test suite quickly. This can help testers and developers forecast test execution time, allocate resources, or simply ensure that all planned cases are in place.
Explanation:
bats
: Initiates the BATS testing framework.--count
: Tells BATS to count the number of test cases in the script rather than executing them.path/to/test.bats
: The path to the test script whose test cases are to be counted.
Example Output:
3
This indicates there are three test cases in the specified test script.
Use Case 3: Run BATS Test Cases Recursively
Code:
bats --recursive path/to/directory
Motivation:
Running tests recursively through a directory makes it efficient to manage and execute a large suite of tests that are organized in multiple scripts. This feature supports scalability and organization in testing, allowing teams to build comprehensive testing suites logically distributed across directories.
Explanation:
bats
: Calls the BATS testing system.--recursive
: This option specifies that BATS should search through the indicated directory and execute any.bats
files it finds.path/to/directory
: The target directory containing BATS test scripts.
Example Output:
✓ test1.bats
✓ test2.bats
✓ nested/tests/test3.bats
...
Use Case 4: Output Results in a Specific Format
Code:
bats --formatter pretty|tap|tap13|junit path/to/test.bats
Motivation:
Different projects and environments may have varying needs for the consumption and analysis of test results. By allowing the output in multiple formats, BATS facilitates more widespread compatibility with other testing and CI/CD tools that may require results in a specific format, such as JUnit for Java-based systems.
Explanation:
bats
: Launches the BATS command.--formatter pretty|tap|tap13|junit
: Specifies the format of the output. “pretty” is human-readable, “tap” and “tap13” are protocols, while “junit” is particularly useful in integration with Java testing environments.path/to/test.bats
: Denotes the testing script to be executed and reported on.
Example Output for --formatter junit
:
<testsuite name="bats" tests="3">
<testcase classname="test" name="first test"/>
<testcase classname="test" name="second test">
<failure type=""/>
</testcase>
<testcase classname="test" name="third test"/>
</testsuite>
Use Case 5: Add Timing Information to Tests
Code:
bats --timing path/to/test.bats
Motivation:
Timing information is crucial in performance testing and optimization. By including timing data, BATS provides insights into which specific tests or functionalities are particularly time-consuming. This usage enables targeted performance optimizations.
Explanation:
bats
: Engages BATS for executing tests.--timing
: Instructs BATS to output the timing for each test case, helping analyze test performance.path/to/test.bats
: The file containing the test cases to be timed.
Example Output:
ok 1 first test (0.003s)
not ok 2 second test (0.005s)
ok 3 third test (0.002s)
Use Case 6: Run Specific Number of Jobs in Parallel
Code:
bats --jobs number path/to/test.bats
Motivation:
Running tests in parallel significantly reduces the total time of test execution, especially useful in a CI/CD environment where speedy feedback is crucial. This usage can dramatically accelerate development cycles and improve productivity.
Explanation:
bats
: Initiates the BATS test execution.--jobs number
: Specifies the number of parallel jobs to execute. An optimal number for this depends on the system’s CPU and resource availability.path/to/test.bats
: The batch of test scripts or a test file that is subject to parallel execution.
Example Output with --jobs 2
:
✓ first test
✓ second test
✓ third test
...
Conclusion:
Understanding and effectively using BATS commands allow for robust Bash script testing. With options that cater to different testing needs, from counting test cases to executing tests in various formats, BATS provides a comprehensive testing framework to match diverse environments and requirements, thereby contributing to reliable software development and release processes.