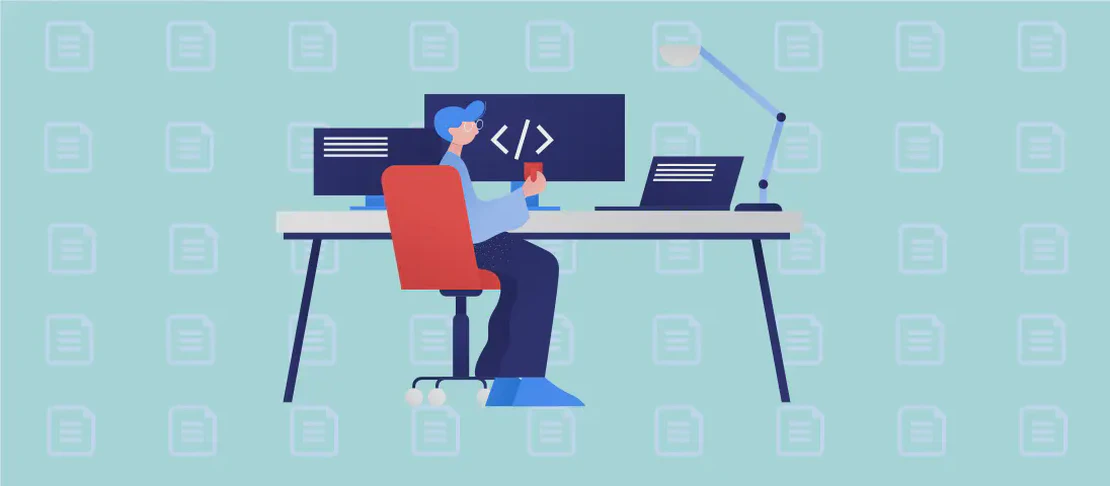
How to use the command 'bazel' (with examples)
Bazel is an open-source build and test tool that is particularly efficient for software development in large-scale environments, similar to other build automation tools like Make, Maven, and Gradle. It was originally developed to support the needs of Google, and it provides high performance and scalability for complex software projects. Bazel ensures fast builds by enabling caching and parallel execution. It supports projects in multiple languages, making it versatile for polyglot projects.
Use case 1: Build the specified target in the workspace
Code:
bazel build //path/to:target
Motivation:
The primary motivation for using the bazel build
command is to compile and assemble the components of your application specified by a particular target within the workspace. This is crucial for developers who want to ensure their code changes are correctly compiled and can be integrated with other components without error. Using this command can increase the efficiency and consistency of builds by relying on Bazel’s caching system, which avoids recompiling components that haven’t changed.
Explanation:
bazel
: The command-line interface of Bazel, responsible for executing the specified instruction.build
: This argument tells Bazel to carry out the build process for the following target. It triggers Bazel’s build system to compile the resources defined in the target.//path/to:target
: This specifies the path in the workspace to the target you want to build. Paths in Bazel are always prefixed with//
to denote the workspace root, while:target
specifies a particular build target within that path, such as a library or an executable.
Example output:
INFO: Analyzed target //path/to:target (0 packages loaded, 0 targets configured).
INFO: Found 1 target...
Target //path/to:target up-to-date:
bazel-bin/path/to/target
INFO: Build completed successfully, 1 total action
Use case 2: Remove output files and stop the server if running
Code:
bazel clean
Motivation:
The bazel clean
command is essential for maintaining a clean development environment. It is beneficial when developers encounter build issues and suspect that old build artifacts or cached files might be causing conflicts. Running this command ensures that all intermediate build outputs are removed, effectively allowing the developer to start fresh and resolve any issues that might have arisen from corrupted cache files.
Explanation:
bazel
: The command-line interface used to communicate with the Bazel build system.clean
: This command removes all output files inbazel-out
, effectively clearing the whole build cache. By doing so, it ensures that the subsequent build is performed without using the stale data that might have been cached from previous builds.
Example output:
INFO: Starting clean (this may take a while). Consider using --expunge if you want to delete all Bazel output.
Use case 3: Stop the bazel server
Code:
bazel shutdown
Motivation:
The bazel shutdown
command is useful for ensuring that any Bazel server processes running in the background are terminated. This may be necessary when updates or changes to Bazel’s configuration need to be applied and you want to ensure no lingering processes interfere with these changes. Additionally, shutting down the Bazel server can help in releasing system resources that it consumes while running.
Explanation:
bazel
: Indicates the utilization of the Bazel command-line interface.shutdown
: This command instructs Bazel to terminate its background server process, which manages various operations such as caching build data and configuring targets. Shutting down the server ends all such operations, freeing up resources.
Example output:
INFO: invoking shutdown
Use case 4: Display runtime info about the bazel server
Code:
bazel info
Motivation:
Using the bazel info
command is particularly valuable when you want to inspect the current state and configuration of the Bazel server. This is useful for troubleshooting by verifying details such as the execution root and output directory paths, and ensuring Bazel is using the correct installation and JVM settings. Developers use this command to get a quick overview without having to search multiple configuration files.
Explanation:
bazel
: The command interface of the Bazel tool.info
: Indicates that Bazel should return a summary of its current configuration and status. This includes paths to important directories, the output root, and the user-defined environment variables in effect during Bazel operations.
Example output:
bazel-bin: /home/user/.cache/bazel/_bazel_user/abcdef1234/execroot/my_workspace/bazel-out/k8-fastbuild/bin
bazel-genfiles: /home/user/.cache/bazel/_bazel_user/abcdef1234/execroot/my_workspace/bazel-out/k8-fastbuild/genfiles
bazel-testlogs: /home/user/.cache/bazel/_bazel_user/abcdef1234/execroot/my_workspace/bazel-out/k8-fastbuild/testlogs
...
Use case 5: Display help
Code:
bazel help
Motivation:
The bazel help
command is indispensable for both new and experienced developers who need quick assistance with Bazel’s extensive command set and options. It serves as an in-tool reference guide that helps users understand the syntax, available commands, options, rules, and flags within Bazel without leaving the terminal.
Explanation:
bazel
: The Bazel command-line interface.help
: This argument calls upon Bazel to display a general-help guide for the entire suite of Bazel commands. It provides descriptions for each command within Bazel and possibly additional options or arguments relevant to specific scenarios.
Example output:
Usage: bazel <command> <options> ...
Available commands:
analyze-profile Analyzes build profile data.
aquery Analyzes the dependency graph of the build.
build Builds the specified targets.
...
Use case 6: Display version
Code:
bazel version
Motivation:
Running the bazel version
command is crucial for development environments that necessitate consistency across tool versions, ensuring compatibility across different development machines or continuous integration pipelines. It informs the user of the current version of Bazel installed on their system, which is critical for debugging and ensuring compliance with project dependencies.
Explanation:
bazel
: Refers to the Bazel command interface.version
: This command directs Bazel to output its current version number along with other relevant version details like the JVM version in use and the host machine’s information.
Example output:
Build label: 5.0.0
Build time: Mon Feb 1 00:00:00 2021 (1612137600)
Build timestamp: 1612137600
Build timestamp as int: 1612137600
Conclusion:
Bazel provides a robust and efficient toolset for managing software builds and tests. The command-line interface shared by Bazel facilitates a range of essential developer tasks, from building targeted application components to managing the build environment for fault-free operation. Every command, from cleaning up outdated files to acquiring system details, is essential for ensuring a smooth and consistent development process. Each use case above exemplifies how Bazel efficiently handles common build system requirements while maintaining powerful features for advanced use cases.