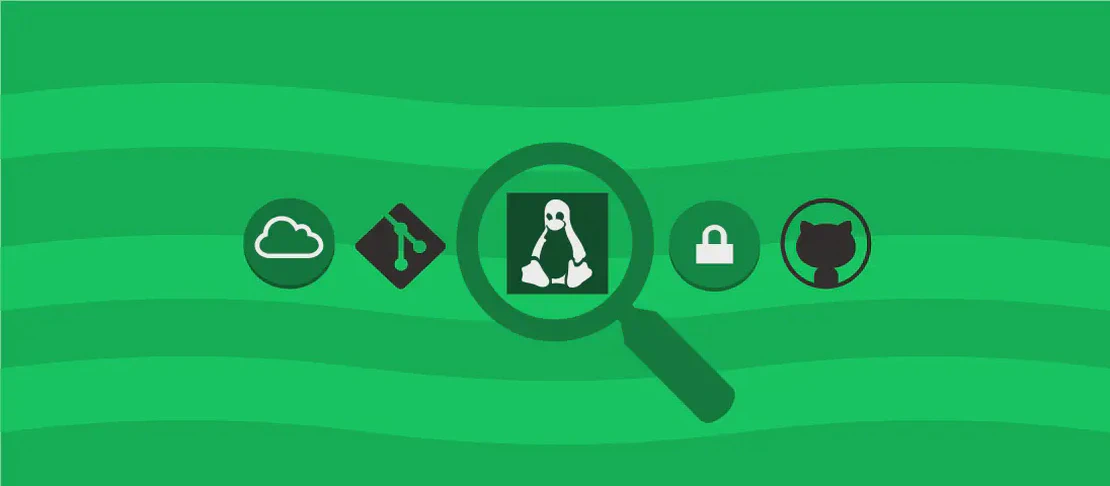
Mastering the 'bb' Command for Clojure Scripting (with examples)
The bb
command refers to Babashka, a native Clojure interpreter designed for scripting. It acts as a powerful tool that allows developers to rapidly execute Clojure code without needing the full JVM setup, making it an excellent resource for scripting tasks. It’s known for its speed and is perfect for small utility scripts that benefit from Clojure’s expressive syntax and functional programming capabilities. In the following sections, we illustrate various use cases of the bb
command with comprehensive examples.
Use case 1: Evaluate an Expression
Code:
bb -e "(+ 1 2 3)"
Motivation for this example: Sometimes you just need to perform a quick calculation or evaluate a small piece of code without writing a full script file. This use case demonstrates how Babashka can be utilized for such quick, one-off tasks, letting you leverage Clojure’s functional precision in rapid calculations.
Explanation of each argument:
-e
: This flag tells Babashka to evaluate the expression given in the command line. This mode is perfect for single, non-complex lines of Clojure code."(+ 1 2 3)"
: This is the Clojure expression being evaluated. It uses the built-in+
function to sum the numbers 1, 2, and 3.
Example output:
6
Use case 2: Evaluate a Script File
Code:
bb -f path/to/script.clj
Motivation for this example: Running entire scripts is fundamental when using Babashka for scripting tasks. Think of a scenario where you have a predefined set of operations you regularly perform and have compiled them into a Clojure script file. Babashka allows you to execute these scripts swiftly without needing to spin up a JVM.
Explanation of each argument:
-f
: This stands for ‘file’, indicating to Babashka that it should evaluate a complete script file located at the specified path.path/to/script.clj
: This is the path to the script file you wish to execute. You need to provide the correct path for Babashka to successfully find and run the script.
Example output:
(Example output would vary based on the content of script.clj
, so no specific output can be provided here.)
Use case 3: Bind Input to a Sequence of Lines from stdin
Code:
printf "first\nsecond" | bb -i "(map clojure.string/capitalize *input*)"
Motivation for this example:
Incorporating data directly from standard input is a common need in scripting. Suppose you are processing lines of text, for instance, log entries or names, and you want to transform or manipulate each line. This example demonstrates how you can use Babashka to read a list from stdin
, process it, and return the manipulated outcome.
Explanation of each argument:
printf "first\nsecond"
: This simulates input from a newline-separated string.|
: The pipe operator, used here to feed the output ofprintf
as the input to the Babashka command.-i
: This flag binds the input fromstdin
to*input*
, which Babashka processes as a sequence of strings, one per line."(map clojure.string/capitalize *input*)"
: The Clojure code maps thecapitalize
function across each line in the input, transforming it.
Example output:
("First" "Second")
Use case 4: Bind Input to a Sequence of EDN Values from stdin
Code:
echo "{:key 'val}" | bb -I "(:key (first *input*))"
Motivation for this example:
EDN (Extensible Data Notation) is a flexible format that Clojure programs often use for data exchange. This example illustrates how to use Babashka to read and manipulate EDN data streamed through stdin
, which can be crucial when dealing with configuration files or transferring complex data structures in a Clojure-friendly manner.
Explanation of each argument:
echo "{:key 'val}"
: This simulates passing EDN data viastdin
.|
: The pipe operator to passecho
output as input to Babashka.-I
: This flag treats the input as EDN, meaning Babashka will parse the input data accordingly."(:key (first *input*))"
: This Clojure expression extracts the value corresponding to the:key
in the first EDN element from input.
Example output:
val
Conclusion:
These use cases exemplify the versatile capabilities of Babashka. Whether for quick evaluations, full script execution, straightforward text processing, or handling EDN, the bb
command is a bridge between powerful Clojure programming and practical scripting requirements—making it an invaluable tool for developers looking to leverage Clojure’s expressiveness across various automation tasks.