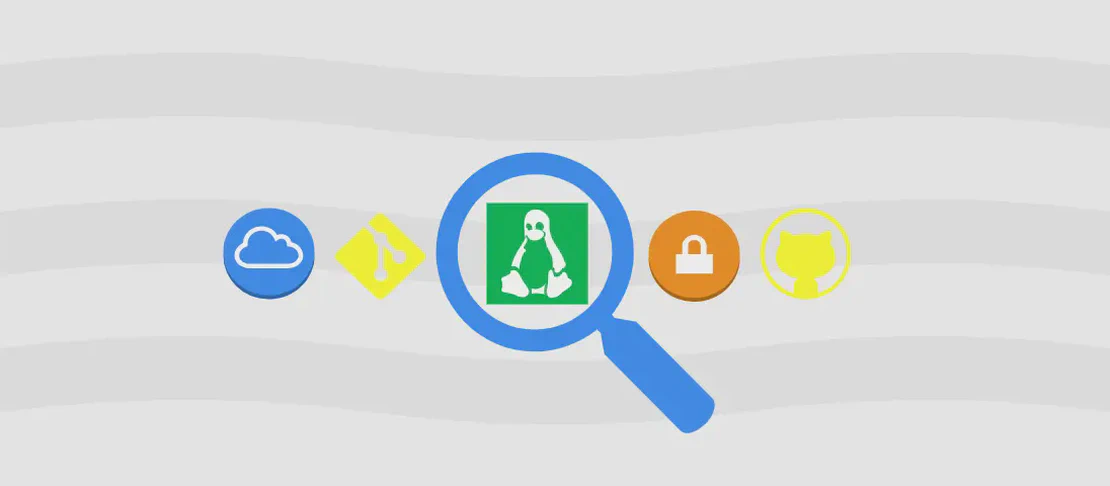
How to use the command 'bc' (with examples)
The bc
command stands for “basic calculator,” but it is much more than that. It is an arbitrary precision calculator language, meaning it can handle very large and very small numbers with precision beyond standard floating-point representation. Frequently used in scripting and quick terminal calculations, bc
is a versatile, essential tool for its ability to operate in both interactive and command-line modes. It supports a wide variety of mathematical operations and can also execute custom scripts written in its own language.
Use case 1: Start an interactive session
Code:
bc
Motivation:
Starting an interactive session with bc
is useful when you need to perform a sequence of calculations on-the-fly. This mode is particularly beneficial for performing quick computations without needing to create scripts or use a dedicated desktop calculator application.
Explanation:
The command bc
without any additional arguments launches the calculator in an interactive mode right inside the terminal. Here, you can enter calculations line-by-line and immediately see the results. The interactivity allows for experimentation, modifications, and learning by doing, all in real-time.
Example output:
> 25 + 75
100
> 7 * 8
56
> quit
Use case 2: Start an interactive session with the standard math library enabled
Code:
bc --interactive --mathlib
Motivation:
Sometimes, basic arithmetic is not enough, especially when working with trigonometric functions, logarithms, and exponential calculations. By enabling the math library in an interactive session, you can compute more complex mathematical expressions. This is essential for users performing advanced scientific or engineering calculations.
Explanation:
--interactive
: Althoughbc
defaults to interactive mode when no files or expressions are provided, this flag explicitly tellsbc
to open in interactive mode, ensuring clarity of purpose.--mathlib
: This argument activates predefined functions withinbc
, such as sine (s), cosine (c), arctangent (a), logarithmic (l), and exponential (e) functions, thus expanding its computational capability significantly.
Example output:
> s(1)
.8414709848
> c(1)
.5403023058
> quit
Use case 3: Calculate an expression
Code:
echo '5 / 3' | bc
Motivation:
This use case demonstrates how to calculate a simple mathematical expression from the command line directly without entering the interactive mode. It’s advantageous when you want to embed mathematical operations in a shell script or quickly calculate an expression from the terminal with a single command.
Explanation:
echo '5 / 3'
: This part of the command generates the string5 / 3
, which is an expression for division.|
: The pipe operator sends the output ofecho
as input to the next command.bc
: This takes the input fromecho
and computes the division of 5 by 3.
Example output:
1
Use case 4: Execute a script
Code:
bc path/to/script.bc
Motivation:
Executing a script with bc
is ideal for automating complex calculations or performing repetitive tasks. This method allows users to write scripts with a series of operations that bc
can execute sequentially, making it highly valuable for batch processing and tasks requiring consistent processing logic.
Explanation:
path/to/script.bc
: This is the path to a file containingbc
language script. The script file should contain validbc
syntax and operations that you want to execute.
Example output:
Assuming path/to/script.bc
contains:
x = 25 * 8
print x
The output would be:
200
Use case 5: Calculate an expression with the specified scale
Code:
echo 'scale = 10; 5 / 3' | bc
Motivation:
In scenarios where precision is critical, like financial calculations or scientific computations, defining the scale—the number of digits after the decimal point—is crucial. This example showcases how to manage result precision using bc
.
Explanation:
scale = 10;
: Sets the scale or decimal precision to 10 places.5 / 3
: Computes the division of 5 by 3 with the previously defined precision.
Example output:
1.6666666666
Use case 6: Calculate a sine/cosine/arctangent/natural logarithm/exponential function using mathlib
Code:
echo 's|c|a|l|e(1)' | bc --mathlib
Motivation:
Mathematics involving trigonometric, logarithmic, or exponential functions is commonplace in many fields, from engineering to data science. The use of bc
with the math library allows complex mathematical functions to be calculated easily from within scripts or command-line input.
Explanation:
's|c|a|l|e(1)'
: A placeholder calculation for sine (s), cosine (c), arctangent (a), and logarithmic/exponential (l|e) with the input value 1. Each character represents a mathematical function: sine (’s’), cosine (‘c’), arctangent (‘a’), and exponential/logarithm (’l’/’e’).--mathlib
: Enables access to advanced mathematical functions inbc
.
Example output:
.8414709848
.5403023058
.7853981633
.0
2.718281828
Use case 7: Execute an inline factorial script
Code:
echo "define factorial(n) { if (n <= 1) return 1; return n*factorial(n-1); }; factorial(10)" | bc
Motivation:
Factorials are a foundational concept in mathematics, particularly in combinatorics and statistical calculations. This inline scripting capability allows users to calculate factorials and other recursive functions without needing a separate script file, which is incredibly handy for short, declarative tasks like mathematics homework or quick factorial lookups.
Explanation:
define factorial(n) {...}
: This is a function definition withinbc
. It recursively calculates the factorial ofn
, wheren
is a non-negative integer.factorial(10)
: Calls thefactorial
function with an argument of 10, triggering the calculation.
Example output:
3628800
Conclusion:
The bc
command is an incredibly powerful tool that extends far beyond simple arithmetic. Its ability to handle large numbers, high precision calculations, and perform advanced mathematical operations makes it a staple tool for anyone needing quick, reliable calculations. From interactive sessions to scripted solutions, bc
provides a versatile environment for mathematical computations. Knowing how to leverage each use case allows users to maximize its utility in both everyday tasks and more complex computational scenarios.